How to Remove All Spaces Out of a String in PHP
-
Use
str_replace()
Function to Strip All Spaces Out in PHP -
Use
preg_replace()
Function to Strip All Spaces Out in PHP
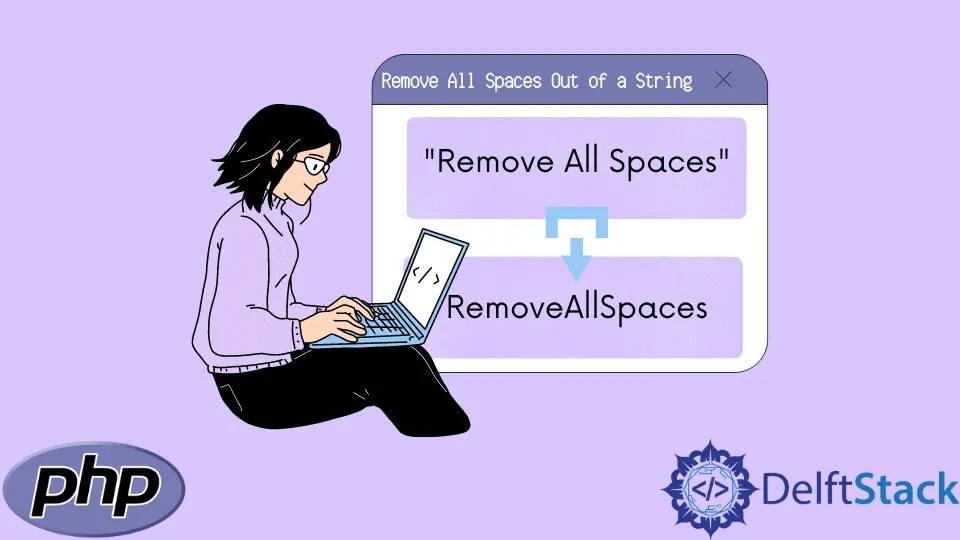
This article will introduce methods to strip all spaces out of a string
in PHP. Striping all spaces out means to remove all spaces from a given string
.
- Using
str_replace()
function - Using
preg_replace()
function
Use str_replace()
Function to Strip All Spaces Out in PHP
We use the built-in function str_replace()
to replace the substring from a string
or an array. The replacement string is passed as a parameter. The correct syntax to use this function is as follows.
str_replace($searchString, $replaceString, $originalString, $count);
The built-in function str_replace()
has four parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$searchString |
mandatory | It is the substring or an array that we want to find and replace. |
$replaceString |
mandatory | It is the string that we want to put in place of the $searchString . The function will check the occurrences of $searchString and replace it with $replaceString . It can be an array as well. |
$originalString |
mandatory | It is the original string from which we want to find a substring or a character to replace. |
$count |
optional | It specifies the total number of the replacements done on the $originalString . |
This function returns the final string
obtained after performing all the replacements on it.
The program below shows how we can use the str_replace()
function to remove all the spaces from a given string
.
<?php
$searchString = " ";
$replaceString = "";
$originalString = "This is a programming tutorial";
$outputString = str_replace($searchString, $replaceString, $originalString);
echo("The original string is: $originalString \n");
echo("The string without spaces is: $outputString");
?>
We have passed a space character as $searchString
and an empty string as $replaceString
. The output will be the string
without spaces.
Output:
The original string is: This is a programming tutorial
The string without spaces is: Thisisaprogrammingtutorial
Now, if we pass the $count
parameter, the function will tell us the number of replacements made on this string.
<?php
$searchString = " ";
$replaceString = "";
$originalString = "This is a programming tutorial";
$outputString = str_replace($searchString, $replaceString, $originalString, $count);
echo("The original string is: $originalString \n");
echo("The string without spaces is: $outputString \n");
echo("The number of replacement operations is: $count");
?>
Output:
The original string is: This is a programming tutorial
The string without spaces is: Thisisaprogrammingtutorial
The number of replacement operations is: 4
Use preg_replace()
Function to Strip All Spaces Out in PHP
In PHP, we can also use the preg_replace()
function to remove all spaces from a string
. This function will not just remove the space character, but it will also remove tabs if there are any in our string. The correct syntax to use this function is as follows:
preg_replace($regexPattern, $replacementVar, $original, $limit, $count)
The function preg_replace()
accepts five parameters. The detail of its parameters is as follows
Parameters | Description | |
---|---|---|
$regexPattern |
mandatory | It is the pattern we will search in the original string or array. |
$replacementVar |
mandatory | It is the string or array we use as a replacement for the searched value. |
$original |
mandatory | It is the string or an array from which we want to find value and replace it. |
$limit |
optional | This parameter limits the number of replacements. |
$count |
optional | This parameter tells about the number of total replacements made on our original string or array. |
We will use the pattern /\s+/
to find white spaces. The program that removes the spaces from the string
is as follows:
<?php
$searchString = " ";
$replaceString = "";
$originalString = "This is a programming tutorial";
$outputString = preg_replace('/\s+/', '', $originalString);
echo("The original string is: $originalString \n");
echo("The string without spaces is: $outputString \n");
?>
Output:
The original string is: This is a programming tutorial
The string without spaces is: Thisisaprogrammingtutorial
We know that the total number of replacements on this string
is 4. Now we will limit the number of replacements.
<?php
$searchString = " ";
$replaceString = "";
$limit = 2;
$originalString = "This is a programming tutorial";
$outputString = preg_replace('/\s+/', '', $originalString,$limit);
echo("The original string is: $originalString \n");
echo("The string without spaces is: $outputString \n");
?>
Output:
The original string is: This is a programming tutorial
The string without spaces is: Thisisa programming tutorial
Note that now there are only two replacements.