帶有使用者輸入的 Java Do-While 迴圈
Zeeshan Afridi
2024年2月15日
Java
Java Loop
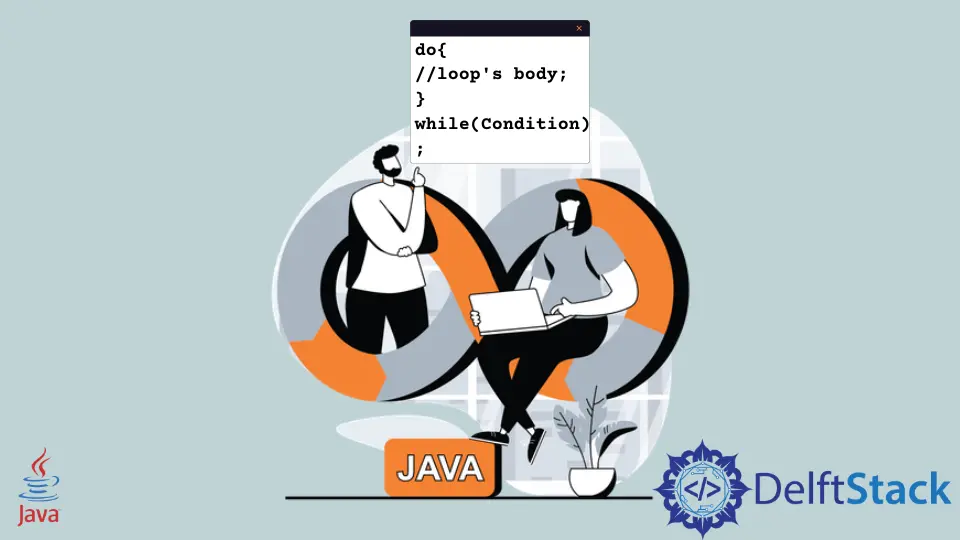
在本文中,我們將討論在 Java 中使用 do while
迴圈。
在 Java 中使用 do-while
迴圈
do-while
迴圈類似於其他迴圈,如 java 中的 for
和 while
迴圈。它還用於根據特定條件反覆迭代。
do-while
迴圈的獨特之處在於它的獨特之處在於 do-while
迴圈至少執行一次迴圈體,然後執行迴圈的條件表示式,可以是 true
或 假
。條件表示式必須是布林表示式。
語法:
Do {
// body of the loop;
}
while (Condition)
;
程式碼示例:
package codes;
public class Codes {
public static void main(String[] args) {
int count = 0;
// Do-While loop
do {
System.out.println("This is the Do part of the loop"); // Body of the loop
}
// Conditional expression of the loop
while (count > 1);
System.out.println(
"The Conditional expression is false so the Loop is terminated in the first iteration ");
}
}
輸出:
This is the Do part of the loop
The Conditional expression is false so the Loop is terminated in the first iteration
do-while
迴圈語句
do-while
迴圈的工作非常簡單。do-while
迴圈有兩部分,一是主體部分,二是條件部分。
首先,無論條件表示式如何,主體都會執行一次,然後條件表示式會檢查是否為 True
。迴圈將繼續執行;否則,迴圈將被終止。
程式碼示例:
package codes;
public class Codes {
public static void main(String[] args) {
int count = 0;
// Do-While loop
do {
System.out.println("Count is " + count); // Body of the loop
}
// Conditional expression of the loop
while (count++ < 9);
}
}
輸出:
Count is 0
Count is 1
Count is 2
Count is 3
Count is 4
Count is 5
Count is 6
Count is 7
Count is 8
Count is 9
在本例中,首先執行迴圈的 do
部分,然後檢查條件,直到條件為 true
。迴圈相應地進行了迭代,但隨著條件變為假
,迴圈終止。
在 Java 中使用 do-while
迴圈獲取使用者輸入
正如所討論的,do-while
迴圈有時是 Java 程式語言的理想特性,因為有時你希望在迴圈終止之前執行迴圈體。就像顯示選單,要求玩遊戲,甚至在迴圈的 do
部分中獲取使用者的輸入,然後在迴圈的條件表示式中使用該輸入。
在 do-while
迴圈中接受使用者的輸入是你將遇到的最有用的情況之一。
程式碼示例:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
String input;
String buyAgain = null;
do {
System.out.println("********MENU********");
System.out.println("Press 1 for Coke");
System.out.println("Press 2 for Tea");
System.out.println("Press 3 for Orange Juice");
System.out.println("Press 4 for Coffee");
input = scan.next();
if (input.equals("1")) {
System.out.println("Your Coke is ready, Please enjoy it");
} else if (input.equals("2")) {
System.out.println("Please take your Tea and enjoy");
} else if (input.equals("3")) {
System.out.println("Enjoy your Orange juice");
} else if (input.equals("4")) {
System.out.println("Here's your Coffe Please enjoy");
} else {
System.out.println("Invalid input\nPress 1 to view menu");
buyAgain = scan.next();
}
System.out.println("Would you like something else\nPress 1 for yes and 0 for not now");
buyAgain = scan.next();
} while (!buyAgain.equals("0"));
}
}
輸出:
********MENU********
Press 1 for Coke
Press 2 for Tea
Press 3 for Orange Juice
Press 4 for Coffee
1
Your Coke is ready, Please enjoy it
Would you like something else
Press 1 for yes and 0 for not now
1
********MENU********
Press 1 for Coke
Press 2 for Tea
Press 3 for Orange Juice
Press 4 for Coffee
0
Invalid input
Press 1 to view menu
1
Would you like something else
Press 1 for yes and 0 for not now
0
在這段程式碼中,do
部分用於顯示選單並接受使用者輸入,而條件部分取決於使用者輸入。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Zeeshan Afridi
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn