How to Write Into a File in PHP
-
Use
file_put_contents()
Function to Write Into a File in PHP -
Use
fopen()
,fwrite()
andfclose()
Functions to Write Into a File in PHP
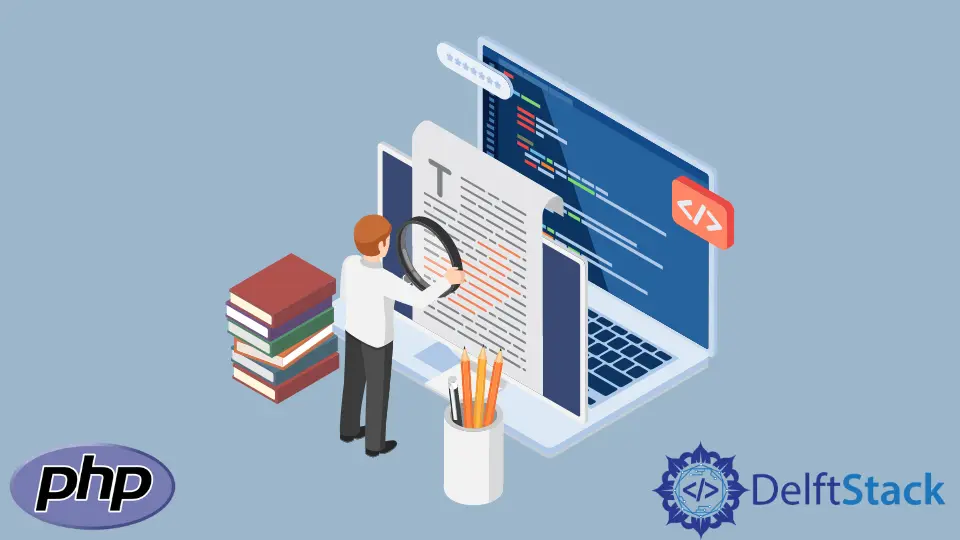
In this article, we will introduce methods to write into a file in PHP.
- Using
file_put_contents()
function - Using
fopen()
,fwrite()
andfclose()
functions
Use file_put_contents()
Function to Write Into a File in PHP
The built-in function file_put_contents()
writes the data into a file in PHP. It searches for the file to write in, and if the desired file is not present, it creates a new file. We can use this function to write into a file. The correct syntax to use this function is as follows.
file_put_contents($pathOfFile, $info, $customContext, $mode);
This function accepts four parameters. The detail of these parameters is as follows.
Parameter | Description | |
---|---|---|
$pathOfFile |
mandatory | Path of the file. |
$info |
mandatory | Data to write in a file. It can be a string. |
$customContext |
optional | Specify a custom context. |
$mode |
optional | The mode in which the data will be written on the file. It can be FILE_USE_INCLUDE_PATH , FILE_APPEND , and LOCK_EX . |
This function returns the number of bytes written on the file if successful, or False
if it fails.
The following program will write the data into a file.
<?php
$data = "This is a program";
$bytes = file_put_contents("myfile.json", $data);
echo "The number of bytes written is $bytes.";
?>
Output:
The number of bytes written is 17.
Use fopen()
, fwrite()
and fclose()
Functions to Write Into a File in PHP
The built-in functions fopen()
, fwrite()
and fclose()
are used to open a file, write into a file and close a file. The correct syntax to use these functions is as follows
fopen($fileName, $mode, $path, $context);
This function has four parameters, and their details are as follows.
Parameters | Description | |
---|---|---|
$fileName |
mandatory | It is the name of the file to open. |
$mode |
mandatory | It is the mode of the file. There are several modes, check here. |
$path |
optional | It is the path to search file. |
$context |
optional | It is used to set the context of the file. |
The possible modes include,
mode |
Description |
---|---|
r |
Read only |
r+ |
Read and write |
w |
Write only. If the file does not exist, attempt to create it. |
w+ |
Read and write. If the file does not exist, attempt to create it. |
a |
Append. |
a+ |
Read and append. |
x |
Create and write only. |
x+ |
Create and read and write |
fwrite($fileName, $info, $length);
It has three parameters. The details of its parameters are as follows.
Parameter | Description | |
---|---|---|
$fileName |
mandatory | It is the file to write in. |
$info |
mandatory | It is the information that will be written in the file. |
$length |
optional | It is the number of bytes to be written in the file. |
fclose($fileName);
This function accepts only one parameter that is the name of the file to be closed. It returns True
on success and False
on failure.
The below program writes the data into a file.
<?php
$myfile = fopen("myfile.txt", "w");
$bytes = fwrite($myfile, "This is a program");
fclose($myfile);
echo "The number of bytes written is $bytes.";
?>
Here the mode in fopen()
function is set to w
that means the file is opened for writing only.
Output:
The number of bytes written is 17.