How to Extract a PowerShell Substring From a String
-
Extract a PowerShell Substring With the
Substring()
Method - Extract a PowerShell Substring at the Left of a String
- Extract a PowerShell Substring Before and After a Specified Character
- Extract a PowerShell Substring Between Two Characters
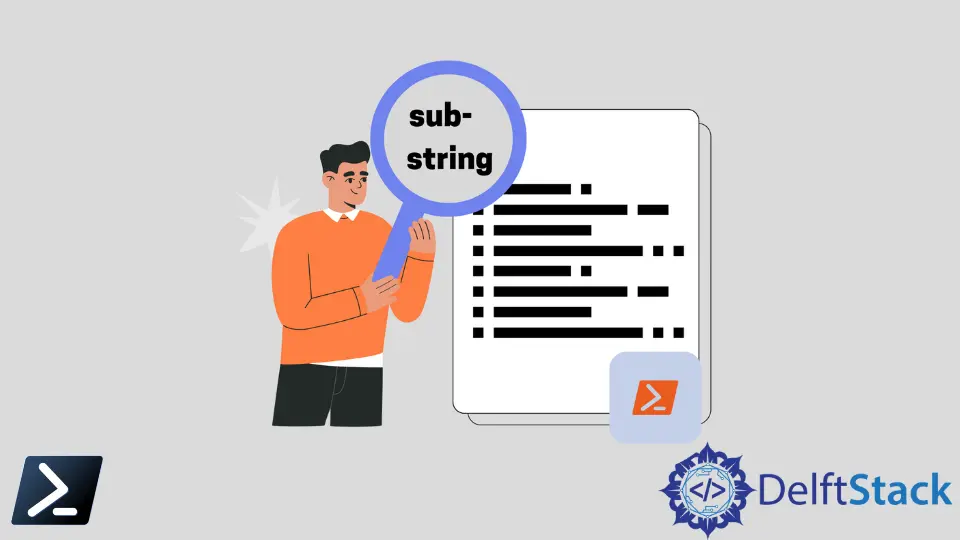
This article illustrates how we can extract a substring from a string on PowerShell. A substring is simply a part of a string.
We can create a substring in PowerShell using the Substring()
and split()
methods. For example, if we have the string Delftstack.com
, Delftstack
is a substring and com
is another.
This article will discuss the Substring()
method.
Extract a PowerShell Substring With the Substring()
Method
Let’s start by looking at the syntax of the Substring()
method.
string.Substring(int startIndex, int length)
The int
in the syntax marks the startIndex
and length
as index numbers. The startIndex
indicates the first character of the substring we want to extract, while length
is the number of characters we want to extract from a string.
We use the IndexOf
method to determine a substring’s startIndex
and length
.
We determine the startIndex
by adding 1
to the resulting value of the IndexOf
the substring. Let’s look at an example.
Assuming ned.delftstack.com
is our string, what is the first letter n
index position in our string? We can determine the position by running the command below:
"ned.delftstack.com".IndexOf('n')
The result is 0
. This is because the IndexOf
method searches for a string’s first occurrence from left to right and always starts from 0.
Hence, if we wish to extract a substring starting from the letter n
, our startIndex
will be 0.
We can also use LastIndexOf
to find the location of the last occurrence of a string. For example, the last occurrence of the letter d
would be:
"ned.delftstack.com".LastIndexOf('d')
This basic information will allow you to manipulate strings in PowerShell.
Extract a PowerShell Substring at the Left of a String
Let’s put the information above to work.
Suppose we want to extract the substring ned
from ned.delftstack.com
. How would we go about it?
We will begin by saving our string in a variable called ourstrng
.
$ourstrng = "ned.delftstack.com"
From the syntax of the Substring()
Method, our command will be:
$ourstrng.Substring(0, 3)
This will return ned
since our startIndex
is 0
and length
is 3 characters. What if our goal is to extract delftstack
from our string?
The first step is to determine the startIndex
for our substring. In this case, we will use the first period, which is the separator.
$ourstrng.IndexOf(".")
The result will be 3
. But if you can recall, we add 1
to the result for the string’s first character; hence, our startIndex
will be 4
.
The length will be the number of characters we want the substring to have; we will count from 1. The substring delftstack
has 10
characters.
$ourstrng.Substring(4, 10)
This will return delftstack
.
Extract a PowerShell Substring Before and After a Specified Character
Let’s say we want to extract both ned
and delftstack.com
from our string. How would we go about it?
First, we need to determine the position of our separator, the first .
. The command below will save the separator in a variable called $sepchar
.
$sepchar = $ourstrng.IndexOf(".")
To extract our substrings, we will run the command below:
$ourstrng.Substring(0, $sepchar)
This will extract the first part, which is ned
. For the other substring, we will run:
$ourstrng.Substring($sepchar + 1)
This will return deftstack.com
.
Extract a PowerShell Substring Between Two Characters
If our string is ned.delftstack.com
, our substring will be delftstack
since it is between our first and second periods. Such a script will require three commands.
The first command will determine the position of our first period (.)
and save it into the variable firstsep
.
$firstsep = $ourstrng.IndexOf(".")
The second command will determine the second position of our period using the LastIndexOf
method and save it into the variable lastrep
.
$lastrep = $ourstrng.LastIndexOf(".")
The last command will extract the substring delftstack
from our string.
$ourstrng.Substring($firstsep + 1, $lastrep - 4)
In conclusion, you can extract a substring in a string before, after, and between PowerShell separators. The Substring()
Method is handy, as we have seen above.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn