How to Replace Object in an Array in JavaScript
- Replace Object in an Array Using the Index in JavaScript
- Replace Object in an Array Using the Splice Method in JavaScript
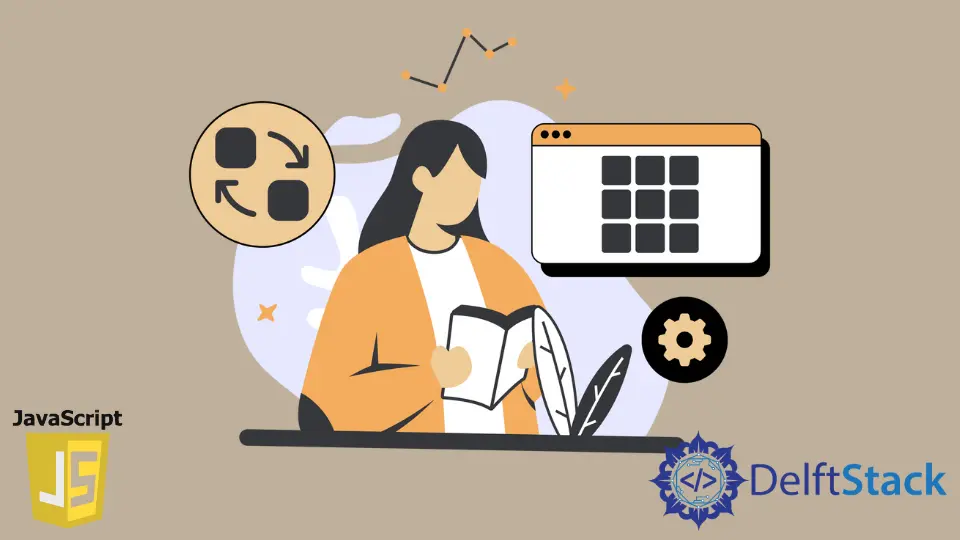
This article will tackle how to replace the different objects stored in the array in JavaScript easily because JavaScript is a dynamic language.
In JavaScript, the objects and the type in an array are dynamic or can change. We can store different object types in an array.
Replace Object in an Array Using the Index in JavaScript
By knowing the object’s index, we can easily replace any object in an array. It is one of the most common ways to replace the objects in the array in JavaScript.
Let’s understand this using a simple example.
In the code below, we created an array named selectedColors
, and we stored five objects with a similar type.
Example code:
let selectedColors = ['Red', 'Blue', 'Orange', 'Black', 'Pink'];
console.log(selectedColors);
Output:
[ 'Red', 'Blue', 'Orange', 'Black', 'Pink' ]
Suppose we want to replace the first two objects with different colors names in the array. We can do that using the index of these objects.
At index 0, we have Red, and at index 1, we have Blue. We can replace these two colors using selectedColors[]
, give the index number of the object we want to replace, and assign a new color.
Example code:
let selectedColors = ['Red', 'Blue', 'Orange', 'Black', 'Pink'];
selectedColors[0] = 'Green';
selectedColors[1] = 'White';
console.log(selectedColors);
Output:
[ 'Green', 'White', 'Orange', 'Black', 'Pink' ]
As we can see in the code, we used selectedColors[0] = 'Green';
which means we assign the Green at index 0, and again we use the same procedure for selectedColors[1] = 'White';
where we give White at index 1.
After running the code, Red and Blue are replaced by Green and White, respectively. In the output, we have Green now at index 0 and White at index 1.
Replace Object in an Array Using the Splice Method in JavaScript
Another way to replace the object in an array in JavaScript is to use the splice method. The splice method allows us to update the array’s objects by removing or replacing existing elements in an array at the desired index.
If we want to replace an object in an array, we will need its index first.
Let’s understand how this method works by the following example.
Example code:
let monthNames = ['January', 'March', 'April', 'June'];
monthNames.splice(1, 0, 'February');
console.log('February is added in the list ', monthNames);
monthNames.splice(4, 1, 'May');
console.log('June is replaced by May ', monthNames);
Output:
February is added in the list [ 'January', 'February', 'March', 'April', 'June' ]
June is replaced by May [ 'January', 'February', 'March', 'April', 'May' ]
First, we created an array named monthNames
and stored the month’s names.
After that, we applied the splice() method
on the array by using monthNames.splice(1, 0, "February");
. This resulted in the new element February
at index 1 without removing any element in the array.
The 1, 0, "February"
in the mothNames.splice()
means adding a new element at index 1, and 0 means we are not removing any element in the array.
We applied another splice()
method on the resulted array after the first splice()
using monthNames.splice(4, 1, "May");
to replace the element June
which is at index 4 by the new element May
.
In monthNames.splice(4, 1, "May");
, the 4, 1
means that we want to replace the element at index 4 with May
, and 1 means removing one element from the index 4.
In the output, the element June
is replaced by the new element May
at index 4. This is how we can use the splice()
method to replace any object in an array in JavaScript.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript