How to Convert an Array Object to a String in PowerShell
- Convert an Array Object to a String in PowerShell Using Double Inverted Commas
-
Convert an Array Object to a String in PowerShell Using the
-join
Operator - Convert an Array Object to a String in PowerShell Using Explicit Conversion
- Convert an Array Object to a String in PowerShell Using the Output Field Separator Variable
-
Convert an Array Object to a String in PowerShell Using the
[system.String]::Join()
Function -
Convert an Array Object to a String in PowerShell Using the
Out-String
Cmdlet - Conclusion
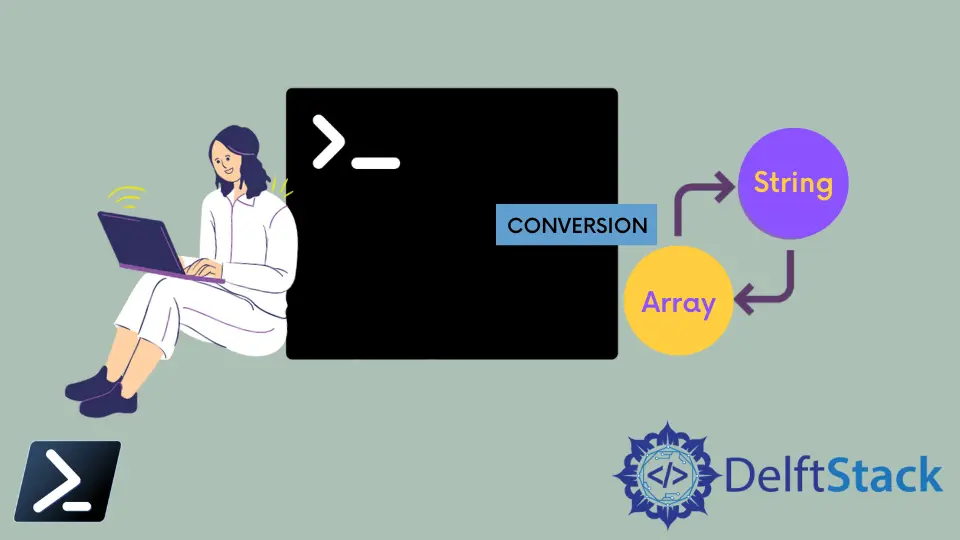
In PowerShell scripting, often manipulating data involves transforming array objects into strings, a common task encountered in various scenarios. Whether you’re concatenating elements for display, formatting output, or preparing data for further processing, PowerShell provides a diverse set of methods to streamline the conversion process.
This article explores several approaches, ranging from the straightforward use of double inverted commas to the -join
operator, explicit conversion, the Output Field Separator variable, [system.String]::Join()
, and the Out-String
cmdlet.
Convert an Array Object to a String in PowerShell Using Double Inverted Commas
A straightforward approach to convert an array object into a string is by using double inverted commas (" "
).
Double inverted commas are used to denote strings in PowerShell. When applied to an array variable, this method implicitly converts the array into a string by concatenating its elements.
The resulting string will have the array elements joined together without any separators.
Let’s consider a practical example. Suppose we have an array object called $address
:
$address = "Where", "are", "you", "from?"
To convert this array into a string using double inverted commas, we simply enclose the array variable in the quotes:
$address = "Where", "are", "you", "from?"
"$address"
This single line of code is sufficient to transform the array into a string. When PowerShell encounters this syntax, it interprets it as a request to concatenate the elements of the array into a single string.
Output:
Where are you from?
Let’s apply $address.GetType()
to verify that the original variable is indeed an array:
$address = "Where", "are", "you", "from?"
$address.GetType()
Output:
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True Object[] System.Array
A subsequent check of the data type using "$address".GetType()
confirms that the variable has been transformed into a string:
$address = "Where", "are", "you", "from?"
"$address".GetType()
Output:
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True String System.Object
The use of double inverted commas simplifies the process of converting an array object into a string in PowerShell. It provides a concise and intuitive way to achieve this transformation.
Convert an Array Object to a String in PowerShell Using the -join
Operator
The -join
operator in PowerShell is designed to join an array of items into a single string. By specifying a separator, we control how the elements are concatenated.
In the context of converting an array to a string, the -join
operator provides flexibility in shaping the output according to our requirements.
Let’s illustrate the process using a practical example. Assume we have the same array named $address
.
To convert this array into a string using the -join
operator, we specify a separator within the operator:
$address = "Where", "are", "you", "from?"
$joinedString = $address -join " "
Here, we use a space (" "
) as the separator to join the elements of the array with a space in between.
The use of the -join
operator eliminates the need for explicit iteration or casting, providing a concise and readable solution for converting an array to a string.
To inspect the result, we can simply output the contents of the $joinedString
variable:
$address = "Where", "are", "you", "from?"
$joinedString = $address -join " "
$joinedString
Output:
Where are you from?
The -join
operator streamlines the process of converting array objects into strings in PowerShell by allowing us to control the concatenation with a specified separator.
Convert an Array Object to a String in PowerShell Using Explicit Conversion
Explicit conversion offers another straightforward method to transform an array object into a string by explicitly casting it to the desired data type. In the case of converting an array to a string, we explicitly cast the array variable to the string data type.
This informs PowerShell to treat the array as a string, resulting in the concatenation of its elements into a single string.
Consider the following example where we have an array named $address
. To explicitly convert this array into a string, we use the casting operator [string]
:
$address = "Where", "are", "you", "from?"
$convertedString = [string]$address
The explicit conversion is denoted by [string]
, indicating that the variable $address
is to be treated as a string and concatenate its elements.
To observe the result, we can simply output the contents of the $convertedString
variable:
$address = "Where", "are", "you", "from?"
$convertedString = [string]$address
$convertedString
Output:
Where are you from?
Explicit conversion is particularly useful when a clear and direct transformation is desired without relying on operators or methods. It provides a concise and readable way to convert an array to a string in a single line of code.
Convert an Array Object to a String in PowerShell Using the Output Field Separator Variable
The Output Field Separator variable, $OFS
, provides a handy way to control how array elements are concatenated when converted to a string.
By setting the $OFS
variable to a specific value, we influence how the array elements are joined. This allows for customization of the string output according to our preferences.
To convert the array $address
into a string using the $OFS
variable, we set $OFS
to a desired separator and then enclose the array variable in double inverted commas:
$address = "Where", "are", "you", "from?"
$OFS = '-'
$outputString = "$address"
Here, we use a hyphen (-
) as the separator. When PowerShell encounters this syntax with a set $OFS
, it concatenates the array elements using the specified separator.
To examine the result, we can simply output the contents of the $outputString
variable:
$address = "Where", "are", "you", "from?"
$OFS = '-'
$outputString = "$address"
$outputString
Output:
Where-are-you-from?
The $OFS
variable is a powerful tool for customizing the output format, allowing for dynamic adjustments to how array elements are presented in the resulting string.
Convert an Array Object to a String in PowerShell Using the [system.String]::Join()
Function
In PowerShell, the [system.String]::Join()
function offers another robust method for converting an array object into a string.
The [system.String]::Join()
function is a static method of the System.String
class that facilitates the concatenation of array elements into a single string. By providing a separator and the array as arguments, this method simplifies the process of converting an array to a string.
Let’s consider the same array named $address
with four string elements. To convert the $address
array into a string using [system.String]::Join()
, we call the method with the desired separator and the array:
$address = "Where", "are", "you", "from?"
$joinedString = [system.String]::Join(" ", $address)
Here, we use a space (" "
) as the separator. This method effectively replaces the need for explicit iteration or casting, streamlining the conversion process.
To observe the result, we can simply output the contents of the $joinedString
variable:
$address = "Where", "are", "you", "from?"
$joinedString = [system.String]::Join(" ", $address)
$joinedString
Output:
Where are you from?
Using [system.String]::Join()
is especially advantageous when dealing with arrays, offering a clean and concise way to control the concatenation process.
Convert an Array Object to a String in PowerShell Using the Out-String
Cmdlet
The Out-String
cmdlet is another versatile tool that can be employed to convert an array object into a string. It concatenates the elements of the array, producing a single string.
It’s particularly useful when dealing with objects that may not have a direct string representation.
Let’s consider a scenario where we have an array named $address
with four string elements. To convert this array into a string using the Out-String
cmdlet, we simply pass the array as input to the cmdlet:
$address = "Where", "are", "you", "from?"
$stringOutput = $address | Out-String
Here, we use the Out-String
cmdlet by piping the array to it ($address | Out-String
). This operation implicitly concatenates the elements of the array, producing a single string.
To see the result, we can simply output the contents of the $stringOutput
variable:
$address = "Where", "are", "you", "from?"
$stringOutput = $address | Out-String
$stringOutput
Output:
Where
are
you
from?
The Out-String
cmdlet is particularly useful when working with complex objects or arrays that may not have a straightforward string representation. It ensures that the output is formatted as a string for easy consumption or further processing.
Conclusion
PowerShell offers a variety of methods to convert an array object into a string, each with its unique advantages. Whether utilizing the double inverted commas, the -join
operator, explicit conversion, the Output Field Separator variable, [system.String]::Join()
, or the Out-String
cmdlet, PowerShell provides developers with a rich toolkit to handle this common task.
The choice of method depends on your specific requirements, coding preferences, and the nature of the data being manipulated. By understanding these techniques, you can seamlessly transform array objects into strings, enhancing code readability and effectiveness in various scripting scenarios.
Related Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell