Java에서 forEach와 함께 인덱스 사용
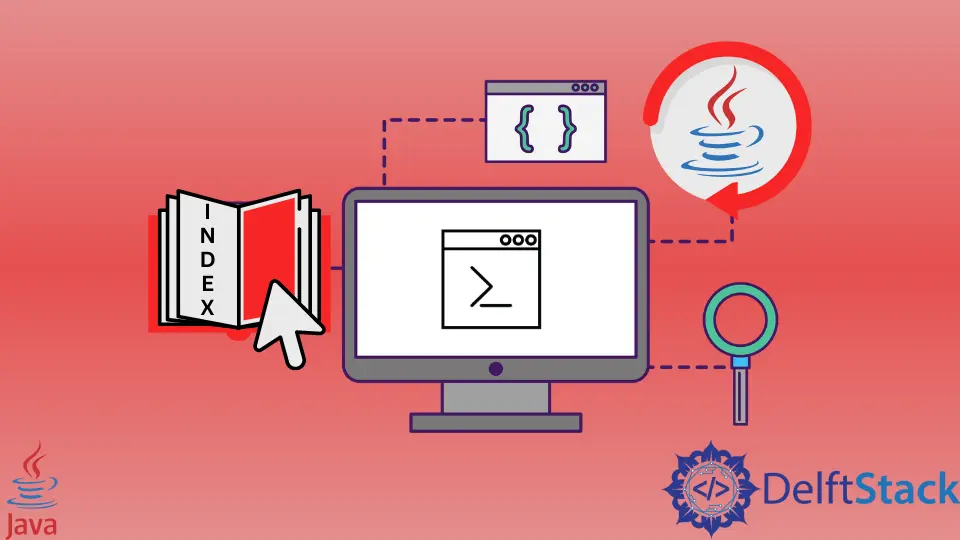
특정 색인과 함께 Java forEach()
메소드를 사용하려면 이 Java 기사를 따를 수 있습니다. 경우에 따라 특정 인덱스와 관련된 특정 작업을 수행해야 합니다.
이 글에서는 forEach()
함수를 인덱스 조합과 함께 사용하는 방법에 대해 알아봅니다. 또한 주제를 쉽게 이해할 수 있도록 필요한 예와 설명을 사용하여 주제를 다룰 것입니다.
일반적으로 forEach()
메서드는 인덱스를 사용할 수 없지만 몇 가지 방법이 있습니다. 이를 위해 IntStream.range()
메서드를 사용해야 합니다.
한 가지 예를 살펴보겠습니다.
배열 인덱스와 함께 forEach()
메서드 사용
아래 예제에서는 배열의 특정 인덱스와 함께 forEach()
메서드를 사용하는 방법을 보여줍니다. 예제 코드는 다음과 같습니다.
// importing necessary packages
import java.util.stream.IntStream;
public class JavaForEachIndex {
public static void main(String[] args) {
// Creating an array of string
String[] StrArr = {"A", "B", "C", "D", "E"};
// Finding the length of the string array
int Len = StrArr.length;
// Using forEach with index
IntStream.range(0, Len).forEach(index
-> System.out.println("Value of array at Index : " + (index + 1) + " = " + StrArr[index]));
}
}
우리는 이미 각 라인의 목적을 명령했습니다. 이제 예제 코드를 실행하면 아래 출력이 표시됩니다.
Value of array at Index : 1 = A
Value of array at Index : 2 = B
Value of array at Index : 3 = C
Value of array at Index : 4 = D
Value of array at Index : 5 = E
목록 및 HashMap 인덱스와 함께 forEach()
메서드 사용
아래 예제에서는 특정 목록 인덱스와 함께 forEach()
메서드를 사용하는 방법을 보여줍니다. 예제의 코드는 다음과 같습니다.
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
public class JavaForEachIndex {
public static void main(String[] args) {
// Creating a list of string
List<String> StrList = Arrays.asList("A", "B", "C", "D", "E");
// Creating a HashMap
// Put the List of String to the HashMap
HashMap<Integer, String> Collection = StrList.stream().collect(HashMap<Integer, String>::new,
(MyMap, StreamVal) -> MyMap.put(MyMap.size(), StreamVal), (MyMap, map2) -> {});
// Using the forEach with index
Collection.forEach(
(index, val) -> System.out.println("Value of list element at " + index + " = " + val));
}
}
우리는 이미 각 라인의 목적을 명령했습니다. 이제 예제 코드를 실행하면 콘솔에 아래와 같은 출력이 표시됩니다.
Value of list element at 0 = A
Value of list element at 1 = B
Value of list element at 2 = C
Value of list element at 3 = D
Value of list element at 4 = E
여기에서 공유되는 코드 예제는 Java로 되어 있으며 시스템에 Java가 포함되어 있지 않은 경우 환경에 Java를 설치해야 합니다.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn