How to Move File to a Folder in PHP
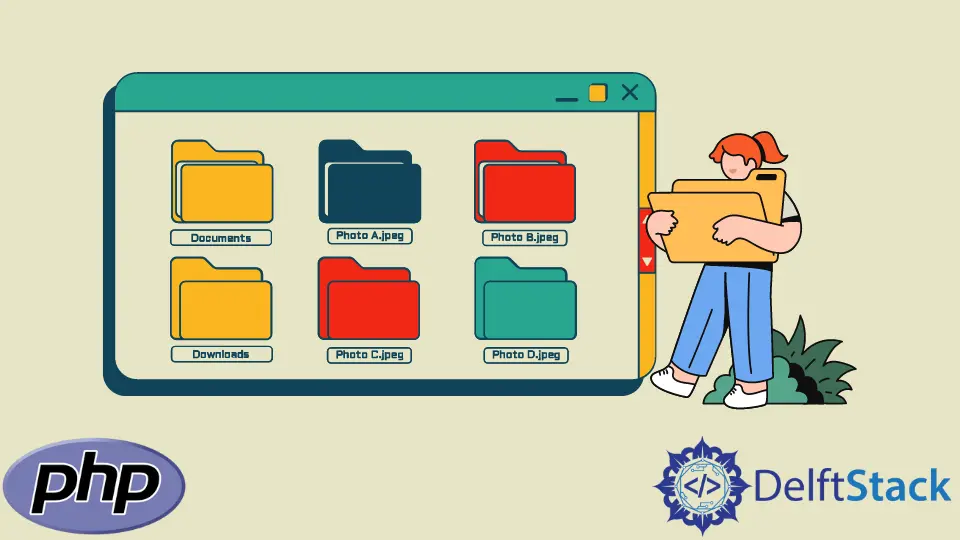
This article will explain the method to move a file from one folder to another. It might seem tricky, but it is super easy to do so. All you need is to practice the PHP’s native function rename
. Its name implies that it works by renaming a file or directory. It might confuse you at first, but renaming the path of a file ultimately moves it to the new location.
Use the rename()
Function to Move File to Another Folder in PHP
In order to use the rename
function, we need to specify the source and destination locations of the file, which is to be moved. The source location is the current directory where the file is kept, and the destination location is where we want to move our file. Let’s discuss it with the help of an example!
Suppose we have two directories named source_directory
and dest_directory
. We have a file called move-test.txt
in the source_directory
, and we wish to move it to dest_directory
using PHP.
Let’s have a look at the following code:
<?php
$currentLocation = 'source_directory/move-test.txt';
$newLocation = 'dest_directory/move-test.txt';
$moved = rename($currentLocation, $newLocation);
if($moved)
{
echo "File moved successfully";
}
?>
The explanation of the above-mentioned code is as follows.
- The current path of the file is specified first using the variable
$currentLocation
. - Then, the new location along with the filename is specified. In simpler words, it is the new location where we wish to move our file to.
- If you wish to give your file a different name, it can be done by changing the filename
move-test.txt
in the variable$newLocation
with your new filename. - The rename function takes two parameters:
$currentLocation
for the file path before moving operation and$newLocation
for the new file path after successfully moving it to the desired location.
Important Considerations
The rename
function returns TRUE
, if the move operation is successful. Two important things to note here are:
- The file to be moved must exist. You should make sure that the file you are trying to move must exist. Otherwise, it will throw a warning stating:
The system cannot find the file specified.
- An existing file can be overwritten. The rename function can result in the overwriting of an existing file, which means if
$newLocation
is the name of some existing file, then after the move operation with rename function, the existing file will be overwritten.
to Check if File Exists
In order to check whether the file exists or not before moving it to another folder, you can use the is_file
function provided by PHP.
The code example to check if the file exists or not using the is_file
function is as follows:
<?php
$currentLocation = 'source_directory/move-test.txt';
$newLocation = 'dest_directory/move-test.txt';
if(is_file($currentLocation))
{
$moved = rename($currentLocation, $newLocation);
}
if($moved)
{
echo "File moved successfully";
}
?>
In the above example, is_file($currentLocation)
is used to check whether the file we wish to move exists or not before calling rename function that performs the move operation.
to Check if File Is Not Overwritten
The method to ensure that an existing file is not overwritten while moving a file from one location to another is explained in the following code example:
<?php
$currentLocation = 'source_directory/move-test.txt';
$newLocation = 'dest_directory/move-test.txt';
if(!is_file($newLocation))
{
$moved = rename($currentLocation, $newLocation);
}
if($moved)
{
echo "File moved successfully";
}
?>
In the above example, is_file($newLocation)
is used to check if another file exists that could be overwritten when the rename
function is called.