How to Remove Empty Array Elements in PHP
-
Use
array_filter()
Function to Remove the Empty Array Elements in PHP -
Use
array_diff()
Function to Remove the Empty Array Elements in PHP -
Use
unset()
Function to Remove the Empty Array Elements in PHP
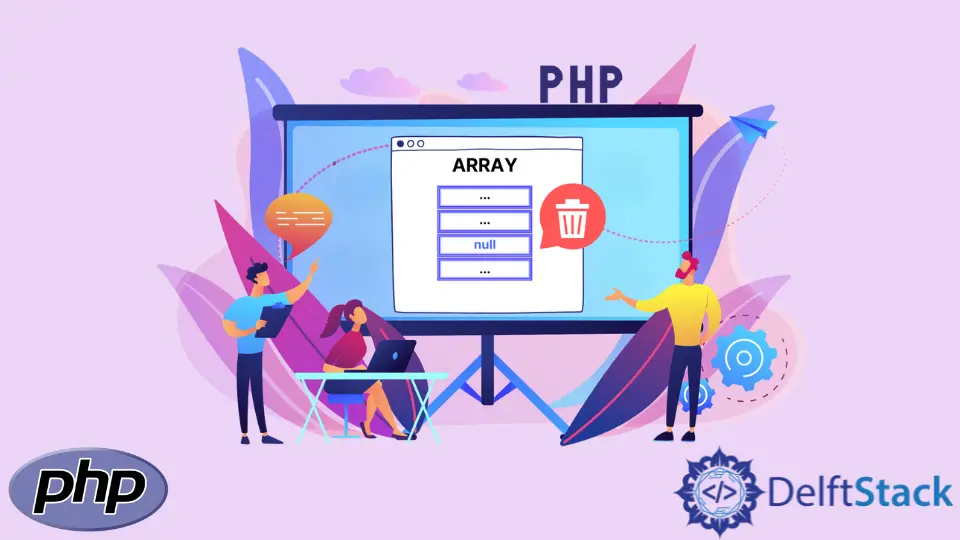
In this article, we will introduce methods to remove the empty array elements in PHP.
- Using
array_filter()
function - Using
array_diff()
function - Using
unset()
function
Use array_filter()
Function to Remove the Empty Array Elements in PHP
The built-in function array_filter()
removes all the empty elements, zeros, false and null values from an array. This function uses a callback function to filter the array values. If no callback function is specified, it removes the empty elements, zeros, false
and null
values.
The correct syntax to use this function is as follows
array_filter($arrayName, $callbackFunction, $callbackParameter)
Here the $arrayName
is the only mandatory parameter. The parameter $callbackFunction
is the callback function specified for the operation on the array. The parameter $callbackParameter
tells about the parameters passed to the callback function.
<?php
//Declare the array
$flowers = array(
"Rose",
"Lili",
"Jasmine",
0,
"Hibiscus",
"",
"Tulip",
null,
"Sun Flower",
"Daffodil",
"Daisy");
$flowers = array_filter($flowers);
echo "The array is:\n";
print_r($flowers);
?>
We have not specified any callback function, hence the returned array has no empty elements, zeros, and null values.
Output:
The array is:
Array
(
[0] => Rose
[1] => Lili
[3] => Jasmine
[4] => Hibiscus
[6] => Tulip
[8] => Sun Flower
[10] => Daffodil
[11] => Daisy
)
Use array_diff()
Function to Remove the Empty Array Elements in PHP
The built-in function array_diff()
is used to find the difference between two or more arrays
. It can be used to delete empty elements from an array. It does not re-index the array. The correct syntax to use this function is as follows.
array_diff($array1, $array2, $array3, ... , $arrayN);
It takes N number of the parameters (arrays). It compares the first array with all other arrays. It returns an array that contains all the elements of the first array that are not present in other arrays.
<?php
//Declare the array
$flowers = array(
"Rose",
"Lili",
"Jasmine",
0,
"Hibiscus",
"",
"Tulip",
null,
"Sun Flower",
"Daffodil",
"Daisy");
$flowers = array_diff($flowers, array("",0,null));
echo "The array is:\n";
print_r($flowers);
?>
Here, we have compared our array with an array that contains the empty string, 0, and null value. Then the returned array has no empty elements.
Output:
The array is:
Array
(
[0] => Rose
[1] => Lili
[3] => Jasmine
[4] => Hibiscus
[6] => Tulip
[8] => Sun Flower
[10] => Daffodil
[11] => Daisy
)
Use unset()
Function to Remove the Empty Array Elements in PHP
The unset()
function removes the value stored in a variable. We can use it to remove the empty elements from an array. The correct syntax to use this function is as follows.
unset($variableName);
It only accepts one parameter $variableName
. The $variableName
is a variable of which we want to remove the value.
<?php
//Declare the array
$flowers = array("Rose","Lili","","Jasmine","Hibiscus","Tulip","Sun Flower","","Daffodil","Daisy");
foreach($flowers as $key => $link)
{
if($link === '')
{
unset($flowers[$key]);
}
}
echo "The array is:\n";
print_r($flowers);
?>
Here, we have used a foreach
loop to find the empty array elements. If an array element is empty, its index along with array name is passed as a parameter to the unset()
function.
Output:
The array is:
Array
(
[0] => Rose
[1] => Lili
[3] => Jasmine
[4] => Hibiscus
[5] => Tulip
[6] => Sun Flower
[8] => Daffodil
[9] => Daisy
)