How to Use Foreach Loop in Multidimensional Array in PHP
-
Use the
foreach
Loop to Access Elements of a Multidimensional Array in PHP -
Use the Nested
foreach
Loop to Access Elements of a Multidimensional Array in PHP
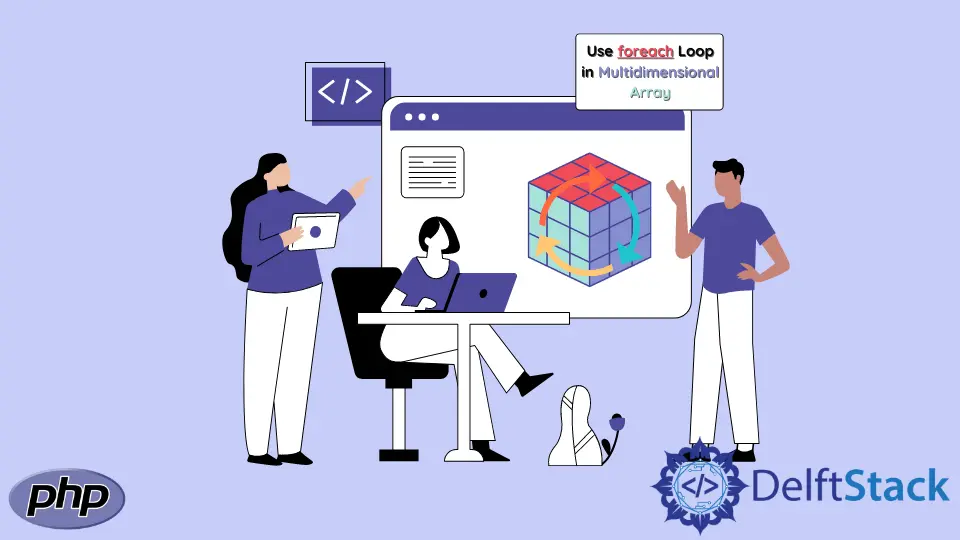
This tutorial will introduce the multidimensional array in PHP. We will also learn to use the foreach
loop in a multidimensional array.
Use the foreach
Loop to Access Elements of a Multidimensional Array in PHP
A multidimensional array contains one or more than one array inside an array.
An array can be two-dimensional three dimensional and can have more levels of dimensions. The complexity of an array increases with the increasing dimensions, and it is hard to manage the array.
We need two indices to access the two-dimensional array and three indices to access the three-dimensional array. In this article, we will discuss the two-dimensional array.
The following code example creates a two-dimensional array.
$bikes = array (
array("CRF300L",28,19.5),
array("CBR600RR",70,33),
array("KTM390 ",40,10)
);
Output:
Array
(
[0] => Array
(
[0] => CRF300L
[1] => 28
[2] => 19.5
)
[1] => Array
(
[0] => CBR600RR
[1] => 70
[2] => 33
)
[2] => Array
(
[0] => KTM390
[1] => 40
[2] => 10
)
)
The output above shows the contents of the $bikes
array.
We can see the array is numerically indexed. We can utilize the foreach
loop to access the array elements.
The foreach
loop is a special loop that works only on the array. As we know, that array contains a pair of keys and values; the foreach
loop is used to loop through the key/value pair of an array.
The syntax of the foreach
loop is shown below.
foreach($array as $element){
// statements
}
In the foreach
loop, the $element
variable will contain the value of the current array item for every iteration. The loop continues till the last element in the array.
In the case of the two-dimensional array, we can use the foreach
loop to access the first nested array in the first iteration and so on.
For example, write the foreach
loop where the array is $bikes
that we created above. Write the element as $bike
as shown in the example below.
Inside the loop, use echo
to display the content of the nested array using the indices on the $bike
variable. Use the indices as $bike[0]
, $bike[1]
and $bike[2]
.
The example is shown below.
foreach ($bikes as $bike){
echo $bike[0]."<br>";
echo $bike[1]."<br>";
echo $bike[2]."<br>";
echo "<br>";
}
Output:
CRF300L
28
19.5
CBR600RR
70
33
KTM390
40
10
In this way, we can use the foreach
loop to access the elements of a multidimensional array.
Use the Nested foreach
Loop to Access Elements of a Multidimensional Array in PHP
In this method, we will discuss how we can access the elements of a two-dimensional array using the nested foreach
loop. We can use the foreach
loop over the key/value pairs in an array.
The syntax for it is shown below.
foreach($array as $key=>value){
// statements
}
In this way, we can access the key and value of each element in an array using the foreach
loop.
We can create a nested foreach
loop to access all the key/value pairs in the two-dimensional array. We will use the array created above for the demonstration in this method.
For example, write the foreach
loop where the $bikes
variable is the array. Set the $number
variable as key and the $bike
variable as value.
Next, write another foreach
loop inside the just created loop. In the nested loop, write the $bike
variable as an array and set the $num
and $value
as key and value.
Inside the nested loops, print the variables $num
, $number
, and $value
as shown in the example below. Therefore, we can use the nested foreach
loop to access the elements of a multidimensional array in PHP.
Example Code:
foreach($bikes as $number => $bike)
{
foreach($bike as $num => $value)
{
print "Index ".$num." of ".$number." indexed array contains " .$value. "<br>";
}
}
Output:
Index 0 of 0 indexed array contains CRF300L
Index 1 of 0 indexed array contains 28
Index 2 of 0 indexed array contains 19.5
Index 0 of 1 indexed array contains CBR600RR
Index 1 of 1 indexed array contains 70
Index 2 of 1 indexed array contains 33
Index 0 of 2 indexed array contains KTM390
Index 1 of 2 indexed array contains 40
Index 2 of 2 indexed array contains 10
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedInRelated Article - PHP Array
- How to Determine the First and Last Iteration in a Foreach Loop in PHP
- How to Convert an Array to a String in PHP
- How to Get the First Element of an Array in PHP
- How to Echo or Print an Array in PHP
- How to Delete an Element From an Array in PHP
- How to Remove Empty Array Elements in PHP