How to Convert an Array to a String in PHP
-
Use
implode()
Function to Convert an Array to a String in PHP -
Use
json_encode()
Function to Convert an Array to a String in PHP -
Use
serialize()
Function to Convert an Array to a String in PHP
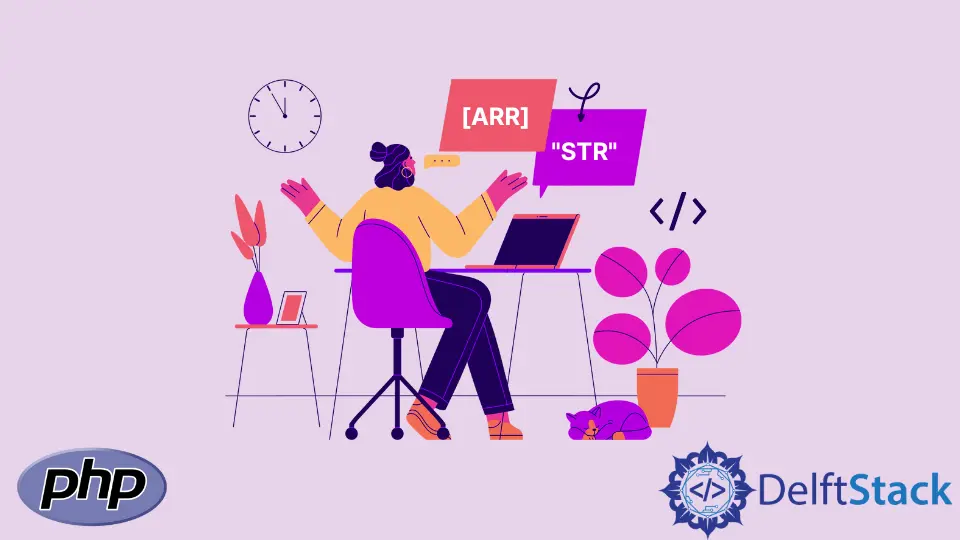
In this article, we will introduce methods to convert an array to a string.
- Using
implode()
function - Using
json_encode()
function - Using
serialize()
function
Use implode()
Function to Convert an Array to a String in PHP
The implode()
function converts an array to a string. It returns the string that has all the elements of the array. The correct syntax to use this function is as follows
implode($string, $arrayName);
The variable $string
is the separator to separate the elements of the array. The variable $arrayName
is the array to be converted.
<?php
$arr = array("This","is", "an", "array");
$string = implode(" ",$arr);
echo "The array is converted to the string.";
echo "\n";
echo "The string is '$string'";
?>
Here we have passed a white space string as a separator to separate the elements of the array.
Output:
The array is converted to the string.
The string is 'This is an array'
Use json_encode()
Function to Convert an Array to a String in PHP
The json_encode()
function is used to convert an array to a json string. json_encode()
also converts an object to json string.
json_encode( $ArrayName );
The variable ArrayName
shows the array to be converted to a string.
<?php
$array = ["Lili", "Rose", "Jasmine", "Daisy"];
$JsonObject = json_encode($array);
echo "The array is converted to the JSON string.";
echo "\n";
echo"The JSON string is $JsonObject";
?>
Output:
The array is converted to the JSON string.
The JSON string is ["Lili","Rose","Jasmine","Daisy"]
Use serialize()
Function to Convert an Array to a String in PHP
The serialize()
function converts an array to a string effectively. It also returns the index value and string length along with each element of the array.
serialize($ArrayName);
The function accepts the array as a parameter and returns a string.
<?php
$array = ["Lili", "Rose", "Jasmine", "Daisy"];
$JsonObject = serialize($array);
echo "The array is converted to the JSON string.";
echo "\n";
echo"The JSON string is $JsonObject";
?>
Output:
The array is converted to the JSON string.
The JSON string is a:4:{i:0;s:4:"Lili";i:1;s:4:"Rose";i:2;s:7:"Jasmine";i:3;s:5:"Daisy";}
The output is an array with the description that tells
- The number of elements in an array
a:4
, the array has 4 elements - The index of each element and the element length
i:0;s:4:"Lili"
;
Related Article - PHP Array
- How to Determine the First and Last Iteration in a Foreach Loop in PHP
- How to Get the First Element of an Array in PHP
- How to Echo or Print an Array in PHP
- How to Delete an Element From an Array in PHP
- How to Remove Empty Array Elements in PHP