Byte Array in PowerShell
- Convert Data to a Byte Array in PowerShell
- Convert Data to a Byte Array in PowerShell 5+
- Convert Data to a Byte Array in PowerShell 7+
- Convert Byte Array to String in PowerShell
- Convert String to Byte Array in PowerShell
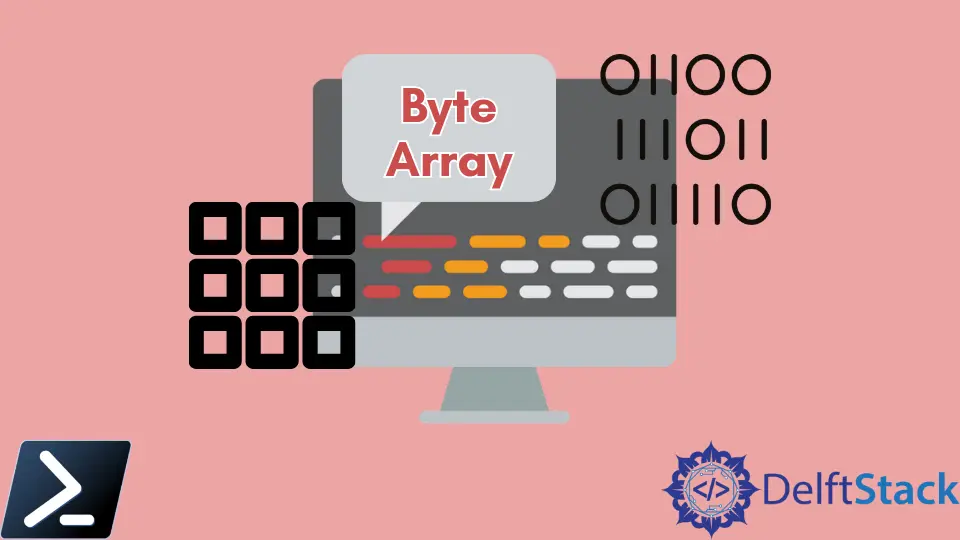
This article aims to demonstrate how to convert data present in various forms to a Byte array using PowerShell Scripting API.
Convert Data to a Byte Array in PowerShell
While automating tasks and handling data, the data may need to be processed in a specific manner to extract useful information or modify data in a meaningful manner. Handling such data in PowerShell can sometimes become inconvenient to process, especially in another data type.
To make the processing easier or reduce the number of processing calculations, it can be ideal in some cases to convert the data into a Byte array and then perform operations on that instead of the original data.
Consider the following code:
$data = Get-Content "a.exe"
Get-Item "a.exe" | Format-List -Property * -Force
Write-Host "File Datatype: "$data.GetType().Name
This gives the following output:
< Output redacted >
.
.
.
.
BaseName : a
Target :
LinkType :
Length : 400644
.
.
.
Extension : .exe
Name : a.exe
Exists : True
.
.
.
.
.
Attributes : Archive
File Datatype: Object[]
From the above output, the file we have opened is a Windows executable (exe
) file. After looking at its attributes using Get-Item
and Format-List
cmdlets, meaningful insight can be gained regarding the file, especially the size, which can prove quite useful during iterations.
One unfavorable aspect of this situation is that the retrieved file data by using the Get-Content
cmdlet is that Object[]
is returned.
Let’s see how we can convert this into Byte[]
for scenario-dependent operations.
Convert Data to a Byte Array in PowerShell 5+
Consider the following code:
$file = "a.exe"
[byte[]]$data = Get-Content $file -Encoding Byte
Write-Host "File Datatype: "$data.GetType().Name
for ($i = 0; $i -lt 10; $i++) { Write-Host $data[$i] }
This gives the following output:
File Datatype: Byte[]
Byte No 0 : 77
Byte No 1 : 90
Byte No 2 : 144
Byte No 3 : 0
Byte No 4 : 3
Byte No 5 : 0
Byte No 6 : 0
Byte No 7 : 0
Byte No 8 : 4
Byte No 9 : 0
Byte No 10 : 0
With type casting and the -Encoding
parameter, it is possible to read the file as a Byte array directly. We can verify that the result is indeed a Byte array by using the GetType()
method and accessing its property named Name
.
To further verify that the data has indeed converted correctly, we can write up a small for
loop and print some of the bytes of the file.
Convert Data to a Byte Array in PowerShell 7+
Consider the following code:
[byte[]]$data = Get-Content "a.exe" -AsByteStream
Write-Host $data.GetType().Name
This gives the following output:
textByte[]
Most of the syntax is the same between versions. The only difference is that -Encoding Byte
has been replaced by -AsByteStream
.
Convert Byte Array to String in PowerShell
Converting any given byte array to a string is easy. Consider the following code:
$array = @(0x54, 0x68, 0x65, 0x20, 0x6f, 0x62, 0x6a, 0x65, 0x63, 0x74, 0x20, 0x6f, 0x66, 0x20, 0x6c, 0x69, 0x66, 0x65, 0x20, 0x69, 0x73, 0x20, 0x6e, 0x6f, 0x74, 0x20, 0x74, 0x6f, 0x20, 0x62, 0x65, 0x20, 0x6f, 0x6e, 0x20, 0x74, 0x68, 0x65, 0x20, 0x73, 0x69, 0x64, 0x65, 0x20, 0x6f, 0x66, 0x20, 0x74, 0x68, 0x65, 0x20, 0x6d, 0x61, 0x6a, 0x6f, 0x72, 0x69, 0x74, 0x79, 0x2c, 0x20, 0x62, 0x75, 0x74, 0x20, 0x74, 0x6f, 0x20, 0x65, 0x73, 0x63, 0x61, 0x70, 0x65, 0x20, 0x66, 0x69, 0x6e, 0x64, 0x69, 0x6e, 0x67, 0x20, 0x6f, 0x6e, 0x65, 0x73, 0x65, 0x6c, 0x66, 0x20, 0x69, 0x6e, 0x20, 0x74, 0x68, 0x65, 0x20, 0x72, 0x61, 0x6e, 0x6b, 0x73, 0x20, 0x6f, 0x66, 0x20, 0x74, 0x68, 0x65, 0x20, 0x69, 0x6e, 0x73, 0x61, 0x6e, 0x65, 0x2e)
$string = [System.Text.Encoding]::UTF8.GetString($array)
$string
Which gives the following output:
The object of life is not to be on the side of the majority but to escape finding oneself in the ranks of the insane.
Using the UTF8.GetString
method, we can convert any UTF8-encoded byte array back into its string representation. Be sure to look out for the encoding of the text, as using this method on other encodings (such as ASCII) may yield abnormal results.
Convert String to Byte Array in PowerShell
Similarly to how a byte array can be converted into its string representation, a string can also be converted into its byte representation.
Consider the following code:
$string = "Never esteem anything as of advantage to you that will make you break your word or lose your self-respect."
$bytes = [System.Text.Encoding]::Unicode.GetBytes($string)
Write-Host "First 10 Bytes of String are: "
for ($i = 0; $i -lt 10; $i++) { Write-Host $bytes[$i] }
This gives the output:
First 10 Bytes of String are:
78
0
101
0
118
0
101
0
114
0
Unicode.GetBytes
can be used in this specific scenario to get bytes of a Unicode string. Ensure that the string is Unicode; otherwise, the conversion can result in losing important data.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn