Performance and Readability of for Loop and foreach Loop in PHP
-
the
for
Loop andforeach
Loop With Range -
the
for
Loop With a Postfix and Prefix Increment -
the
for
Loop With thecount
Function -
the
foreach
Without a Key andforeach
With a Key -
the
for
Loop Withcount
Function andforeach
With a Key and Value -
the
for
Loop Withcount
andforeach
Without a Key -
the
for
Loop Withcount
andforeach
With Range -
Readability of
for
Loop andforeach
Loop
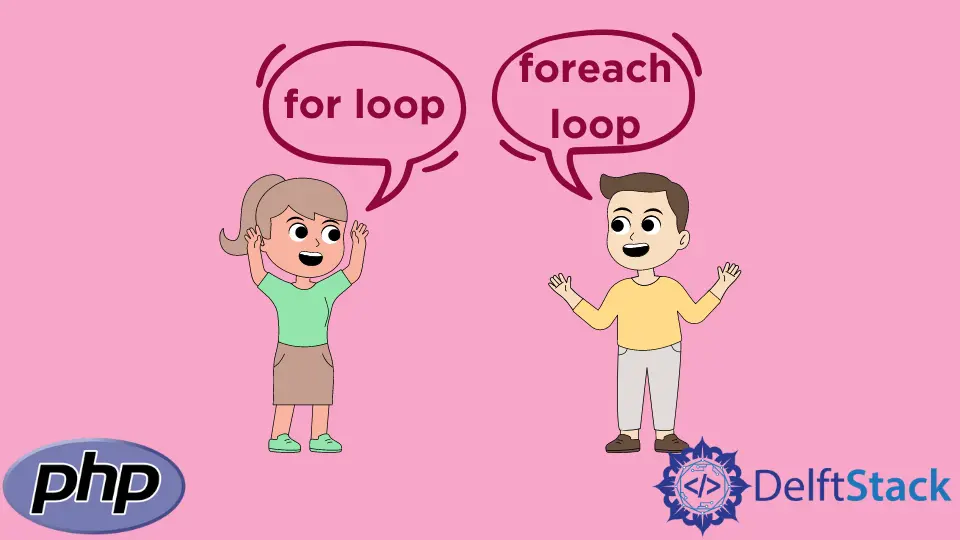
This article does a comparison between the for
loop and foreach
in PHP. The comparison aims to determine which loop has a faster execution speed and better readability.
the for
Loop and foreach
Loop With Range
We compared the for
loop and foreach
loop with range
in a hundred thousand iterations. The test results show that the for
loop executes faster than the foreach
loop.
The reason is that the for
loop does its comparison by increasing integer values. In comparison, foreach
with range
has to create an array before extracting each element and checking for the end of the array.
<?php
$time_one = microtime(true);
for ($i=0; $i < 100000; $i++) {
# code...
}
echo 'For loop: ' . (microtime(true) - $time_one) . ' s', PHP_EOL;
echo "<br />";
$time_two = microtime(true);
foreach (range(0, 100000) as $i) {
# code...
}
echo 'Foreach + range loop: ' . (microtime(true) - $time_two) . ' s', PHP_EOL;
?>
Output:
For loop: 0.001291036605835 s
Foreach + range loop: 0.00661301612854 s
the for
Loop With a Postfix and Prefix Increment
If the time difference is quite critical, you should know that the for
loop with a prefix increment executes faster than a for
loop with a postfix increment.
That is because postfix keeps a copy of the previous value before it increments it by one while prefix increment adds one without keeping a copy of the previous value.
Example:
<?php
$time_one = microtime(true);
for ($i=0; $i < 100000; ++$i) {
# code...
}
echo 'For loop with prefix $i: ' . (microtime(true) - $time_one) . ' s', PHP_EOL;
echo "<br />";
$time_two = microtime(true);
for ($i=0; $i < 100000; $i++) {
# code...
}
echo 'For loop with postfix $i: ' . (microtime(true) - $time_two) . ' s', PHP_EOL;
?>
Output:
For loop with prefix $i: 0.0012187957763672 s
For loop with postfix $i: 0.0031270980834961 s
the for
Loop With the count
Function
The for
loop with the count
function executes faster than without.
We run two tests in the code block below, the first test used a for
loop with a count
function, and the second test used the number of elements in the array without the count
function.
Example:
<?php
$myArray = [1, 4, 6, 8, 11, 14, 16, 18, 21, 24];
$time_one = microtime(true);
for ($i=0; $i < count($myArray); $i++) {
# code...
}
echo 'For loop with count function: ' . (microtime(true) - $time_one) . ' s', PHP_EOL;
echo "<br />";
$time_two = microtime(true);
for ($i=0; $i < 10; $i++) {
# code...
}
echo 'For loop without count function: ' . (microtime(true) - $time_two) . ' s', PHP_EOL;
?>
Output:
For loop with count function: 6.9141387939453E-6 s
For loop without count function: 9.5367431640625E-7 s
the foreach
Without a Key and foreach
With a Key
A foreach
loop without a key executes faster when compared with a foreach
loop with a key and value.
The snippet below has an array of ten elements where we execute a foreach
loop on the array. The first execution does not use a key, while the second execution uses a key and value.
Example:
<?php
$myArray = [1, 4, 6, 8, 11, 14, 16, 18, 21, 24];
$time_one = microtime(true);
foreach ($myArray as $value) {
# code...
}
echo 'Foreach loop without key: ' . (microtime(true) - $time_one) . ' s', PHP_EOL;
echo "<br />";
$t01 = microtime(true);
foreach ($myArray as $key => $value) {
# code...
}
echo 'Foreach loop with key and value: ' . (microtime(true) - $t01) . ' s', PHP_EOL;
?>
Output:
Foreach loop without key: 0.00055599212646484 s
Foreach loop with key and value: 9.5367431640625E-7 s
the for
Loop With count
Function and foreach
With a Key and Value
A test on an array with ten elements between the foreach
(with key and value) and for
with the count
function shows that a foreach
with key and a value executes faster than a for
loop with the count function.
Example:
<?php
$myArray = [1, 4, 6, 8, 11, 14, 16, 18, 21, 24];
$time_one = microtime(true);
for ($i=0; $i < count($myArray); $i++) {
# code...
}
echo 'For loop with count: ' . (microtime(true) - $time_one) . ' s', PHP_EOL;
echo "<br />";
$time_two = microtime(true);
foreach ($myArray as $key => $value) {
# code...
}
echo 'Foreach loop with key and value: ' . (microtime(true) - $time_two) . ' s', PHP_EOL;
?>
Output:
For loop with count: 8.1062316894531E-6 s
Foreach loop with key and value: 2.1457672119141E-6 s
the for
Loop With count
and foreach
Without a Key
Both for
loop with a count
function and foreach
without a key execute at the same speed.
<?php
$myArray = [1, 4, 6, 8, 11, 14, 16, 18, 21, 24];
$time_one = microtime(true);
for ($i=0; $i < count($myArray); $i++) {
# code...
}
echo 'For loop with count: ' . (microtime(true) - $time_one) . ' s', PHP_EOL;
echo "<br />";
$time_two = microtime(true);
foreach ($myArray as $value) {
# code...
}
echo 'Foreach loop without key: ' . (microtime(true) - $time_two) . ' s', PHP_EOL;
?>
Output:
For loop with count: 9.5367431640625E-7 s
Foreach loop without key: 9.5367431640625E-7 s
the for
Loop With count
and foreach
With Range
The for
loop with count executes faster than the foreach
loop with range.
<?php
$myArray = [1, 4, 6, 8, 11, 14, 16, 18, 21, 24];
$time_one = microtime(true);
for ($i=0; $i < count($myArray); $i++) {
# code...
}
echo 'For loop with count: ' . (microtime(true) - $time_one) . ' s', PHP_EOL;
echo "<br />";
$time_two = microtime(true);
foreach (range(0, 10) as $i) {
# code...
}
echo 'Foreach loop with range: ' . (microtime(true) - $time_two) . ' s', PHP_EOL;
?>
Output:
For loop with count: 1.0013580322266E-5 s
Foreach loop with range: 3.0994415283203E-6 s
Take note when you run the code examples in your web browser, you’ll get different time results. We performed all the code examples above with PHP 8.1.2 on Windows 10.
Readability of for
Loop and foreach
Loop
In terms of readability, you’ll find that the for
loop has better readability than the foreach
loop. That is because, for the for
loop, you’ll know that you are doing a comparison of a counter less than the bigger number.
When using foreach
with range, you’ll have to deduce the purpose of the range
function within the foreach
loop.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn