How to Shuffle Deck of Cards in Java
Rashmi Patidar
Feb 02, 2024
Java
Java ArrayList
Java Loop
Java Array
-
Shuffle the
ArrayList
Using the Traditional Loop in Java -
Shuffle the
ArrayList
Using the Collectionsshuffle
Function in Java
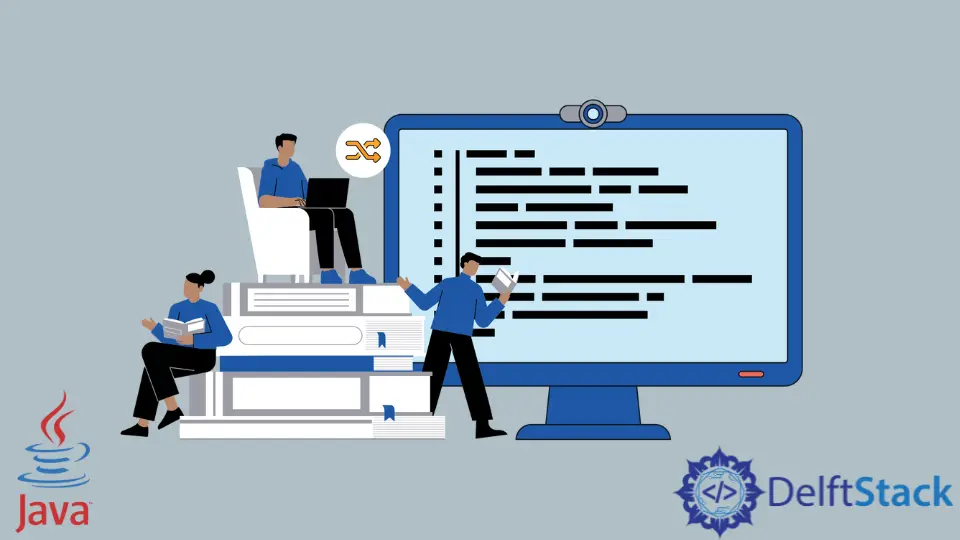
The word shuffle literally means to arrange the elements, objects, or cards in random or undefined order. The objects list that does not follow a defined pattern is considered shuffled.
Similarly, in Java, various methods allow a user to shuffle elements. Below is the code block to demonstrate this process.
Shuffle the ArrayList
Using the Traditional Loop in Java
import java.util.ArrayList;
public class Main {
private static final int SIZE_OF_DECK = 52;
public static void main(String[] args) {
ArrayList<Integer> cardsDeck = new ArrayList<>();
for (int i = 0; i < SIZE_OF_DECK; ++i) {
cardsDeck.add(i);
}
System.out.println("Deck Of Cards:" + cardsDeck);
ArrayList<Integer> shuffledDeck = new ArrayList<>();
while (cardsDeck.size() > 0) {
int index = (int) (Math.random() * cardsDeck.size());
int removedCard = cardsDeck.remove(index);
shuffledDeck.add(removedCard);
}
System.out.println("Shuffled Cards" + shuffledDeck);
}
}