How to Loop Through Associative Array in PHP
-
Use the
foreach
Loop to Loop Through an Associative Array and Obtain the Keys in PHP -
Use the
array_keys()
Function With theforeach
Loop to Obtain Keys From an Associative Array in PHP -
Use the
array_keys()
Function With thefor
Loop to Obtain Keys From an Associative Array in PHP
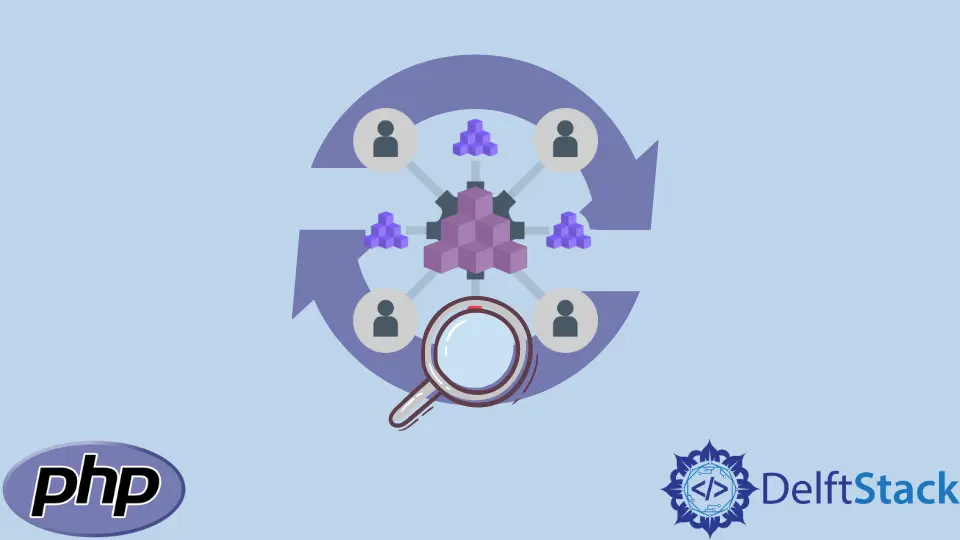
This article will introduce a few methods to loop through an associative array in PHP. We will also learn how to obtain the keys from the array.
Use the foreach
Loop to Loop Through an Associative Array and Obtain the Keys in PHP
There are many looping statements in PHP and other popular programming languages like for
, while
, do...while
, foreach
, etc. These different loops are used according to the nature of the problem.
We can use the foreach
loop to loop through an array. The foreach
loop is suitable for iterating through each element of an array.
The syntax of the foreach
loop is shown below.
foreach($array as $value){
statements
}
Here, $array
is the array iterated, and $value
is the array’s item in each iteration.
We can also use the foreach
loop in an associative array to loop through the key and value of the array. An associative array is a type of array that contains a key and value pair for each item of the array.
Using the foreach
loop, we can obtain the key and value of the array items. The foreach
loop syntax for an associative array is shown below.
foreach($array as $key => $value){
statements
}
Here, $key
is the index of the array item, and $value
is the item.
The following instructions and examples demonstrate how to loop through an associative array and obtain each key from the array.
-
Create an associative array
$age
with the name and age of people as key and value. -
Next, use the
foreach
loop where$age
is the array and$key
and$value
are the key-value pairs asforeach ($age as $key => $value)
. -
Use the
echo
function inside the loop to print the$key
.
Example Code:
<?php
$age = array("Paul"=>"35", "Brandon"=>"37", "Jack"=>"43");
foreach ($age as $key => $value) {
echo $key."<br>";
}
?>
Output:
Paul
Brandon
Jack
In the example above, we have used the following associative array.
Array ( [Paul] => 35 [Brandon] => 37 [Jack] => 43 )
We printed the keys of the array, which are the names of the people. They can be seen in the output section above.
Use the array_keys()
Function With the foreach
Loop to Obtain Keys From an Associative Array in PHP
PHP provides a function array_keys()
that picks the keys from an array supplied as an argument. Then, we can use the foreach
loop to loop through the keys and list them all.
The syntax of the array_keys()
function is shown below.
array_key($array, $search_value)
This function returns an array of keys. The argument $array
is the array from which the key is to be extracted.
The argument search_value
is an optional argument through which we can extract the key specifying the specific value.
For example,
-
Consider an associative array
$nationality
with the following key-value pairs.Array ( [Paul] => England [Brandon] => New Zealand [Jack] => Ireland )
-
Create a variable
$names
and assign thearray_keys()
function to it with the$nationality
as the argument of the function. -
Next, use the
foreach
loop to loop through the$names
array. -
Inside the loop, print each item of the
$names
array with the$name
value.
Example Code:
<?php
$nationality = array("Paul"=>"England", "Brandon"=>"New Zealand", "Jack"=>"Ireland");
$names =array_keys($nationality);
foreach ($names as $name) {
echo $name."<br>";
}
?>
In the example above, the output of the $names
array looks like this.
Array ( [0] => Paul [1] => Brandon [2] => Jack ) Paul
Thus, we obtained an array of the $nationality
keys. We looped through this array using the foreach
loop to extract each item.
The output is shown below.
Output:
Paul
Brandon
Jack
In this way, we can obtain the keys of an array using the array_keys()
function in PHP.
Use the array_keys()
Function With the for
Loop to Obtain Keys From an Associative Array in PHP
The third method imitates the second method using the for
loop. The difference between the for
and foreach
loop is that we can manually specify the condition and number of iterations in the for
loop.
We can count the number of elements of the array with the count()
function to determine the number of iterations. The array_keys()
function is the same as in the second method.
Here, we will consider the same associative array used in the above method.
-
After applying the
array_keys()
function to the array, create afor
loop. -
Use the
count()
function to count the length of the$names
array. -
Use the length to specify the ending condition of the
for
loop. -
Start the loop from the counter
0
. -
Initialize it with the
$i
variable. -
Increment the variable
$i
with1
in thefor
loop. -
Inside the loop, use the index
$i
in the$names
array to print each item.
Example Code:
<?php
$nationality = array("Paul"=>"England", "Brandon"=>"New Zealand", "Jack"=>"Ireland");
$names =array_keys($nationality);
for($i=0; $i<count($names); $i++) {
echo $names[$i]."<br>";
}
?>
Output:
Paul
Brandon
Jack
This way, we can use the for
loop and array_keys()
to obtain keys from an associative array in PHP.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedInRelated Article - PHP Array
- How to Determine the First and Last Iteration in a Foreach Loop in PHP
- How to Convert an Array to a String in PHP
- How to Get the First Element of an Array in PHP
- How to Echo or Print an Array in PHP
- How to Delete an Element From an Array in PHP
- How to Remove Empty Array Elements in PHP