How to Get the First Element of an Array in PHP
- Accessing the First Element Using Index
-
Using the
reset()
Function -
Using the
current()
Function - Conclusion
- FAQ
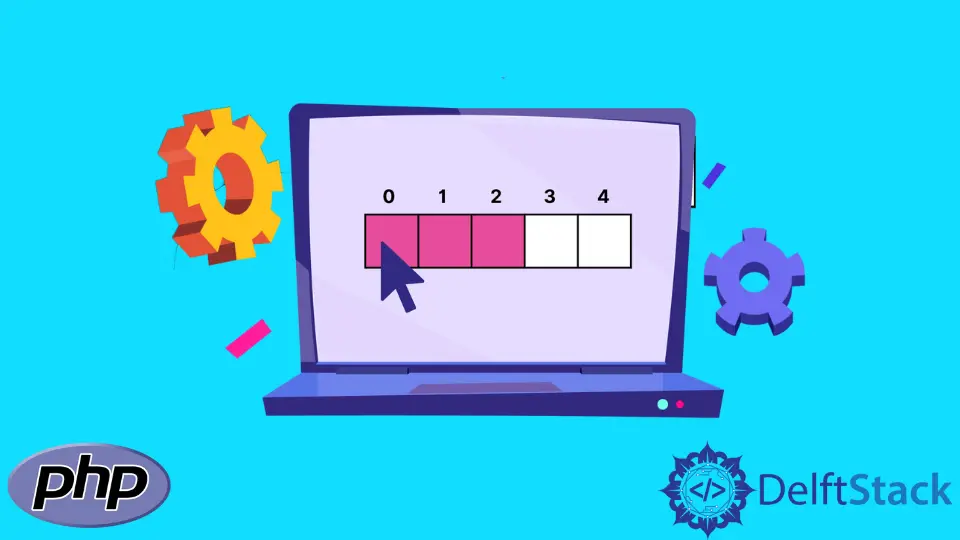
When working with arrays in PHP, retrieving the first element is a common task that developers often encounter. Whether you’re building a simple application or working on a complex project, knowing how to access the first item in an array can streamline your coding process.
This article will guide you through various methods to achieve this, including using the index of the elements, the reset()
function, and the current()
function. By the end of this article, you’ll have a solid understanding of how to efficiently retrieve the first element of an array in PHP, enhancing your coding skills and efficiency.
Accessing the First Element Using Index
One of the simplest ways to get the first element of an array in PHP is by using its index. In PHP, array indices start at 0, meaning the first element is always located at index 0. Here’s how you can do it:
$array = ["apple", "banana", "cherry"];
$firstElement = $array[0];
echo $firstElement;
Output:
apple
In this example, we define an array containing three fruit names. To access the first element, we simply refer to $array[0]
. This method is straightforward and efficient, especially for developers familiar with the concept of indexing in arrays. It’s important to ensure that the array is not empty before attempting to access an index, as this would result in an undefined index notice. This method is particularly useful when you know the structure of your array and need quick access to its first element.
Using the reset()
Function
Another effective way to retrieve the first element of an array in PHP is by using the reset()
function. This function resets the array’s internal pointer to the first element and returns its value. Here’s how it works:
$array = ["apple", "banana", "cherry"];
$firstElement = reset($array);
echo $firstElement;
Output:
apple
In this code snippet, we again define an array with three fruit names. By calling reset($array)
, we not only reset the internal pointer of the array but also directly obtain the first element. This method is particularly beneficial when working with associative arrays or when you want to ensure that the pointer is set to the beginning of the array. It can be a cleaner approach, especially when dealing with larger arrays where you may want to manipulate the pointer as needed.
Using the current()
Function
The current()
function is another handy method to retrieve the first element of an array. This function returns the value of the element pointed to by the internal pointer of the array. Here’s an example:
$array = ["apple", "banana", "cherry"];
$firstElement = current($array);
echo $firstElement;
Output:
apple
In this example, we again have an array with three fruit names. By using current($array)
, we get the value of the element at the current pointer position, which, if the pointer has not been moved, will be the first element. This method is particularly useful when you’re iterating through an array and want to access the current element without manually managing the pointer. It provides a flexible way to work with arrays, especially in more complex scenarios where you might be traversing the array multiple times.
Conclusion
In conclusion, retrieving the first element of an array in PHP can be accomplished through various methods, each with its advantages. Whether you choose to access the element directly using its index, utilize the reset()
function, or leverage the current()
function, understanding these techniques will enhance your coding efficiency and versatility in PHP. As you continue to work with arrays, these methods will become second nature, allowing you to write cleaner and more effective code.
FAQ
-
How can I check if an array is empty before accessing its first element?
You can use theempty()
function in PHP to check if an array is empty. For example,if (!empty($array)) { ... }
will ensure that you only access the first element if the array contains items. -
What happens if I try to access the first element of an empty array?
If you attempt to access the first element of an empty array, PHP will throw a notice for an undefined index. It’s always good practice to check if the array is empty before trying to access its elements. -
Can I use these methods with associative arrays?
Yes, you can use these methods with associative arrays as well. Thereset()
andcurrent()
functions will return the first value of the array regardless of whether it is indexed or associative. -
Is there a performance difference between these methods?
In most cases, the performance difference is negligible for small arrays. However, using direct indexing is generally the fastest method for accessing an element if you know the index. Thereset()
andcurrent()
functions may have slight overhead due to pointer management. -
Can these methods be used in a loop?
Yes, you can use these methods in loops. For instance, you can iterate through an array and usecurrent()
to get the current element during each iteration. This can simplify the process of accessing array elements dynamically.