사용자 입력이 있는 Java Do-While 루프
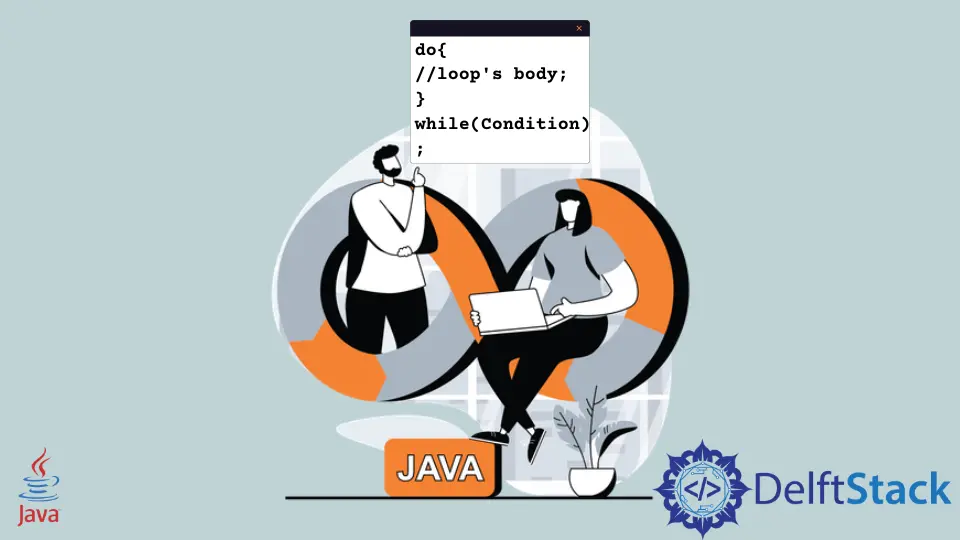
이 기사에서는 Java에서 do while
루프를 사용하는 방법에 대해 설명합니다.
Java에서 do-while
루프 사용
do-while
루프는 Java의 for
및 while
루프와 같은 다른 루프와 유사합니다. 또한 특정 조건에 따라 계속해서 반복하는 데 사용됩니다.
독특하게 만드는 do-while
루프의 특수성은 do-while
루프가 루프의 본문을 최소한 한 번 실행한 다음 true일 수 있는 루프의 조건부 표현식을 실행합니다.
또는 거짓
. 조건식은 부울 식이어야 합니다.
통사론:
Do {
// body of the loop;
}
while (Condition)
;
코드 예:
package codes;
public class Codes {
public static void main(String[] args) {
int count = 0;
// Do-While loop
do {
System.out.println("This is the Do part of the loop"); // Body of the loop
}
// Conditional expression of the loop
while (count > 1);
System.out.println(
"The Conditional expression is false so the Loop is terminated in the first iteration ");
}
}
출력:
This is the Do part of the loop
The Conditional expression is false so the Loop is terminated in the first iteration
do-while
루프 문
do-while
루프의 작업은 매우 간단합니다. do-while
루프에는 두 부분이 있습니다. 하나는 본문 부분이고 두 번째 부분은 조건 부분입니다.
우선 조건식에 관계없이 본문을 한 번 실행한 다음 조건식이 True
인지 확인합니다. 루프는 계속 실행됩니다. 그렇지 않으면 루프가 종료됩니다.
코드 예:
package codes;
public class Codes {
public static void main(String[] args) {
int count = 0;
// Do-While loop
do {
System.out.println("Count is " + count); // Body of the loop
}
// Conditional expression of the loop
while (count++ < 9);
}
}
출력:
Count is 0
Count is 1
Count is 2
Count is 3
Count is 4
Count is 5
Count is 6
Count is 7
Count is 8
Count is 9
이 예에서 루프의 do
부분이 먼저 실행된 다음 조건이 true
가 될 때까지 조건이 확인됩니다. 루프는 그에 따라 반복되었지만 조건이 false
가 되면서 루프가 종료되었습니다.
Java에서 do-while
루프를 사용하여 사용자 입력 받기
논의한 바와 같이 do-while
루프는 때때로 루프가 종료되기 전에 루프 본문을 실행하고 싶기 때문에 Java 프로그래밍 언어의 바람직한 기능입니다. 메뉴 표시, 게임 요청, 루프의 do
부분에서 사용자 입력을 받은 다음 루프의 조건식에서 해당 입력을 사용하는 것과 같습니다.
do-while
루프에서 사용자 입력을 받는 것은 가장 유용한 경우 중 하나입니다.
코드 예:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
String input;
String buyAgain = null;
do {
System.out.println("********MENU********");
System.out.println("Press 1 for Coke");
System.out.println("Press 2 for Tea");
System.out.println("Press 3 for Orange Juice");
System.out.println("Press 4 for Coffee");
input = scan.next();
if (input.equals("1")) {
System.out.println("Your Coke is ready, Please enjoy it");
} else if (input.equals("2")) {
System.out.println("Please take your Tea and enjoy");
} else if (input.equals("3")) {
System.out.println("Enjoy your Orange juice");
} else if (input.equals("4")) {
System.out.println("Here's your Coffe Please enjoy");
} else {
System.out.println("Invalid input\nPress 1 to view menu");
buyAgain = scan.next();
}
System.out.println("Would you like something else\nPress 1 for yes and 0 for not now");
buyAgain = scan.next();
} while (!buyAgain.equals("0"));
}
}
출력:
********MENU********
Press 1 for Coke
Press 2 for Tea
Press 3 for Orange Juice
Press 4 for Coffee
1
Your Coke is ready, Please enjoy it
Would you like something else
Press 1 for yes and 0 for not now
1
********MENU********
Press 1 for Coke
Press 2 for Tea
Press 3 for Orange Juice
Press 4 for Coffee
0
Invalid input
Press 1 to view menu
1
Would you like something else
Press 1 for yes and 0 for not now
0
이 코드에서 do
부분은 메뉴를 표시하고 사용자로부터 입력을 받는 데 사용되는 반면 조건 부분은 사용자 입력에 따라 다릅니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn