How to Sort a String Array Alphabetically in Java
- Sort a String Array Without Using Built-In Methods in Java
-
Sort a String Array Using the
stringArraySort()
Method in Java -
Sort a String Array Using the
compareTo()
Method in Java -
Sort a String Array Using the
Arrays.sort()
Method in Java - Sort a String Array in Descending Order in Java
- Sort a String Array by Ignoring the Case in Java
- Sort a String Array Alphabetically in Java
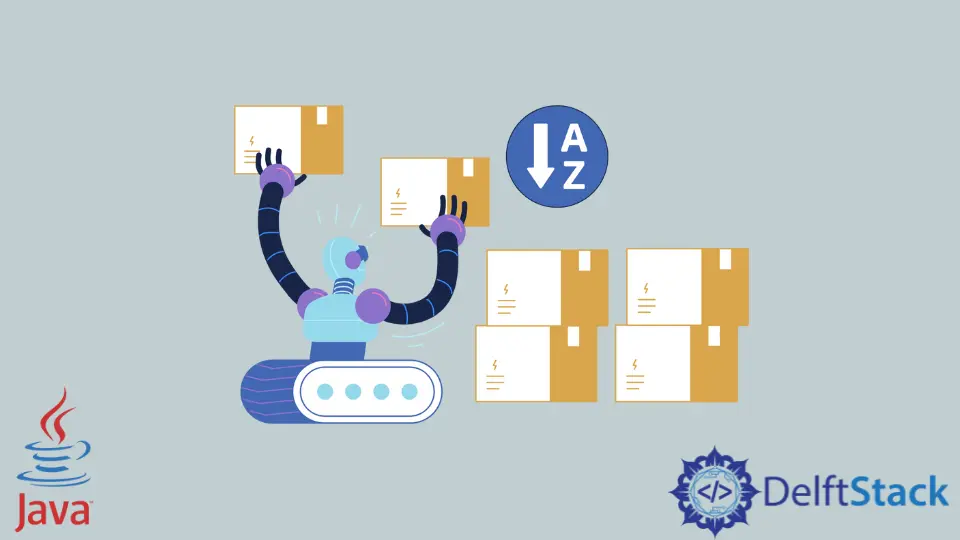
This tutorial introduces how to sort string arrays alphabetically in Java. We’ve also listed some example codes to help you understand the topic.
Sorting is arranging data in a particular order. We can sort integers in ascending or descending order, or we can sort English characters or words in lexicographical order.
Lexicographical order is the order in which words would occur if they were present in an English word dictionary. In this guide, we will teach you ways on how to sort a string array in Java.
We will be comparing strings in this article with words like smaller or greater. One string is smaller than the other if it occurs first according to the lexicographical order.
Additionally, in the lexicographical order, all uppercase letters precede the lowercase ones; this means that a word starting with Z
will be present before a word starting with a
in a sorted array.
Sort a String Array Without Using Built-In Methods in Java
We will first try to implement our own sorting method in Java. We will create a method called compareStrings()
, which compares two strings and tells us which one is smaller or larger.
Next, we will compare each string with all of the other strings present in the array by using our compareStrings()
method and place that string in its correct position. The algorithm for the two methods is given below.
- The input for this method will be two strings, and it will return an integer value. The output of this method is as follows.
- If
string1
>string2
: return positive integer - If
string1
<string2
: return negative integer - If
string1
==string2
: return0
- If
- We will loop through each character of both strings simultaneously and compare their Unicode values. We use the built-in
charAt()
method to get the Unicode values. These values allow us to compare characters as if they were normal integers. - If the Unicode values are the same for both the characters, we will move on to the next character of each string.
- If the Unicode values differ, then we return the difference of the values.
- The loop ends when we run out of characters from either string.
- If the two strings have different lengths outside the loop, then we return this difference. We do this because all characters of the smaller string may be present at the beginning of the longer one. For example,
app
andapplication
. In this case, the longer string will come after the smaller one. - If the strings are of the same length, we will simply return
0
, indicating that the two strings are the same.
Sort a String Array Using the stringArraySort()
Method in Java
This method will take an array of strings as an input and returns the sorted array. Here, we will have two loops, one nested in the other. They are used to compare each element of the array with all of the remaining elements.
We compare them using our compareString()
method for each pair of elements inside the loop. If the first string is greater compared to the other, we swap their positions.
The complete code is shown below.
public class Main {
public static int compareStrings(String word1, String word2) {
for (int i = 0; i < Math.min(word1.length(), word2.length()); i++) {
if ((int) word1.charAt(i) != (int) word2.charAt(i)) // comparing unicode values
return (int) word1.charAt(i) - (int) word2.charAt(i);
}
if (word1.length()
!= word2.length()) // smaller word is occurs at the beginning of the larger word
return word1.length() - word2.length();
else
return 0;
}
public static String[] stringArraySort(String[] words) {
for (int i = 0; i < words.length - 1; i++) {
for (int j = i + 1; j < words.length; j++) {
if (compareStrings(words[i], words[j]) > 0) // words[i] is greater than words[j]
{
String temp = words[i];
words[i] = words[j];
words[j] = temp;
}
}
}
return words;
}
public static void main(String[] args) {
String[] arrToSort = {"apple", "oranges", "bananas", "Strawberry", "Blueberry"};
String[] sortedArr = stringArraySort(arrToSort);
for (int i = 0; i < sortedArr.length; i++) System.out.print(sortedArr[i] + " ");
}
}
Output:
Blueberry Strawberry apple bananas oranges
Sort a String Array Using the compareTo()
Method in Java
In the code above, we wrote a method that will compare two strings and return an integer value to denote which string will come first if they were present in a dictionary.
Java provides us with a built-in method called compareTo()
, which can do this work for us. The syntax of this method is shown below.
string1.compareTo(string2)
The output of this method is similar to the compareStrings()
method that we defined earlier.
- If
string1
>string2
: return positive integer - If
string1
<string2
: return negative integer - If
string1
==string2
: return 0
The following code demonstrates how the compareTo()
method works in Java.
public class Main {
public static void main(String[] args) {
System.out.println("apple".compareTo("orange")); // apple < orange
System.out.println("AAA".compareTo("aaa")); // AAA < aaa
System.out.println("ZZZ".compareTo("aaa")); // ZZZ < aaa
System.out.println("zzz".compareTo("aaa")); // zzz > aaa
System.out.println("strawberry".compareTo("strawberry")); // strawberry == strawberry
}
}
Output:
-14
-32
-7
25
0
We can use the compareTo()
method to sort arrays. This program will be very similar to the previous one above. The only difference is that we will use the compareTo()
method instead of the compareStrings()
method that we defined earlier.
public class Main {
public static String[] stringArraySort(String[] words) {
for (int i = 0; i < words.length - 1; i++) {
for (int j = i + 1; j < words.length; j++) {
if (words[i].compareTo(words[j]) > 0) // words[i] is greater than words[j]
{
String temp = words[i];
words[i] = words[j];
words[j] = temp;
}
}
}
return words;
}
public static void main(String[] args) {
String[] arrToSort = {"apple", "oranges", "bananas", "Strawberry", "Blueberry"};
String[] sortedArr = stringArraySort(arrToSort);
for (int i = 0; i < sortedArr.length; i++) System.out.print(sortedArr[i] + " ");
}
}
Output:
Blueberry Strawberry apple bananas oranges
Sort a String Array Using the Arrays.sort()
Method in Java
There is an even simpler way of sorting arrays. We can use the sort()
method of the Arrays
class to do this. The syntax of this method is shown below.
Arrays.sort(stringArray)
By default, it will sort the array in the natural order (lexicographically). However, we can change this order by specifying different comparators.
A comparator is a method used to compare two objects and tell us which one is smaller or greater than the other one. The sort()
method uses the Quicksort
Algorithm and has a time complexity of O(n*log(n))
.
The following code shows the default behavior of the sort()
method.
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
String[] arrToSort = {"apple", "oranges", "bananas", "Strawberry", "Blueberry"};
Arrays.sort(arrToSort);
for (int i = 0; i < arrToSort.length; i++) {
System.out.print(arrToSort[i] + " ");
}
}
}
Output:
Blueberry Strawberry apple bananas oranges
Sort a String Array in Descending Order in Java
To sort the array in the reverse natural order or the descending order, we can use the reverseOrder()
comparator as shown below.
import java.util.Arrays;
import java.util.Collections;
public class Main {
public static void main(String[] args) {
String[] arrToSort = {"apple", "oranges", "bananas", "Strawberry", "Blueberry"};
Arrays.sort(arrToSort, Collections.reverseOrder());
for (int i = 0; i < arrToSort.length; i++) {
System.out.print(arrToSort[i] + " ");
}
}
}
Output:
oranges bananas apple Strawberry Blueberry
Sort a String Array by Ignoring the Case in Java
To set a string array in alphabetical order but ignore the case of the character, use this code example below.
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
String[] arrToSort = {"apple", "oranges", "bananas", "Strawberry", "Blueberry"};
Arrays.sort(arrToSort, String.CASE_INSENSITIVE_ORDER); // case insensitive sort
for (int i = 0; i < arrToSort.length; i++) {
System.out.print(arrToSort[i] + " ");
}
}
}
Output:
apple bananas Blueberry oranges Strawberry
Sort a String Array Alphabetically in Java
Sorting is arranging elements in a particular order. We can sort a string array in alphabetical or lexicographical order. Java has a built-in compareTo()
method, which can be used to compare two strings and tell which one is greater than the other. We can use this method to write our sorting algorithm.
Java also has the Arrays.sort()
method, which can sort a string array. We can use different comparators for our sort()
method to set the array order in different ways.
Related Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java