How to Require_once vs Include in PHP
-
When to Use the
include
vs.require
Keywords in PHP -
When to Use the
require_once
vs.include
Keywords in PHP
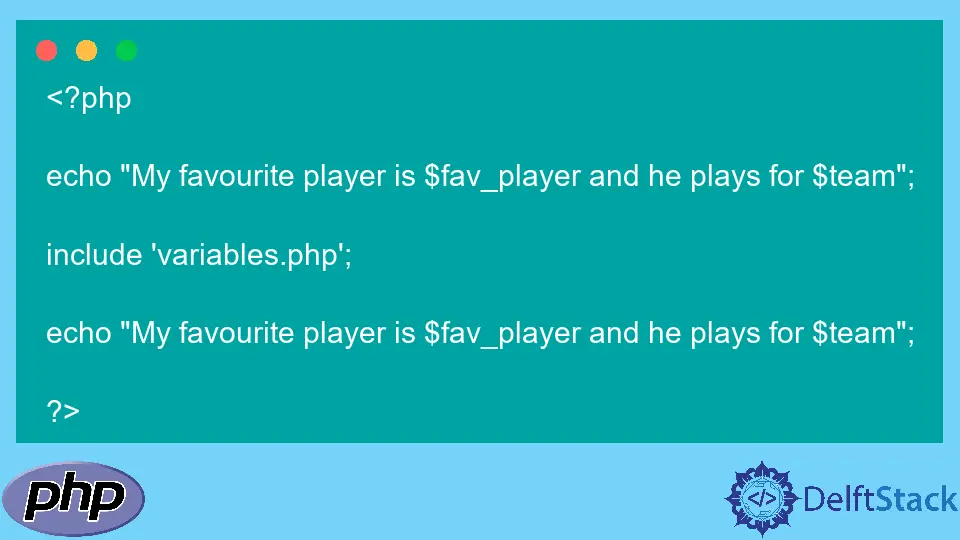
For developers, during the development cycle, we separate different PHP files across our development or production directory, but we might need a function or class in another PHP file. It would be counterintuitive and against the DRY approach to repeat that same class or function in another file.
Therefore, PHP has four keywords to add or give access to the content of another PHP file, include
, require
, include_once
, and require_once
. In this article, we will consider all keywords, but we’ll focus more on require_once
and include
.
When to Use the include
vs. require
Keywords in PHP
The keywords include
and require
copy all of the content, text or code, or markup, from the specified PHP file into the include statement’s target PHP file. However, the include
keyword behaves differently from require
in handling failure.
require
produces a fatal error (E_COMPILE ERROR
) and stops the script.include
produces only a warning (E_WARNING
), and the script continues even if the next section needs the code from the PHP file you included.
Both keywords are the same way if no error occurs.
Let’s try to add a simple PHP file (variables.php
) that holds a couple of variables to our current PHP file.
The variables.php
file:
<?php
$team = 'Golden State Warriors';
$fav_player = 'Steph Curry';
?>
Now, let us use the include
keyword to add the content of the variables.php
:
<?php
echo "My favourite player is $fav_player and he plays for $team";
include 'variables.php';
echo "My favourite player is $fav_player and he plays for $team";
?>
The output of the above code snippet:
My favourite player is and he plays for
My favourite player is Steph Curry and he plays for Golden State Warriors
As you can see, the first line doesn’t output the contents of the variables because we have not included the variables.php
file, but once we add it, it has the contents of the variables.
Now, let’s use the require
keyword:
<?php
echo "My favourite player is $fav_player and he plays for $team";
include 'variables.php';
echo "My favourite player is $fav_player and he plays for $team";
?>
Output:
My favourite player is and he plays for
My favourite player is Steph Curry and he plays for Golden State Warriors
The same output was produced. Now, let’s misspell the variables.php
file in our code and see the effect.
For the include
keyword:
My favourite player is and he plays for
Warning: include(variable.php): failed to open stream: No such file or directory in /home/runner/Jinku/index.php on line 5
Warning: include(): Failed opening 'variable.php' for inclusion (include_path='.:/nix/store/bq7pj5lz7rq92p3d3qyy25lpzic9phy5-php-7.4.21/lib/php') in /home/runner/Jinku/index.php on line 5
My favourite player is and he plays for
For the require
keyword:
My favourite player is and he plays for
Warning: require(variable.php): failed to open stream: No such file or directory in /home/runner/Jinku/index.php on line 5
Fatal error: require(): Failed opening required 'variable.php' (include_path='.:/nix/store/bq7pj5lz7rq92p3d3qyy25lpzic9phy5-php-7.4.21/lib/php') in /home/runner/Jinku/index.php on line 5
You can now see the difference between both. With the include
keyword, the code continued executing even though the file included wasn’t present and only gave a warning.
However, with the require
keyword, the code produced a Fatal error and stopped completely at that point.
When to Use the require_once
vs. include
Keywords in PHP
There are appropriate areas to use include
or require
. It is more advisable to use require
all the time; however, if your application can do without the content in the PHP file we intend to add to the current PHP file, there might not be an issue.
To give more context to understand the include
, require
, and require_once
keywords, let’s try an example. For this example, we will create a functions.php
file that will be included and required to showcase the difference.
The functions.php
file:
<?php
function add_sums($a, $b) {
return $a + $b;
}
?>
Let’s use include
:
<html>
<head>
<title>PHP Test</title>
</head>
<body>
<?php
include 'functions.php';
echo "The program is starting\n";
$sum = add_sums(2,3);
echo "The program is done\n";
echo $sum;
?>
</body>
</html>
The output of the code snippet:
The program is done 5
Let’s use require
:
<html>
<head>
<title>PHP Test</title>
</head>
<body>
<?php
require 'functions.php';
echo "The program is starting\n";
$sum = add_sums(2,3);
echo "The program is done\n";
echo $sum;
?>
</body>
</html>
The output of the code snippet:
The program is done 5
However, if the functions.php
file is unavailable, and we use include
, the following will be the output:
Warning: include(functions.php): failed to open stream: No such file or directory in /home/runner/Jinku/index.php on line 7
Warning: include(): Failed opening 'functions.php' for inclusion (include_path='.:/nix/store/bq7pj5lz7rq92p3d3qyy25lpzic9phy5-php-7.4.21/lib/php') in /home/runner/Jinku/index.php on line 7
The program is starting
Fatal error: Uncaught Error: Call to undefined function add_sums() in /home/runner/Jinku/index.php:9 Stack trace: #0 {main} thrown in /home/runner/Jinku/index.php on line 9
Though the functions.php
was not there, the code was still running until it hit a Fatal error due to a call to an undefined function, which is not an effective means to work within PHP applications.
However, when we use require
, the error looks like this:
Warning: require(functions.php): failed to open stream: No such file or directory in /home/runner/Jinku/index.php on line 7
Fatal error: require(): Failed opening required 'functions.php' (include_path='.:/nix/store/bq7pj5lz7rq92p3d3qyy25lpzic9phy5-php-7.4.21/lib/php') in /home/runner/Jinku/index.php on line 7
Because we used the require
keyword, we could prevent the fatal error due to a call to an undefined function. For rather complex PHP applications, require
will be more appropriate to be able to catch errors quickly and prevent unwanted consequences.
Now, let’s take it a step further. Let’s use the require_once
keyword:
<html>
<head>
<title>PHP Test</title>
</head>
<body>
<?php
// Previous code START
require_once 'functions.php';
echo "The program is starting\n";
$sum = add_sums(2,3);
echo "The program is done\n";
echo $sum;
// Previous code ENDS
// Additional code STARTS
echo "Another program begins";
require 'functions.php';
$sum = add_sums(2,3);
echo $sum;
echo "Another program ends";
?>
</body>
</html>
The output of the above code snippet would produce a fatal error:
The program is starting
The program is done
5
Another program begins
Fatal error: Cannot redeclare add_sums() (previously declared in /home/runner/Jinku/functions.php:3) in /home/runner/Jinku/functions.php on line 3
The require_once
keyword will check if the file has been included, and if so, not include or require it again. Therefore, because we used the require_once
keyword earlier, it prevents requiring or even including the code from functions.php
.
You might want to do this to prevent redeclaration of function calls or variable value assignments, which can be costly for your code.
In the example, if we used require
twice, it will call the function again and produce the same effect as before, resulting in a value of 5.
Combinations to understand:
require
andrequire_once
will throw a fatal error.include
andinclude_once
will throw a fatal error.require_once
andrequire_once
will work fine, but all the content will be recalled and redeclared.include_once
andinclude_once
will work fine, but all the content will be recalled and redeclared.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn