How to Pass an Array to a Function in PowerShell
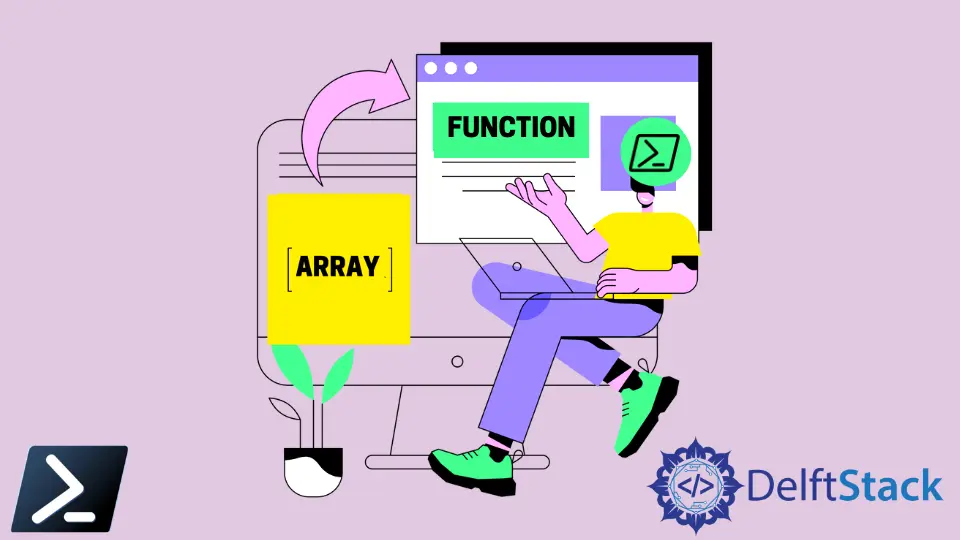
In many programming languages, including PowerShell, arrays are a fundamental data structure. They allow you to store collections of values efficiently, making them a crucial tool for various tasks in PowerShell scripting.
This article will focus on arrays and the convention of passing an array to a function in PowerShell.
PowerShell Arrays
PowerShell arrays are no different than those arrays in general-purpose programming languages such as Java, Python, C#, etc. They provide the capability to hold fixed-size collections of values or objects of any data type.
Syntax:
$intTypeArray = 34, 100, 1000, 45, 455, 1
To check the type of the variable $intTypeArray
in the following example, we will use the in-built GetType()
method.
As expected, the base type is System.Array
. Since we have not explicitly specified the data type of this array, the PowerShell engine has created it as an array of objects.
Interestingly, PowerShell arrays can accommodate diverse types of elements within a single array, as exemplified in the code snippet below.
Code:
$mixedElementArray = 200, 'stringElement', 12.555, 'hello'
Output:
As you can see, the default PowerShell array is based on the Object[]
type.
In PowerShell, every value or object is inherited from the Object
. So, any value or object is assignable to a default PowerShell array.
In addition to the default arrays, PowerShell offers a variant known as strongly typed arrays. These arrays exclusively accommodate collections of a specific data type.
When we create a strongly typed array, it is necessary to cast the reference variable to a specific array type such as int32[]
, string[]
, etc.
[string[]]$stringTypeArray = 'tesla', 'mecedes', 'audi', 'lambo'
Let’s check the type of $stringTypeArray
.
$stringTypeArray.GetType()
Output:
Pass an Array to a PowerShell Function
In PowerShell, strongly typed arrays are recommended to use in your PowerShell programs because it has type safety. Whenever you need to pass an already defined array to a function, the following syntax should work properly.
Syntax:
function <function_identifier>([<data_type>[]]$<parameter_name>)
{
}
In this syntax:
function
: Denotes the keyword used to initiate the definition of a function in PowerShell.<function_name>
: Represents the name of the function.[<data_type>[]]
: Specifies the data type of the parameter, allowing you to work with arrays of the specified data type inside the function.$<parameter_name>
: Serves as the name of the parameter within the function.
In this way, you can easily pass an array to function. To illustrate this, let’s first define a PowerShell function called letsPassAnArray
.
Code:
function letsPassAnArray([string[]]$stringList) {
foreach ($arrEle in $stringList) {
Write-Host $arrEle
}
}
In this example, we define a PowerShell function called letsPassAnArray
with a parameter named $stringList
. Then, we start a foreach
loop to iterate through each element ($arrEle
) in the $stringList
array.
Inside the loop, we write each array element to the console. To test the function, we create a string-type array named $stringArr
with elements 'Apple'
, 'Orange'
, and 'Grapes'
.
Code:
[string[]]$stringArr = 'Apple', 'Orange', 'Grapes'
letsPassAnArray($stringArr)
Finally, we call the letsPassAnArray
function and pass the $stringArr
array as an argument to it, causing the function to iterate through and print each element of the array.
Output:
Conclusion
In PowerShell, understanding arrays and how to pass them to functions is essential for efficient and organized scripting. Whether you’re working with generic arrays that can hold various data types or strongly typed arrays for type safety, mastering array manipulation will empower you to write more effective PowerShell scripts.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.