How to Create Java Do-While Loop With User Input
-
Use a
do-while
Loop in Java -
the
do-while
Loop Statement -
Take User Input With a
do-while
Loop in Java
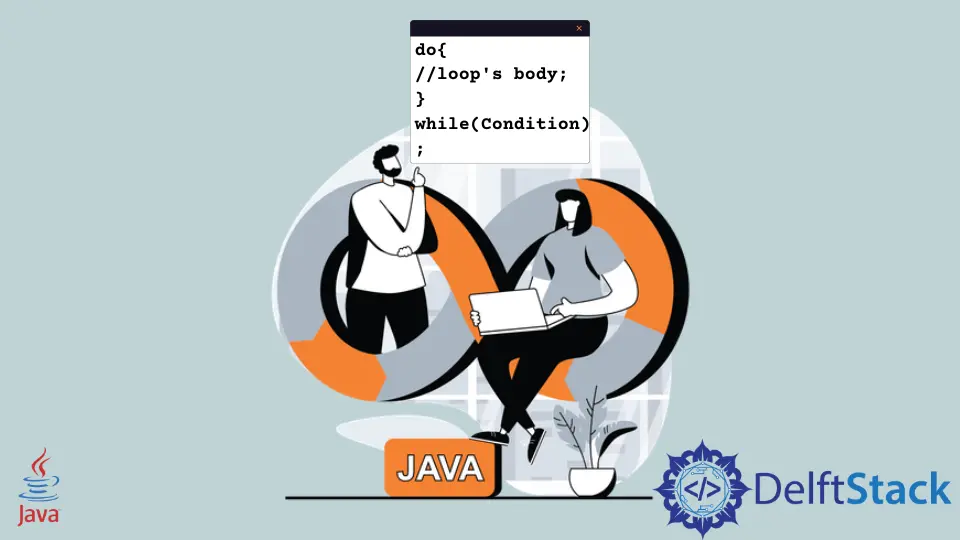
In this article, we’ll discuss using the do while
loop in Java.
Use a do-while
Loop in Java
The do-while
loop is similar to other loops like for
and while
loops in java. It is also used to iterate over and over, depending on a specific condition.
The specialty of the do-while
loop, which makes it unique, is that the do-while
loop executes the body of the loop at least once and then executes the conditional expression of the loop, which can be either true
or false
. The conditional expression must be a Boolean expression.
Syntax:
Do {
// body of the loop;
}
while (Condition)
;
Code Example:
package codes;
public class Codes {
public static void main(String[] args) {
int count = 0;
// Do-While loop
do {
System.out.println("This is the Do part of the loop"); // Body of the loop
}
// Conditional expression of the loop
while (count > 1);
System.out.println(
"The Conditional expression is false so the Loop is terminated in the first iteration ");
}
}
Output:
This is the Do part of the loop
The Conditional expression is false so the Loop is terminated in the first iteration
the do-while
Loop Statement
The working of the do-while
loop is pretty simple. There are two parts of the do-while
loop one is the body part, and the second is the conditional part.
First of all, regardless of the conditional expression, the body will be executed once, and then the conditional expression will check if that’s True
. The loop will continue its execution; otherwise, the loop will be terminated.
Code Example:
package codes;
public class Codes {
public static void main(String[] args) {
int count = 0;
// Do-While loop
do {
System.out.println("Count is " + count); // Body of the loop
}
// Conditional expression of the loop
while (count++ < 9);
}
}
Output:
Count is 0
Count is 1
Count is 2
Count is 3
Count is 4
Count is 5
Count is 6
Count is 7
Count is 8
Count is 9
In this example, the do
part of the loop is executed first, and then the condition is checked until the condition is true
. The loop has iterated accordingly, but as the condition became false
, the loop terminated.
Take User Input With a do-while
Loop in Java
As discussed, the do-while
loop is sometimes a desirable feature of Java programming language because sometimes you would like to execute the body of the loop before the termination of the loop. Just like Displaying a menu, asking to play a game, or even taking a user’s input in the do
part of the loop and then using that input in the conditional expression of the loop.
Taking user’s input in the do-while
loop is one of the most useful cases you will come around to.
Code Example:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
String input;
String buyAgain = null;
do {
System.out.println("********MENU********");
System.out.println("Press 1 for Coke");
System.out.println("Press 2 for Tea");
System.out.println("Press 3 for Orange Juice");
System.out.println("Press 4 for Coffee");
input = scan.next();
if (input.equals("1")) {
System.out.println("Your Coke is ready, Please enjoy it");
} else if (input.equals("2")) {
System.out.println("Please take your Tea and enjoy");
} else if (input.equals("3")) {
System.out.println("Enjoy your Orange juice");
} else if (input.equals("4")) {
System.out.println("Here's your Coffe Please enjoy");
} else {
System.out.println("Invalid input\nPress 1 to view menu");
buyAgain = scan.next();
}
System.out.println("Would you like something else\nPress 1 for yes and 0 for not now");
buyAgain = scan.next();
} while (!buyAgain.equals("0"));
}
}
Output:
********MENU********
Press 1 for Coke
Press 2 for Tea
Press 3 for Orange Juice
Press 4 for Coffee
1
Your Coke is ready, Please enjoy it
Would you like something else
Press 1 for yes and 0 for not now
1
********MENU********
Press 1 for Coke
Press 2 for Tea
Press 3 for Orange Juice
Press 4 for Coffee
0
Invalid input
Press 1 to view menu
1
Would you like something else
Press 1 for yes and 0 for not now
0
In this code, the do
part is used to display the menu and take input from the user, while the conditional part depends on the user input.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn