How to Echo or Print an Array in PHP
-
Use
foreach
Loop to Echo or Print an Array in PHP -
Use
print_r()
Function to Echo or Print an Array in PHP -
Use
var_dump()
Function to Echo or Print an Array in PHP
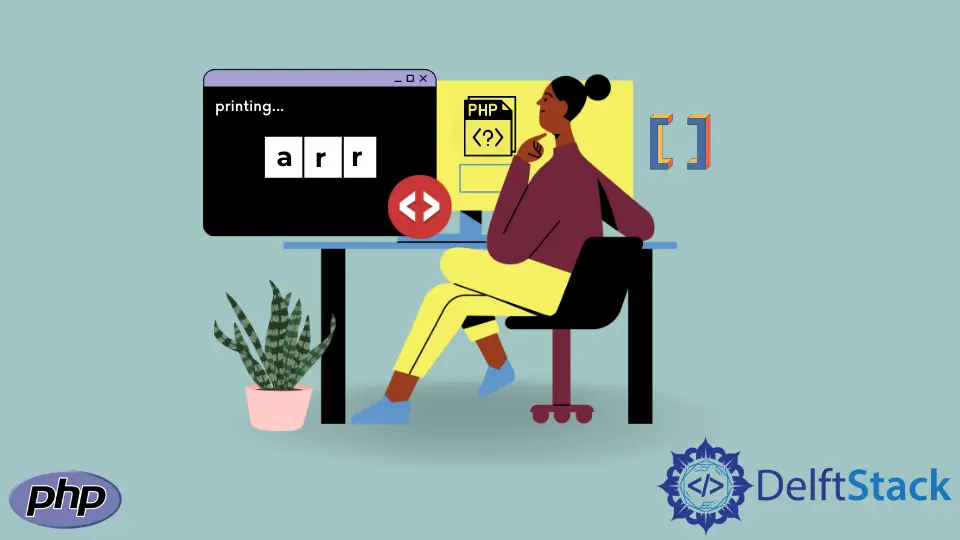
In this article, we will introduce methods to echo or print an array in PHP.
- Using
foreach
loop - Using
print_r()
function - Using
var_dump()
function
Use foreach
Loop to Echo or Print an Array in PHP
The foreach
loop iterates through each element of the array. It is the simplest method to fetch each element of the array. The correct syntax to use a foreach
loop is as follows
foreach( $arrayName as $variableName ) {
// action to perform
}
Each value of the array $arrayName
is assigned to the variable $variableName
. The pointer increments its value in each loop to iterate over the array.
<?php
//Declare the array
$flowers = array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
echo "The array is:\n";
//Prints the array
foreach($flowers as $flower){
echo $flower . "\n";
}
?>
Each value of the array $flowers
is assigned to the variable $flower
. The variable $flower
is then displayed using echo
. In this way, we have printed all the elements of the array.
Output:
The array is:
Rose
Lili
Jasmine
Hibiscus
Tulip
Sun Flower
Daffodil
Daisy
Use print_r()
Function to Echo or Print an Array in PHP
The built-in function print_r()
is used to print the value stored in a variable in PHP. We can also use it to print an array. It prints all the values of the array along with their index number. The correct syntax to use this function is as follows
print_r($variableName, $boolVariable)
It has two parameters. The first parameter $variableName
is a mandatory parameter as its value will be printed. The other parameter $boolVariable
is an optional variable, it is set False
by default. It stores the output of print_r()
function. If its value is True
, then the function will return the value which is supposed to print.
<?php
//Declare the array
$flowers = array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
//Print the structure of the array with data type
print_r($flowers);
?>
The array $flowers
is passed as a parameter to this function.
Output:
Array
(
[0] => Rose
[1] => Lili
[2] => Jasmine
[3] => Hibiscus
[4] => Tulip
[5] => Sun Flower
[6] => Daffodil
[7] => Daisy
)
Use var_dump()
Function to Echo or Print an Array in PHP
The var_dump()
function is used to print the details of any variable or expression. It prints the array with its index value, the data type of each element, and length of each element. It provides the structured information of the variable or array. The correct syntax to use this function is as follows
var_dump($variableName)
It only takes a single parameter $variableName
and returns the structured information of the variable.
<?php
//Declare the array
$flowers = array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
//Print the structure of the array with data type
var_dump($flowers);
?>
The array $flowers
is passed as the parameter to this function.
Output:
array(8) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(5) "Tulip"
[5]=>
string(10) "Sun Flower"
[6]=>
string(8) "Daffodil"
[7]=>
string(5) "Daisy"
}