How to Extract Texts Using Regex in PowerShell
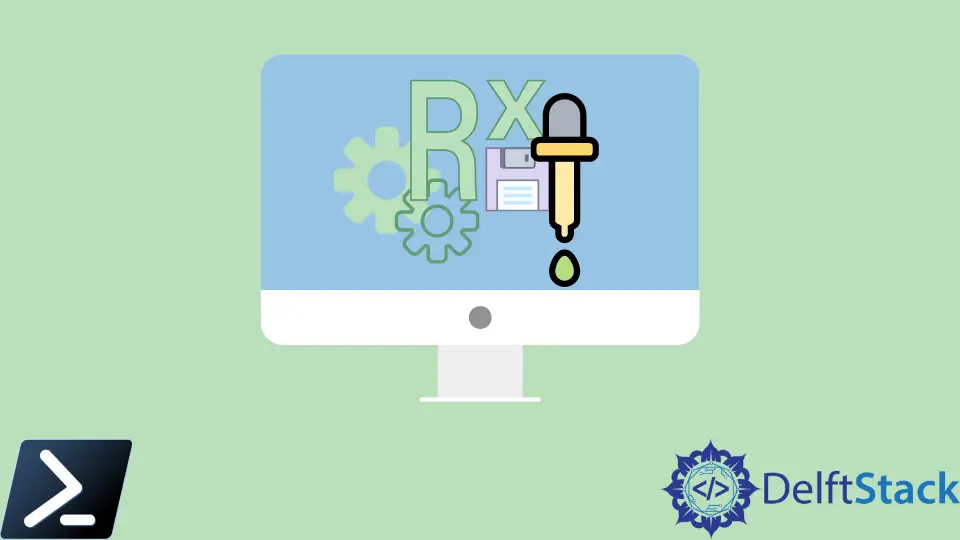
Sometimes we need to extract specific text from a large text with a specific pattern. Suppose you have a text like the one below and want to extract the emails from this text.
Alen <alen@gmail.com>, Stefen <stefen@gmail.com>, Walker <walker@gmail.com>
You must note that all the emails are enclosed by the <
and >
symbols.
The best way to do the task is to use the most popular PowerShell keyword, regex
. Using this keyword, you can provide a specific pattern that needs to match when performing the task.
This article will show how to extract a specific substring from a string using the keyword regex
. Also, we will see necessary examples and explanations to make the topic easier.
Extract Texts Using regex
in PowerShell
In our below-shared example, we will extract all the emails from the string. The code for our example will be the following:
$MyText = 'Alen <alen@gmail.com>, Stefen <stefen@gmail.com>, Walker <walker@gmail.com>'
$MyPattern = '(?<=\<).+?(?=\>)'
[regex]::Matches($MyText, $MyPattern).Value
In the first line of the example above, we first declared a string variable that will hold the given string. After that, we provided a specific pattern that needs to be matched.
The pattern is described in brief below:
(?<=\<)
- It’s the positive look-behind that matches only if the specified character precedes the text. However, it doesn’t include the characters within the match.+?
- captures (+
) of any character one or more. But only a few characters are possible.(?=\>)
- The positive look-ahead that matches only if the text follows the specified character. It doesn’t include the character within the matches.
Lastly, we match the pattern and extract the emails from the text.
After running the above example, you will get an output like the one below:
alen@gmail.com
stefen@gmail.com
walker@gmail.com
Please note that the example codes shared here are only executable on the Windows PowerShell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn