How to Create an Empty Array of Arrays in PowerShell
- Understanding Arrays in PowerShell
-
How to Create an Empty Array of Arrays in PowerShell Using the
@()
Array Subexpression Operator -
How to Create an Empty Array of Arrays in PowerShell Using the
New-Object
Cmdlet With theSystem.Collections.ArrayList
Class -
How to Create an Empty Array of Arrays in PowerShell Using the
@{}
Hashtable - Conclusion
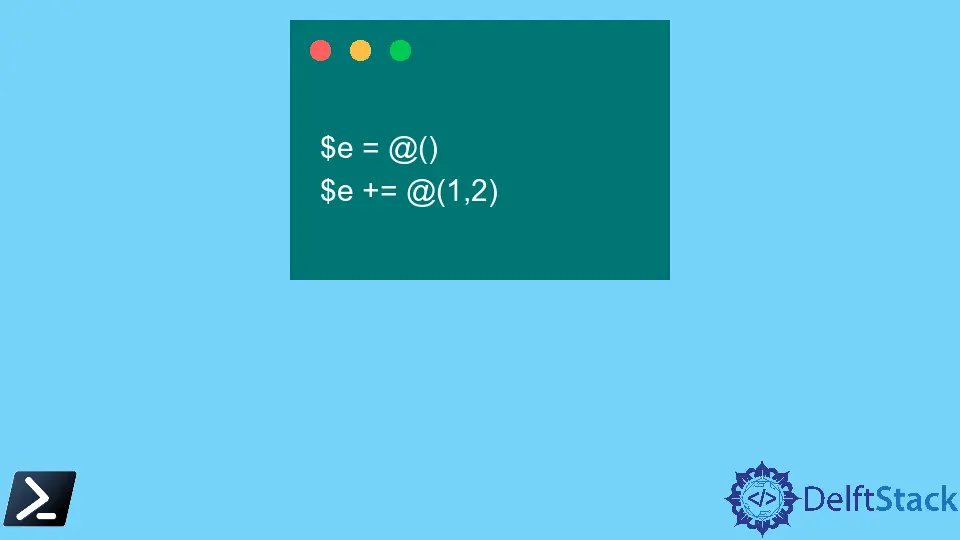
PowerShell, with its versatile scripting capabilities, offers several ways to create and manipulate arrays.
One common requirement is to create an empty array that can hold other arrays. This scenario often arises when you need to organize data into a multi-dimensional structure.
In this article, we’ll explore various methods to create an empty array of arrays in PowerShell.
Understanding Arrays in PowerShell
Before delving into creating an empty array of arrays, it’s crucial to understand the basics of arrays in PowerShell. An array in PowerShell is a data structure that stores a collection of elements.
These elements can be of any data type, and arrays can dynamically resize to accommodate new elements as needed. PowerShell provides several ways to work with arrays, including creating, accessing, and modifying their contents.
Here’s a basic example of creating an array in PowerShell:
$myArray = @(1, 2, 3, 4, 5)
$myArray
In this example, $myArray
is assigned an array containing five integers.
Output:
In PowerShell, arrays can contain elements of any type, including other arrays. An array of arrays, also known as a jagged array, is a data structure that consists of multiple arrays, where each element of the main array holds another array.
This structure enables you to store heterogeneous data in a multi-dimensional format, making it easier to manage and access elements based on different criteria.
Arrays in PowerShell are zero-indexed, meaning the first element is accessed using index 0, the second element using index 1, and so on. PowerShell arrays can dynamically resize, allowing for flexibility in managing data.
How to Create an Empty Array of Arrays in PowerShell Using the @()
Array Subexpression Operator
The @()
array subexpression operator is a versatile tool in PowerShell that can be used for various array operations, including creating empty arrays. When used to create an empty array of arrays, it initializes an array container capable of holding other arrays as its elements.
By using this operator along with the comma ,
to separate elements, we can build an array structure where each element is itself an array.
Let’s see a code example to illustrate how to create an empty array of arrays using the @()
array subexpression operator.
$arrayOfArrays = @()
Here, we initialize an empty array named $arrayOfArrays
using the @()
array subexpression operator. This creates an empty array container capable of holding other arrays.
Since no elements are provided within the @()
operator, the resulting array is empty.
Adding Arrays to the Empty Array
Once you have created the empty array of arrays, you can add individual arrays to it as elements. This is commonly done using the +=
operator, which appends an element to an existing array:
$arrayOfArrays += , (1, 2, 3)
$arrayOfArrays += , (4, 5)
$arrayOfArrays += , (6, 7, 8, 9)
Write-Host "First Array:"
$arrayOfArrays[0]
Write-Host "Second Array:"
$arrayOfArrays[1]
Write-Host "Third Array:"
$arrayOfArrays[2]
In this code segment, we add subarrays to the $arrayOfArrays
. To ensure each subarray is treated as a single element, we precede it with a comma ,
.
This prevents PowerShell from concatenating the subarrays into a single array. Each subarray is enclosed within parentheses and separated by commas.
We add three subarrays with varying lengths to demonstrate flexibility.
Finally, we display the contents of the $arrayOfArrays
to verify its structure and contents. PowerShell outputs the array, showing each element as a separate subarray.
Code Output:
The output demonstrates that $arrayOfArrays
is indeed an array containing multiple subarrays, each holding a distinct set of values. This confirms the successful creation of an empty array of arrays using the @()
array subexpression operator in PowerShell.
How to Create an Empty Array of Arrays in PowerShell Using the New-Object
Cmdlet With the System.Collections.ArrayList
Class
Another approach we can use to create an empty array of arrays to store structured data is the New-Object
cmdlet in conjunction with the System.Collections.ArrayList
class.
The System.Collections.ArrayList
class in PowerShell provides a flexible and dynamic array-like data structure. By utilizing the New-Object
cmdlet, we can instantiate an instance of this class to create an empty array.
Subsequently, we can add subarrays as elements to this array of arrays. This approach allows for dynamic resizing and efficient management of arrays, making it suitable for various scripting scenarios.
Let’s see how we can create an empty array of arrays using this approach:
$arrayOfArrays = New-Object System.Collections.ArrayList
Here, we use the New-Object
cmdlet to instantiate a new instance of the System.Collections.ArrayList
class. This creates an empty array container capable of holding other arrays.
The variable $arrayOfArrays
now references this empty ArrayList
object.
Accessing Elements of the Array of Arrays
After creating the empty array, we can now access and manipulate its elements as needed:
$arrayOfArrays.Add(@(11, 12, 13))
$arrayOfArrays.Add(@(14, 15))
$arrayOfArrays.Add(@(16, 17, 18, 19))
Write-Host "First Array:"
$arrayOfArrays[0]
Write-Host "Second Array:"
$arrayOfArrays[1]
Write-Host "Third Array:"
$arrayOfArrays[2]
In this code segment, we add subarrays to the $arrayOfArrays ArrayList
object. We use the .Add()
method of the ArrayList
object to append subarrays to the array.
Each subarray is enclosed within @()
to ensure it is treated as a single element. We add three subarrays with varying lengths to showcase the flexibility of this approach.
Finally, we display the contents of the $arrayOfArrays ArrayList
object to verify its structure and contents. PowerShell outputs the ArrayList
, showing each element as a separate subarray.
Code Output:
The output confirms that $arrayOfArrays
is an ArrayList
containing multiple subarrays, each holding distinct sets of values. This demonstrates the successful creation of an empty array of arrays using the New-Object
cmdlet with the System.Collections.ArrayList
class in PowerShell.
How to Create an Empty Array of Arrays in PowerShell Using the @{}
Hashtable
In PowerShell, hashtables (@{}
) provide a convenient way to store key-value pairs. While typically used for key-value pairs, we can also utilize hashtables to create an empty array of arrays by associating each key with an empty array.
This method provides a clear organizational structure for managing multiple arrays and allows for easy access and manipulation of array elements.
Here’s how to create an empty array of arrays using the @{}
hashtable syntax:
$arrayOfArrays = @{}
Adding Elements to the Array of Arrays
Now that we have created the empty array of arrays using the hashtable approach, we can access and manipulate its elements as needed:
$arrayOfArrays["Array1"] += @(1, 2, 3)
$arrayOfArrays["Array2"] += @(4, 5)
$arrayOfArrays["Array3"] += @(6, 7, 8, 9)
$arrayOfArrays
In this code segment, we add subarrays to the empty arrays within $arrayOfArrays
. We use array indexing to access each empty array by its key ("Array1"
, "Array2"
, "Array3"
) and then append subarrays using the +=
operator.
Each subarray is enclosed within @()
to ensure it is treated as a single element.
Finally, we display the contents of the $arrayOfArrays
hashtable to verify its structure and contents. PowerShell outputs the hashtable, showing each key-value pair, where the values are arrays containing subarrays.
Code Output:
The output confirms that $arrayOfArrays
is a hashtable containing three keys ("Array1"
, "Array2"
, "Array3"
), each associated with an array containing distinct sets of values.
Conclusion
In conclusion, creating an empty array of arrays in PowerShell offers versatility and flexibility for managing complex data structures in scripting and automation tasks.
We explored three distinct methods for achieving this: using the @()
array subexpression operator, the New-Object
cmdlet with the System.Collections.ArrayList
class, and repurposing the @{}
hashtable syntax. Each method presents its advantages and considerations, catering to different preferences and requirements.
The @()
array subexpression operator provides a concise and straightforward approach, leveraging native PowerShell syntax to create empty arrays efficiently. Meanwhile, the New-Object
cmdlet with the System.Collections.ArrayList
class offers dynamic resizing and management capabilities, suitable for scenarios requiring frequent array manipulation.
Finally, repurposing the @{}
hashtable syntax, although unconventional, provides simplicity and readability in certain contexts.
Regardless of the chosen method, mastering the creation of empty arrays of arrays equips PowerShell users with essential skills for developing robust scripts and automation.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn