How to Write Array to a File in PHP
-
Use the
print_r()
andfile_put_contents()
Functions to Write the Array to the File in PHP -
Use
fopen()
,print_r()
andfwrite()
Functions to Write the Array to the File in PHP -
Use
fopen()
,var_export()
andfwrite()
Functions to Write the Array to the File
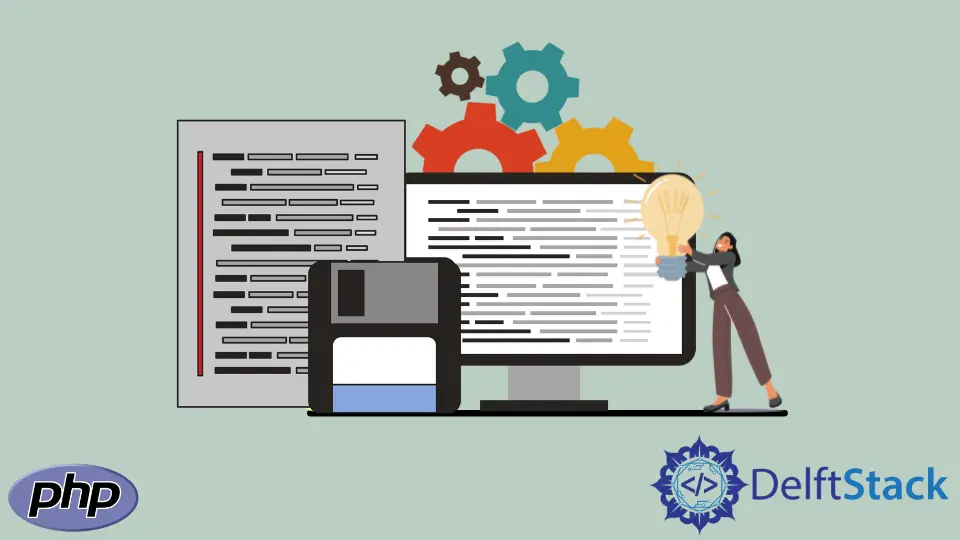
We will introduce a way to write an array to a file in PHP using the print_r()
and the file_put_contents()
functions. This method writes the specified array to the specified file in the system.
We will also introduce a way to print an array to a PHP file using the print_r()
and the fwrite()
functions. We use the fopen()
function to create the file.
We will introduce a permanent solution to display all the PHP errors, changing the php.ini
file. The two methods mentioned above won’t help display the parse errors like missing curly braces and semicolons.
Use the print_r()
and file_put_contents()
Functions to Write the Array to the File in PHP
This method can create an array and use the print_r()
function to return the array and use the file_put_content()
function to load the array to the file. The print_r()
function takes the array to be printed and the boolean value as the parameters. The boolean value determines whether the array will be returned. The default value is false. The file_put_contents()
function takes the file path as the first parameter. The second parameter is the content to be loaded in the file.
For example, create an associative array. An associative array is the type of array where the keys are strings instead of numbers. Create the keys as name
, age
, and bike
. Assign the values Jack
, 24
, and fireblade
as the keys’ value. Assign the array to a variable $b
. Use the print_r()
function and take the parameters $b
and a boolean value of true
. Then, write the file_put_contents()
function and specify a file filename.txt
as the first parameter and the print_r()
function above as the second parameter.
In the example below, the print_r()
function returns the array’s information. It does not print the items on the webpage. A file filename.txt
will be created in the root directory where the array is printed. Check the print_r()
in PHP manual to know more about the function.
Example Code:
# php 7.*
<?php
$b = array (
'name' => 'Jack',
'age' => 24,
'bike' => 'fireblade');
file_put_contents('filename.txt', print_r($b, true));
?>
Output:
Array
(
[name] => Jack
[age] => 24
[bike] => fireblade
)
Use fopen()
, print_r()
and fwrite()
Functions to Write the Array to the File in PHP
We can use the fwrite()
method to write the array to the file. This method creates a writable file in the directory with the fopen()
function. We can create an array and use print_r()
function to return the array and use the fwrite()
function to write the array to the file. The print_r()
function takes the array to be printed and the boolean value as the parameters.
Use the fopen()
function to create a file file.txt
with w
as the second parameter. Assign the fopen()
function to a variable $fp
. Use the print_r()
function and take the parameters $b
and a boolean value of true
. Then, write the fwrite()
function and provide the variable $fp
as the first parameter and the print_r()
function above as the second parameter.
In the example below, the fopen()
function creates a file file.txt
in the root directory. The array is written to the file using the fwrite()
function. Check the PHP manual to learn more about the fwrite()
function.
Example Code:
#php 7.x
<?php
$b = array (
'name' => 'Harry',
'age' => 22,
'bike' => 'hayabusa');
$fp = fopen('file.txt', 'w');
fwrite($fp, print_r($b, true));
fclose($fp);
?>
Output:
Array
(
[name] => Harry
[age] => 22
[bike] => hayabusa
)
Use fopen()
, var_export()
and fwrite()
Functions to Write the Array to the File
We use the var_export()
function instead of the print_r()
function in this method to return the array that needs to be printed. The var_export()
function takes the array to be printed and the boolean value as the parameters just like in the print_r()
function . We create a writable file in the directory with fopen()
function. We use the fwrite()
function to write the array to the file by taking the array and the var_export()
function as the parameters. The var_export()
function returns the valid PHP code of the specified parameter.
For example, write the fwrite()
function and provide the variable $fp
as the first parameter and the var_export()
function above as the second parameter. Supply the variable $b
and the boolean value true
as the parameters for the var_export()
function.
In the example below, var_export()
function results a different output in terms of structure rather than the print_r()
function. The array printed by the var_export()
function is a valid PHP code and can be excuted unlike the output genereated by the print_r()
function. Please check the PHP Manual to understand about the var_export()
function.
Code Example:
#php 7.x
<?php
$b = array (
'name' => 'James',
'age' => 27,
'bike' => 'Yamaha R1');
$fp = fopen('file.txt', 'w');
fwrite($fp, var_export($b, true));
?>
Output:
array (
'name' => 'James',
'age' => 27,
'bike' => 'Yamaha R1',
)
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedInRelated Article - PHP File
- How to Write Into a File in PHP
- How to Require_once vs Include in PHP
- How to Create and Get the Path of tmpfile in PHP
- How to Remove PHP Extension With .Htacess File
- How to Move File to a Folder in PHP
- How to Set End of Line in PHP With PHP_EOL