How to Check if a File Contains a Specific String Using PowerShell
-
Check if a File Contains a Specific String Using
Select-String
in PowerShell -
Check if a File Contains a Specific String Using
Get-Content
in PowerShell - Check Multiple Files that Contains a Specific String
- Conclusion
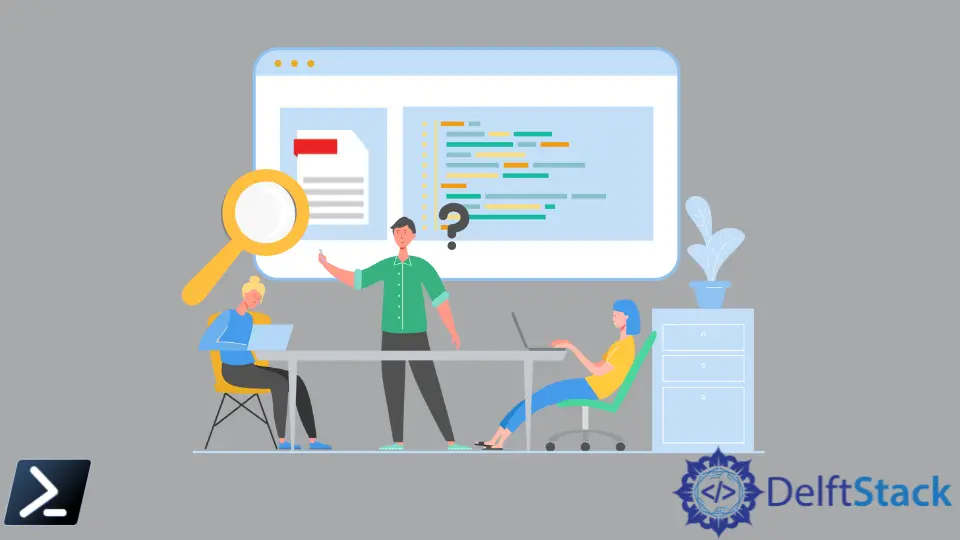
Searching for a specific string within a text file is a common task when working with Windows PowerShell. While Unix and Linux environments have the trusty grep
command, PowerShell offers an alternative approach using the Select-String
, Get-Content
, and Get-ChildItem
cmdlets.
In this article, we’ll explore various methods of how to use PowerShell to find a string in a text file.
Check if a File Contains a Specific String Using Select-String
in PowerShell
Let’s say we have a Demo.txt
file on our machine, and we want to find the string Demonstration
in it.
Here is the PowerShell script to do that:
Select-String -Path C:\Users\pc\Demo\Demo.txt -Pattern 'Demonstration'
In this script, we use Select-String
with the -Path
parameter to specify the file’s location and the -Pattern
parameter to specify the string we’re looking for.
If our file contains the specified pattern ('Demonstration'
), the console will output the file name, the line number, and the line containing the string.
Output:
C:\Users\pc\Demo\Demo.txt:1:This purely for demonstration
The output above displays the Demo.txt
file with its full path, the line containing our string (line 1
), and the line containing the string itself (This is purely for demonstration
).
But what if we only need to know if the file contains or does not contain the string? In such a case, we can modify the script as follows:
$Knw = Select-String -Path C:\Users\pc\Demo\Demo.txt -Pattern "Demonstration"
if ($Knw -ne $null) {
echo Contains String
}
else {
echo Does not Contain String
}
In this script, we defined a variable called $Knw
, which contains the result of the searched string "Demonstration"
in the file located at the specified path using the Select-String
. Then, we check if the variable $Knw
is not equal to $null
, which means that a match was found in the file.
If a match is found, it prints the "Contains String."
. If no match is found, it prints the "Does not Contain String."
.
Output:
Contains String
Since our file does contain the pattern "Demonstration"
, the output prints Contains String
.
Check if a File Contains a Specific String Using Get-Content
in PowerShell
Get-Content
is a cmdlet that fetches the contents of a specified path to a file and reads the contents of the file to return a string object. To use Get-Content
in our Demo.txt
file, we will use the script illustrated below:
Get-Content -Path C:\Users\pc\Demo\Demo.txt | Select-String -Pattern "Demonstration"
In this script, we use the Get-Content
to read the contents of our Demo.txt
file specified by the -Path
parameter and forward it to the Select-String
command, which uses the -Pattern
parameter to locate the Demonstration
string.
Output:
This purely for demonstration
If we only want to confirm the presence of the string, we can use the script shown below:
If (Get-Content C:\Users\pc\Demo\Demo.txt | % { $_ -match "Demonstration" }) {
echo Contains String
}
else {
echo Does not Contains String
}
In this script, we use Get-Content
to read the data of the file located at C:\Users\pc\Demo\Demo.txt
and a pipeline (|
) to process each line of the file one by one. Inside the %{}
block, we check if each line contains the string "Demonstration"
using the -match
operator.
If any line in the file contains "Demonstration"
, it prints "Contains String"
. If no line in the file contains "Demonstration"
, it prints "Does not Contains String"
.
Output:
Contains String
Since our file contains the specified string ("Demonstration"
), we get the Contains String
as the output.
Check Multiple Files that Contains a Specific String
What if you have over fifty text files, and you want to search for a string in all of them? We will discuss two ways to do it.
Using Get-ChildItem
and Search-String
in PowerShell
We can use the Get-ChildItem
and Select-String
cmdlets. The Get-ChildItem
cmdlet will find one or more files based on our search criteria.
Let’s look at an example. Assuming our Demo
folder has over fifty text files, we can search for the string Demonstration
in all of them using this script:
Get-ChildItem -Path C:\Users\pc\Demo\ -Recurse | Select-String -Pattern 'Demonstration'
In this script, we use Get-ChildItem
to list files in the specified directory (-Path
) and its subdirectories (-Recurse
). The pipe operator (|
) passes the list of files to the Select-String
cmdlet.
Lastly, we use Select-String
to search for a specific pattern, 'Demonstration'
in each file.
The console will output the file names containing our string, the line numbers, and the lines containing the string.
Output:
C:\Users\pc\Demo\Demo.txt:1:This purely for demonstration
C:\Users\pc\Demo\Insert.txt:1:We will use this demonstration for a test trial
C:\Users\pc\Demo\myfile.txt:1:This is a demonstration file for Get-ChildItem
Using Select-String
in PowerShell
We can also use Select-String
only to check the specific string on multiple files.
Select-String -Path C:\Users\pc\Demo\*.txt -Pattern 'Demonstration'
In this script, we use the wildcard (*
) in the -Path
parameter to search for all text files in the Demo
folder. The Select-String
cmdlet will search for the string in all matching files.
Output:
C:\Users\pc\Demo\Demo.txt:1:This purely for demonstration
C:\Users\pc\Demo\Insert.txt:1:We will use this demonstration for a test trial
C:\Users\pc\Demo\myfile.txt:1:This is a demonstration file for Get-ChildItem
Same on the above, the console will output the file names containing our string, the line numbers, and the lines containing the string.
Conclusion
Windows PowerShell provides a set of tools for efficiently searching for strings in text files. Whether you prefer using the Select-String
, Get-Content
, or a combination of Get-ChildItem
and Select-String
, you can easily locate and manipulate data within your files.
With these cmdlets, you’ll have no trouble finding the information you need, making text file searches in PowerShell effortless.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn