如何在 PHP 中刪除字串中的所有空格
Minahil Noor
2023年1月30日
PHP
PHP String
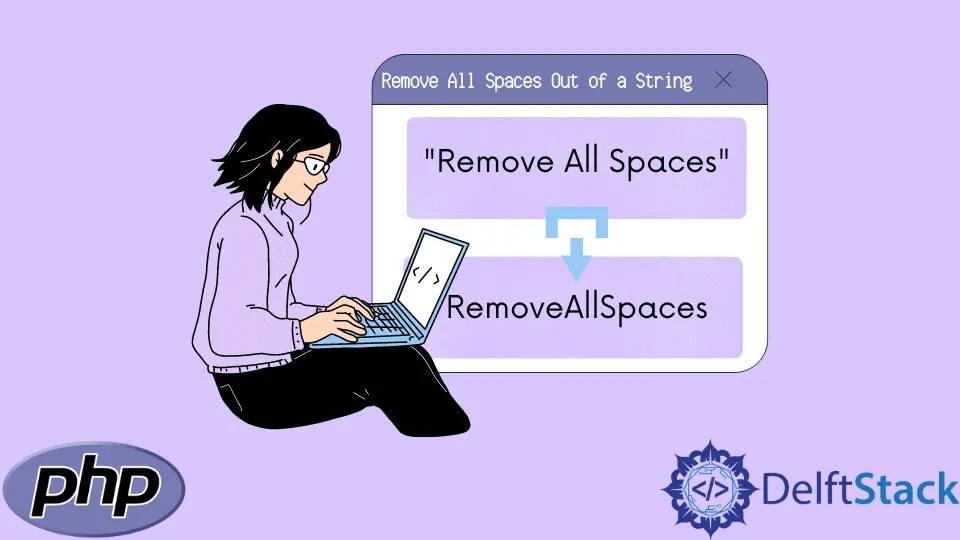
本文將介紹在 PHP 中從一個字串中去掉所有空格的方法。剝離所有空格是指從一個給定的字串中刪除所有空格。
- 使用
str_replace()
函式 - 使用
preg_replace()
函式
在 PHP 中使用 str_replace()
函式去掉所有空格
我們使用內建函式 str_replace()
來替換字串或陣列中的子字串。替換後的字串作為一個引數傳遞。使用該函式的正確語法如下。
str_replace($searchString, $replaceString, $originalString, $count);
內建函式 str_replace()
有四個引數。它的詳細引數如下
引數 | 說明 | |
---|---|---|
$searchString |
強制 | 它是我們要查詢和替換的子字串或陣列 |
$replaceString |
強制 | 它是我們要放在 $searchString 的位置的字串。函式將檢查 $searchString 的出現情況,並用 $replaceString 替換。該函式將檢查 $searchString 的出現情況,然後用 $replaceString 替換它。它也可以是一個 array 。 |
$originalString |
強制 | 它是原始的字串,我們想從中找到一個子字串或一個字元來替換。 |
$count |
可選 | 它告訴人們對 $originalString 進行替換的總次數 |
這個函式返回所有替換後得到的最終字串。
下面的程式顯示了我們如何使用 str_replace()
函式從給定的字串中刪除所有空格。
<?php
$searchString = " ";
$replaceString = "";
$originalString = "This is a programming tutorial";
$outputString = str_replace($searchString, $replaceString, $originalString);
echo("The original string is: $originalString \n");
echo("The string without spaces is: $outputString");
?>
我們已經傳遞了一個空格字元作為 $searchString
和一個空字串作為 $replaceString
。輸出將是沒有空格的字串。
輸出:
The original string is: This is a programming tutorial
The string without spaces is: Thisisaprogrammingtutorial
現在,如果我們傳入 $count
引數,函式將告訴我們這個字串的替換次數。
<?php
$searchString = " ";
$replaceString = "";
$originalString = "This is a programming tutorial";
$outputString = str_replace($searchString, $replaceString, $originalString, $count);
echo("The original string is: $originalString \n");
echo("The string without spaces is: $outputString \n");
echo("The number of replacement operations is: $count");
?>
輸出:
The original string is: This is a programming tutorial
The string without spaces is: Thisisaprogrammingtutorial
The number of replacement operations is: 4
在 PHP 中使用 preg_replace()
函式去除所有空格
在 PHP 中,我們也可以使用 preg_replace()
函式來刪除字串中的所有空格。這個函式不僅可以刪除空格字元,還可以刪除字串中的製表符。使用這個函式的正確語法如下。
preg_replace($regexPattern, $replacementVar, $original, $limit, $count)
函式 preg_replace()
接受五個引數. 它的詳細引數如下
引數 | 說明 | |
---|---|---|
$regexPattern |
強制 | 它是我們將在原始字串或陣列中搜尋的模式 |
$replacementVar |
強制 | 它是我們用來替換搜尋值的字串或陣列 |
$original |
強制 | 它是一個字串或陣列,我們要從其中找到值並替換它。 |
$limit |
可選 | 這個引數限制了替換的數量 |
$count |
可選 | 這個引數告訴了我們對原始字串或陣列進行替換的總次數 |
我們將使用模式 /\s+/
來查詢空格。從字串中刪除空格的程式如下。
<?php
$searchString = " ";
$replaceString = "";
$originalString = "This is a programming tutorial";
$outputString = preg_replace('/\s+/', '', $originalString);
echo("The original string is: $originalString \n");
echo("The string without spaces is: $outputString \n");
?>
輸出:
The original string is: This is a programming tutorial
The string without spaces is: Thisisaprogrammingtutorial
我們知道這個字串的總替換次數是 4,現在我們將限制替換次數。
<?php
$searchString = " ";
$replaceString = "";
$limit = 2;
$originalString = "This is a programming tutorial";
$outputString = preg_replace('/\s+/', '', $originalString,$limit);
echo("The original string is: $originalString \n");
echo("The string without spaces is: $outputString \n");
?>
輸出:
The original string is: This is a programming tutorial
The string without spaces is: Thisisa programming tutorial
注意,現在只有兩個替換。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe