帶有使用者輸入的 Java while 迴圈
Sheeraz Gul
2024年2月16日
Java
Java Loop
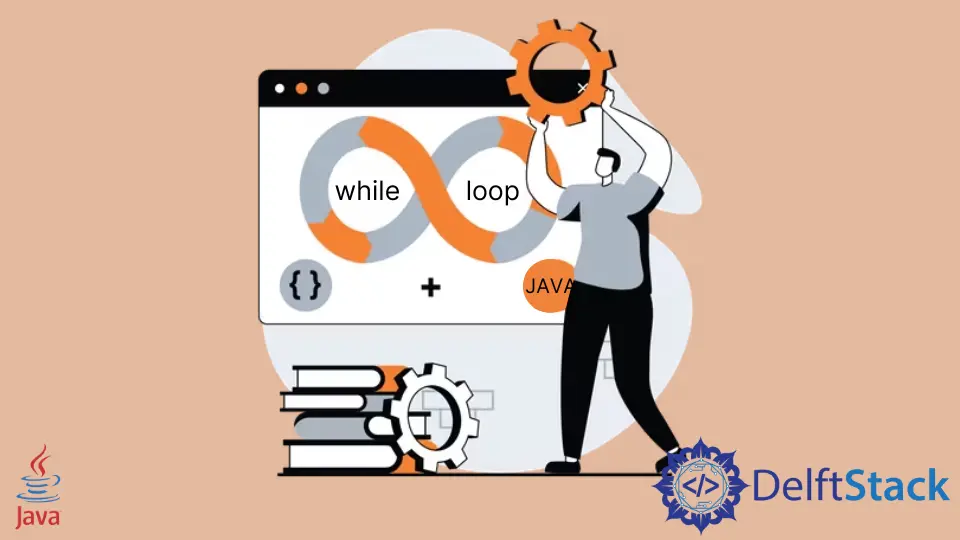
有時,在使用迴圈時,我們在迴圈執行期間需要多個使用者輸入。本教程演示瞭如何在 Java 中建立一個不斷請求使用者輸入的 while
迴圈。
在 Java 中使用帶有使用者輸入的 while
迴圈
我們將使用使用者輸入建立一個 while
迴圈。此示例將檢查陣列中是否存在數字。
迴圈將繼續接受使用者輸入,直到輸入數字不是陣列的成員。
程式碼:
import java.util.*;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] input_array = {10, 12, 14, 17, 19, 21};
System.out.println(Arrays.toString(input_array));
System.out.println("Enter number to check if it is a member of the array");
while (true) {
Scanner input_number = new Scanner(System.in);
int number = input_number.nextInt();
if (Arrays.stream(input_array).anyMatch(i -> i == number)) {
System.out.println(number + " is the member of given array");
} else {
System.out.println(number + " is not the member of given array");
System.out.println("The While Loop Breaks Here");
break;
}
}
}
}
讓我們嘗試多個輸入,直到迴圈中斷。當輸入一個不是陣列成員的數字時,迴圈將中斷。
輸出:
[10, 12, 14, 17, 19, 21]
Enter number to check if it is a member of the array
10
10 is the member of given array
12
12 is the member of given array
13
13 is not the member of given array
The While Loop Breaks Here
執行程式碼這裡。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook