How to Sorting Array Values Using PowerShell
-
Sort Array Values in PowerShell Using the
Sort-Object
Cmdlet -
Sort Array Values in PowerShell Using the
[Array]::Sort()
Method - Conclusion
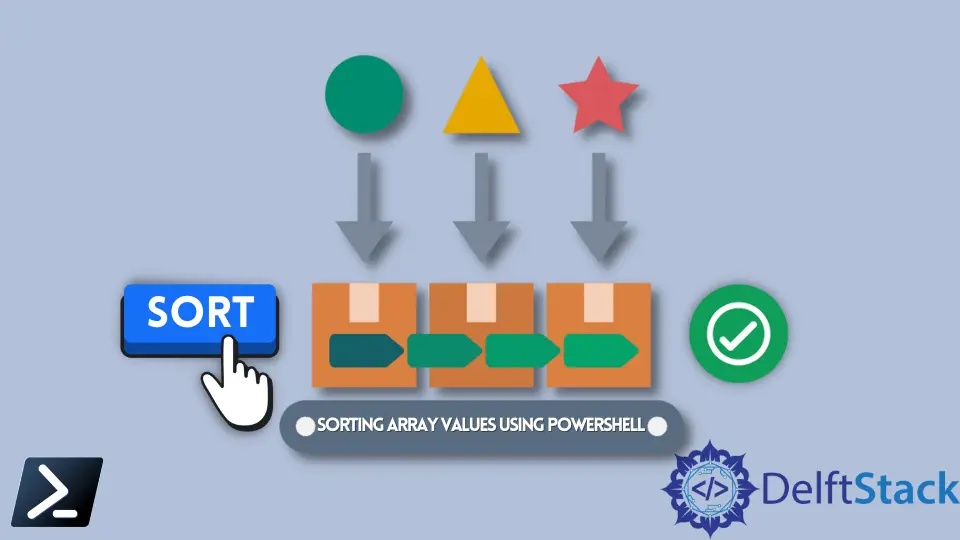
Sorting arrays is a common and essential task in PowerShell scripting, enabling efficient data organization and manipulation. Whether dealing with numeric, string, or custom object arrays, PowerShell provides versatile tools for sorting.
In this comprehensive article, we will explore different methods and techniques to sort array values, catering to various scenarios and preferences.
Sort Array Values in PowerShell Using the Sort-Object
Cmdlet
The Sort-Object
cmdlet provides a convenient and versatile way to arrange array values in ascending or descending order based on various criteria. It is not limited to arrays and can be used with any collection of objects.
When it comes to sorting arrays, each element in the array is considered an object, and the Sort-Object
cmdlet is applied accordingly.
The basic syntax of the Sort-Object
cmdlet is as follows:
<array> | Sort-Object [-Property <property>] [-Descending] [-Culture <culture>] [-Unique]
Where:
<array>
: Represents the array you want to sort.-Property
: Specifies the property based on which sorting should be performed. If not provided, PowerShell uses the default sort properties of the first input object.-Descending
: Sorts the objects in descending order. If this parameter is not used, the sorting is done in ascending order.-Culture
: Specifies the culture to use for sorting. This is optional and defaults to the current culture.-Unique
: Ensures that the sorted list contains unique items.
Let’s dive into practical examples to understand how to use the Sort-Object
cmdlet for sorting array values.
Example 1: Sorting in Ascending Order
$numbers = 4, 2, 7, 1, 5
$sortedNumbers = $numbers | Sort-Object
$sortedNumbers
Here, we have an array of numbers stored in the variable $numbers
. By piping this array to Sort-Object
, we sort the numbers in ascending order.
The sorted array is stored in $sortedNumbers
.
Output:
1
2
4
5
7
Example 2: Sorting in Descending Order
$cities = "New York", "London", "Paris", "Tokyo", "Sydney"
$sortedCitiesDescending = $cities | Sort-Object -Descending
$sortedCitiesDescending
In this example, the array $cities
contains city names. We use the -Descending
parameter to sort them in descending order, and the result is stored in $sortedCitiesDescending
.
Output:
Tokyo
Sydney
Paris
New York
London
Example 3: Sorting Objects Based on Property
$people = @( [PSCustomObject]@{ Name = "Alice"; Age = 25 },
[PSCustomObject]@{ Name = "Bob"; Age = 30 },
[PSCustomObject]@{ Name = "Charlie"; Age = 22 } )
$sortedPeople = $people | Sort-Object Age
$sortedPeople
In this example, we have an array of custom objects representing people with Name
and Age
properties. We use Sort-Object
with the -Property
parameter to sort the array based on the Age
property.
Output:
Name Age
---- ---
Charlie 22
Alice 25
Bob 30
These examples showcase the flexibility and power of the Sort-Object
cmdlet in sorting array values in PowerShell. Whether you are working with simple arrays or arrays of complex objects, Sort-Object
provides a straightforward and effective solution.
Sort Array Values in PowerShell Using the [Array]::Sort()
Method
While the Sort-Object
cmdlet is a powerful tool for sorting arrays, PowerShell also offers the [Array]::Sort()
method, a member of the [Array]
class in .NET
. This method is specifically designed for sorting arrays. It directly modifies the order of elements in the original array, making it an in-place sorting algorithm.
The syntax for using the [Array]::Sort()
method is as follows:
[Array]::Sort(<array>)
<array>
: Represents the array you want to sort.
Let’s see some examples to understand how to utilize the [Array]::Sort()
method for sorting array values.
Example 1: Sorting in Ascending Order
To sort an array in ascending order using the [Array]::Sort()
method, we can invoke the method and pass the array as an argument:
$numbers = 4, 2, 7, 1, 5
[Array]::Sort($numbers)
$numbers
In this example, the array $numbers
contains numeric values. We use the [Array]::Sort()
method to sort the array in ascending order directly. The sorting is performed in place, meaning the original array is modified.
Output:
1
2
4
5
7
Example 2: Sorting Strings in Ascending Order
$fruits = "Apple", "Orange", "Banana", "Grapes"
[Array]::Sort($fruits)
$fruits
Here, the array $fruits
contains string values representing fruits. The [Array]::Sort()
method is applied to sort the array alphabetically in ascending order. The original array is modified in place.
Output:
Apple
Banana
Grapes
Orange
Example 3: Sorting in Descending Order
$ages = 35, 22, 28, 40, 30
[Array]::Sort($ages)
$ages = $ages -join ", "
$ages
In this example, the array $ages
consists of numeric values. We apply [Array]::Sort()
to sort the array in ascending order and then use -join
to concatenate the sorted values into a string for better readability.
Output:
22, 28, 30, 35, 40
The [Array]::Sort()
method is a convenient way to sort arrays in place. It is particularly useful when you want to modify the original array directly.
However, it’s essential to note that this method is not suitable for sorting arrays of complex objects or custom types, where Sort-Object
would be a more appropriate choice.
Conclusion
In this article, we’ve uncovered two fundamental methods: the versatile Sort-Object
cmdlet and the straightforward [Array]::Sort()
method. Each method offers its unique advantages, catering to different scenarios and preferences in PowerShell scripting.
The Sort-Object
cmdlet stands out when dealing with arrays of custom objects or diverse data types with its flexible syntax and ability to sort objects based on properties. Its seamless integration into pipelines makes it a powerful tool for array manipulation, providing both ascending and descending sorting capabilities.
On the other hand, the [Array]::Sort()
method shines in simplicity and efficiency, directly modifying the original array in place. It’s ideal for scenarios where you prioritize modifying the existing array without creating additional variables.
However, it’s crucial to recognize its suitability primarily for sorting simple arrays of primitive data types.
Ultimately, whether you opt for the Sort-Object
cmdlet or the [Array]::Sort()
method depends on the specific requirements of your PowerShell script.