How to Count the Length of Array in PowerShell
- Understanding PowerShell Arrays
-
Use the
Length
Property to Count the Length of Array in PowerShell -
Use the
Count
Property to Count the Length of Array in PowerShell - Conclusion
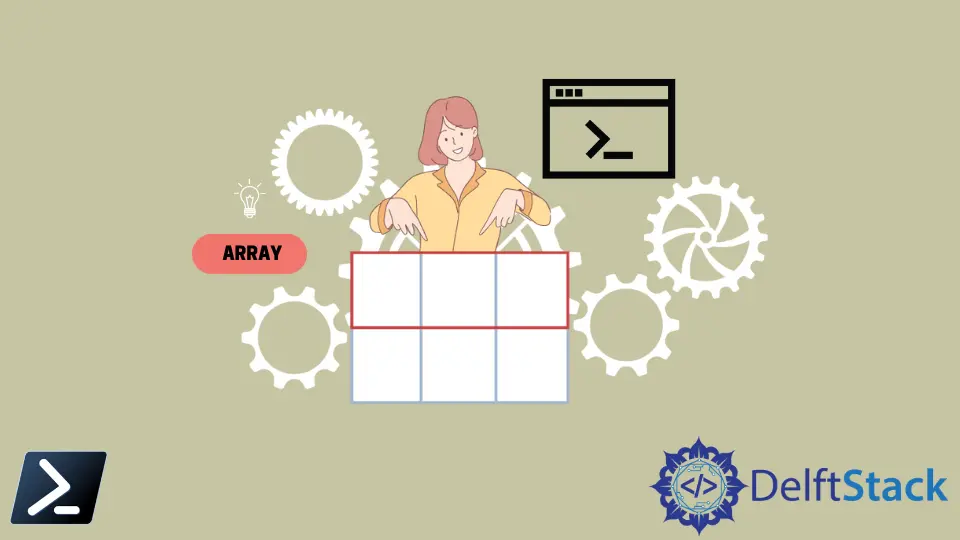
An array is a data structure storing a collection of items, which can be the same or different types.
You can assign an array by assigning multiple values to a variable. Its items can be accessed using an index number in brackets []
.
Sometimes, you might need to know how many items are stored in a PowerShell array. This tutorial will teach you to count the length of an array in PowerShell.
Understanding PowerShell Arrays
Before diving into how to count the length of an array in PowerShell, it’s essential to have a clear understanding of what arrays are and how they work.
An array is a container that holds a fixed number of items, which can be of any data type. Each item in an array is called an element, and each element is associated with a unique index.
PowerShell arrays are zero-indexed, meaning the first element is at index 0, the second element at index 1, and so on.
Arrays can be created by assigning multiple values to a variable, and their items can be accessed using index numbers enclosed in square brackets ([]
).
Use the Length
Property to Count the Length of Array in PowerShell
You can use the Length
property to determine how many items are stored in an array.
First, let’s create an array and assign values to it.
$array = "apple", "ball", "cat"
$array.GetType()
Output:
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True Object[] System.Array
Let’s use the Length
property to count the $array
length.
$array.Length
Output:
3
As you can see, it printed the accurate length of an array.
Handling Single-Item Arrays
Now, let’s create a single item array. You must use the comma (,
) operator or array subexpression syntax.
Syntax of comma operator:
$data = , "apple"
Syntax of array subexpression:
$data = @("apple")
The array items are placed in the @()
parentheses. An empty array will be created when no values are set in ()
.
Now, let’s use the Length
property.
$data.Length
Output:
1
If there are more than 2,147,483,647 elements in an array, the LongLength
property is handy.
Use the Count
Property to Count the Length of Array in PowerShell
Moreover, Count
is an alias for the Length
property. You can also use Count
to find the length of an array.
$data.Count
Output:
1
Conclusion
In PowerShell, efficiently managing arrays is crucial for various scripting tasks. Being able to determine the length of an array using the Length
and Count
properties is a valuable skill.
Whether you’re iterating through array elements or performing specific data manipulations, understanding these properties empowers you to work effectively with arrays in your PowerShell scripts.
Remember that PowerShell’s flexibility and array manipulation capabilities provide you with a versatile toolkit for handling and processing data collections.