How to Generate Random Strings Using PowerShell
-
Use the
Get-Random
Cmdlet to Generate Random Strings in PowerShell -
Use the
GeneratePassword()
Function to Generate Random Passwords in PowerShell -
Use the
New-Guid
Cmdlet to Generate Random Strings in PowerShell -
Use the
Get-Random
Cmdlet With Character Sets to Generate Random Strings in PowerShell - Conclusion
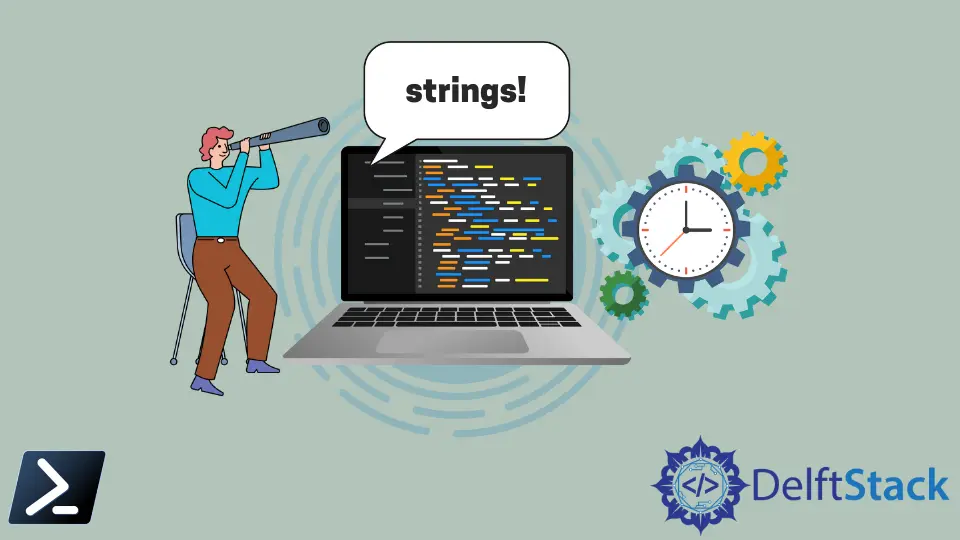
When managing Active Directory, tasks such as importing bulk users with unique temporary passwords can be challenging. Fortunately, PowerShell offers effective solutions for generating random strings to simplify these tasks.
In this article, we will explore how to achieve this using different methods in PowerShell.
Use the Get-Random
Cmdlet to Generate Random Strings in PowerShell
PowerShell provides a native command called Get-Random
, which selects random characters or numbers. When we provide an array of items to the Get-Random
command, it randomly picks one or more objects from that collection.
When creating passwords, we can invoke the Get-Random
cmdlet four times with different input
parameters (special characters, numbers, uppercase, and lowercase letters), concatenate the result strings, and shuffle them using another Get-Random
invoke.
In the example below, we define a hash table called $TokenSet
to store character sets for uppercase(U
), lowercase(L
), numbers(N
), and special characters(S
). This line of code $variablename = Get-Random -Count 5 -InputObject $TokenSet.U
generates a random selection of 5 characters from the character sets(U
, L
, N
, S
) and stores it in a variable($Upper
, $Lower
, $Number
, $Special
).
Then, we combine the randomly selected characters from each set into a single character set called $StringSet
. Lastly, we generate a random string of 15 characters by selecting from the $StringSet
, and then we join them together to form the final password.
Example Code:
$TokenSet = @{
U = [Char[]]'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
L = [Char[]]'abcdefghijklmnopqrstuvwxyz'
N = [Char[]]'0123456789'
S = [Char[]]'!"#$%&''()*+,-./:;<=>?@[\]^_`{|}~'
}
$Upper = Get-Random -Count 5 -InputObject $TokenSet.U
$Lower = Get-Random -Count 5 -InputObject $TokenSet.L
$Number = Get-Random -Count 5 -InputObject $TokenSet.N
$Special = Get-Random -Count 5 -InputObject $TokenSet.S
$StringSet = $Upper + $Lower + $Number + $Special
(Get-Random -Count 15 -InputObject $StringSet) -join ''
Output:
9ZimEXDW*@xo?12
The code above generates a different string each time. However, it involves multiple calls to the Get-Random
cmdlet, which can make the script longer and slower in terms of processing time.
The next section discusses how to achieve the same functionality in just a few lines of code.
Use the GeneratePassword()
Function to Generate Random Passwords in PowerShell
If you need to create password-specific strings, you can use the GeneratePassword()
function from the [System.Web.Security]
namespace. To use this function, you must first load the System.Web
library.
[Reflection.Assembly]::LoadWithPartialName("System.Web")
Once loaded, we can run the GeneratePassword()
function to generate a password randomly.
In the code below, we use the [System.Web.Security.Membership]
namespace to access the GeneratePassword()
function.
Example Code:
[System.Web.Security.Membership]::GeneratePassword(15, 2)
Output:
QQjj*LJ:e=YZ)Fo
As we can see from the example code above, the GeneratePassword()
function accepts two arguments:
-
The first argument takes an integer value representing the number of total characters needed for the password.
-
The second argument takes an integer value representing the number of special characters for the password.
However, this code doesn’t guarantee the presence of at least one numerical character in the password. To ensure that there’s at least one numerical character, you can use the following code.
Example Code:
do {
$pwd = [System.Web.Security.Membership]::GeneratePassword(15, 2)
} until ($pwd -match '\d')
$pwd
First, create a loop using the do
keyword to generate a password and ensure it contains at least one numerical character. Then, generate a random password with 15 characters, including 2 special characters, using this line of code [System.Web.Security.Membership]::GeneratePassword(15, 2)
and store it in the $pwd
variable.
The loop repeats until the generated password contains at least one numerical character.
Output:
Y4UkK4)G+lannRd
In just a few lines of code, the output above generates a different string each time and ensures that there’s at least one numerical character in the generated string.
Use the New-Guid
Cmdlet to Generate Random Strings in PowerShell
PowerShell provides the New-Guid
cmdlet to generate random GUIDs (Globally Unique Identifiers). You can use these GUIDs as random strings for various purposes, including passwords.
In the following example, we generate a random GUID (Globally Unique Identifier) using [System.Guid]::NewGuid()
, then convert the generated GUID to a string representation and store it in the $randomString
variable.
Example Code:
$randomString = [System.Guid]::NewGuid().ToString()
$randomString
Output:
de5881dc-a546-43da-9b89-0bae712406b1
The output will generate a random string.
Use the Get-Random
Cmdlet With Character Sets to Generate Random Strings in PowerShell
You can also generate random strings by defining custom character sets and using the Get-Random
cmdlet. This method allows more control over the character set used for the random strings.
Here’s an example.
Example Code:
$length = 10
$characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'.ToCharArray()
$randomString = -join ($characters | Get-Random -Count $length)
$randomString
First, we define the desired length of the random string and store it in a variable $length
.
Then, we define the characters($characters
) from which the random string will be generated as an array of characters. The .ToCharArray()
method converts the string into an array of its individual characters.
Next, we create a string ($randomString
) of the specified length by randomly choosing characters from the character array, and the selected characters are joined together to form the final random string. Lastly, we print the generated random string.
Output:
9lQSruF40i
The output will generate a random string by choosing the characters in the $characters
.
Conclusion
This article equips you with an array of methods to generate random strings in PowerShell, ensuring that you have the right tool for managing unique passwords and other tasks in Active Directory.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn