Array vs Object Declaration in JavaScript
- Understanding JavaScript Arrays
- Understanding JavaScript Objects
- Key Differences Between Arrays and Objects
- When to Use Arrays vs Objects
- Conclusion
- FAQ
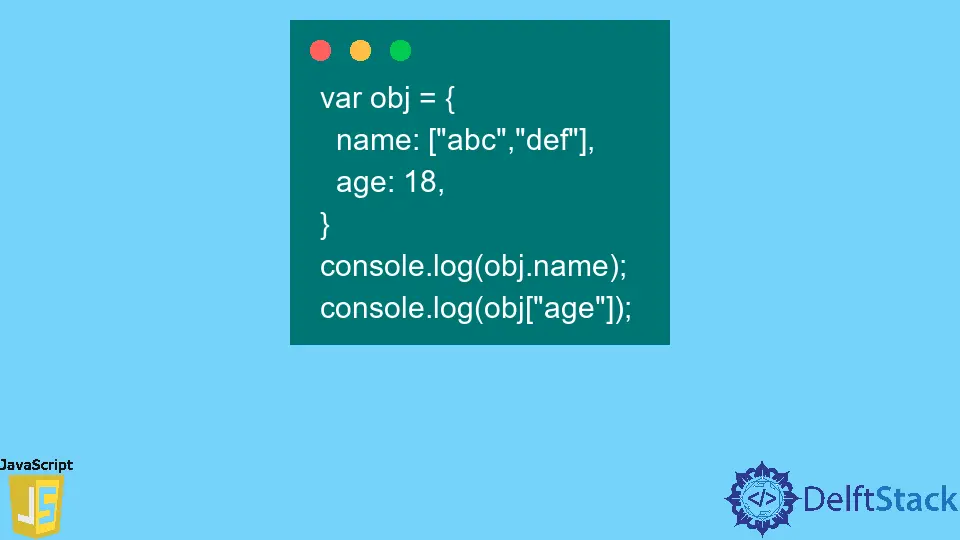
JavaScript is a versatile programming language that allows developers to work with complex data structures. Among these, arrays and objects are two of the most commonly used. Understanding the differences between array and object declarations in JavaScript is crucial for writing efficient and effective code.
This tutorial will break down the distinctions, usage scenarios, and syntax for both arrays and objects, helping you grasp their unique characteristics. Whether you’re a beginner or an experienced developer, knowing when to use an array or an object can significantly enhance your coding practices. Let’s dive into the details!
Understanding JavaScript Arrays
Arrays in JavaScript are ordered collections that can hold multiple values. They are ideal for storing lists of items and allow for easy access to each item based on its index. Arrays are zero-indexed, meaning the first element is at index 0. You can declare an array using square brackets.
Array Declaration Example
let fruits = ["apple", "banana", "cherry"];
Output:
["apple", "banana", "cherry"]
In this example, we declare an array named fruits
that contains three string elements. You can access each element using its index, like fruits[0]
for “apple”. Arrays also come with built-in methods such as push()
, pop()
, and map()
that allow you to manipulate the data easily. They are particularly useful when you need to perform operations on a list of items, such as filtering or transforming data.
Understanding JavaScript Objects
Unlike arrays, objects in JavaScript are unordered collections of key-value pairs. They are perfect for representing complex data structures where each value is associated with a unique key. You can declare an object using curly braces.
Object Declaration Example
let person = {
name: "John",
age: 30,
city: "New York"
};
Output:
{name: "John", age: 30, city: "New York"}
In this example, we declare an object called person
with three properties: name
, age
, and city
. You can access these properties using dot notation or bracket notation, like person.name
or person["age"]
. Objects are ideal for representing real-world entities with multiple attributes, such as a user profile or a product description.
Key Differences Between Arrays and Objects
While both arrays and objects are fundamental data structures in JavaScript, they serve different purposes. Here are some key differences:
- Order: Arrays maintain the order of elements based on their index, while objects do not guarantee any specific order for their properties.
- Access Method: You access array elements using numeric indices, while object properties are accessed using strings as keys.
- Use Cases: Arrays are best for lists of items, while objects are suitable for representing entities with multiple attributes.
Understanding these differences will help you choose the right data structure based on your specific needs.
When to Use Arrays vs Objects
Choosing between arrays and objects often depends on the data you are working with. If you need to store a list of items where the order matters, arrays are your best bet. For example, if you are managing a list of user names or product IDs, an array is the way to go.
On the other hand, if you need to represent an entity with various attributes, like a user profile containing a name, age, and address, an object is more appropriate. Objects allow you to group related data together, making it easier to manage complex structures.
Conclusion
In summary, understanding the differences between array and object declarations in JavaScript is essential for effective coding. Arrays are ideal for ordered lists, while objects are perfect for representing complex data structures with key-value pairs. By mastering these two data structures, you’ll enhance your JavaScript programming skills and be better equipped to tackle various coding challenges. Whether you’re building a simple application or a complex web project, knowing when to use arrays and objects will make a significant difference in your development process.
FAQ
-
What is the main difference between arrays and objects in JavaScript?
Arrays are ordered collections accessed by index, while objects are unordered collections accessed by keys. -
Can an object contain an array?
Yes, an object can contain an array as one of its properties. -
How do you loop through an array in JavaScript?
You can use afor
loop,forEach()
, or other array methods likemap()
to loop through an array. -
Can arrays hold different data types?
Yes, JavaScript arrays can hold elements of different data types, including strings, numbers, and even other objects. -
How do you check if a variable is an array in JavaScript?
You can useArray.isArray(variable)
to check if a variable is an array.
Related Article - JavaScript Object
- How to Search Objects From an Array in JavaScript
- How to Get the Object's Value by a Reference to the Key
- How to Find Object in Array by Property Value in JavaScript
- How to Print Objects in JavaScript
- How to Destroy Object in JavaScript
- Nested Objects in JavaScriptn
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript