How to Determine the First and Last Iteration in a Foreach Loop in PHP
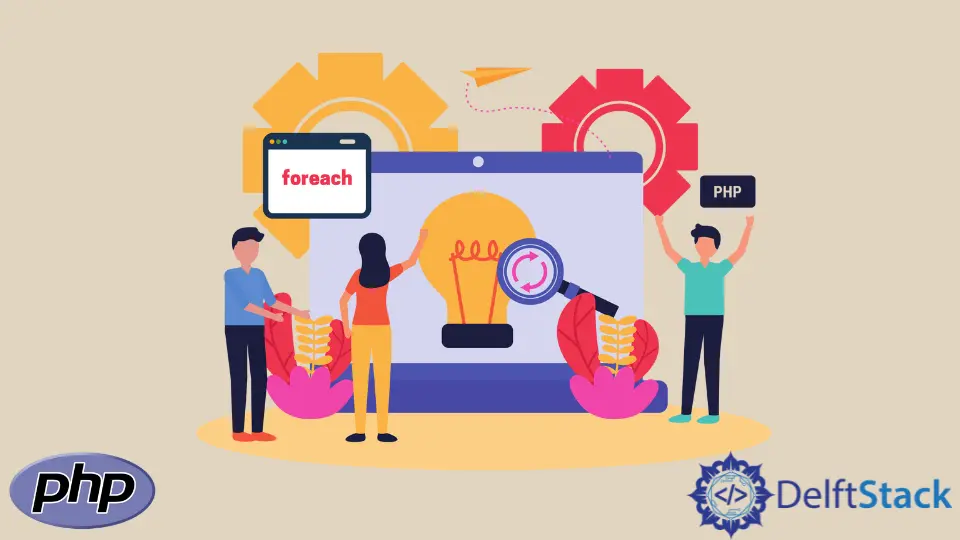
PHP foreach()
loop is being used to loop through a block of code for each element in the array. In this article, we’ll figure out how to use foreach()
loop and how to get the first and last items of an array.
PHP foreach()
Syntax
The foreach()
loop is mainly used for iterating through each value of an array.
Example of foreach()
loop:
for($array as $key => $value){
// Add code here
}
The foreach()
parameters meanings:
$array
- This must be a valid array to use in a loop; If the array passed isn’t valid or a string, return an error like: (Warning: Invalid argument supplied for foreach()).$key
- It is the element’skey
on each iteration.$value
- It is the element’s value on each iteration.
Get the First and Last Item in a foreach()
Loop in PHP
There are several approaches to get the first and last item in a loop using PHP and it varies on different versions.
These are, by using counter (works for all version), using array_key_first()
and array_key_last()
for PHP 7.3.
Use Counter in PHP foreach
Loop
Adding an integer variable and placing the counter at the bottom of the foreach()
loop.
Example:
$array = array("dog", "rabbit", "horse", "rat", "cat");
$x = 1;
$length = count($array);
foreach($array as $animal){
if($x === 1){
//first item
echo $animal; // output: dog
}else if($x === $length){
echo $animal; // output: cat
}
$x++;
}
In the above example, the list of animals is stored as an array.
Then set the $x
as 1 to start the counter.
Use count()
to determine the total length of an array. The iteration of the counter was placed at the bottom of the foreach()
loop - $x++;
to execute the condition to get the first item.
To get the last item, check if the $x
is equal to the total length of the array. If true
, then it gets the last item.
Use array_key_first()
and array_key_last()
in PHP Loop
With the latest version of PHP, getting the first and last item in an array can never be more efficient.
Example:
$array = array("dog", "rabbit", "horse", "rat", "cat");
foreach($array as $index => $animal) {
if ($index === array_key_first($array))
echo $animal; // output: dog
if ($index === array_key_last($array))
echo $animal; // output: cat
}
In the example above, array_key_first()
gets the key of the first item in the array, in this case, it’s 0
.
Then array_key_last()
gets the last key of the array, in this case, 5
.
By inspecting the array, the item that is sitting in the 0
key is the dog
which is the first and in the 5th
key, is the cat
which is the last item.
Related Article - PHP Loop
- How to Loop Through Associative Array in PHP
- How to Break Out of the Foreach Loop in PHP
- Performance and Readability of for Loop and foreach Loop in PHP
- How to Use Foreach Loop in Multidimensional Array in PHP
- How to Check foreach Loop Key Value in PHP