How to Return Two Values in Java Function
-
Return Multiple Values Using the
ArrayList
Class in Java -
Return Two Values Using the
Pair
Class in Java
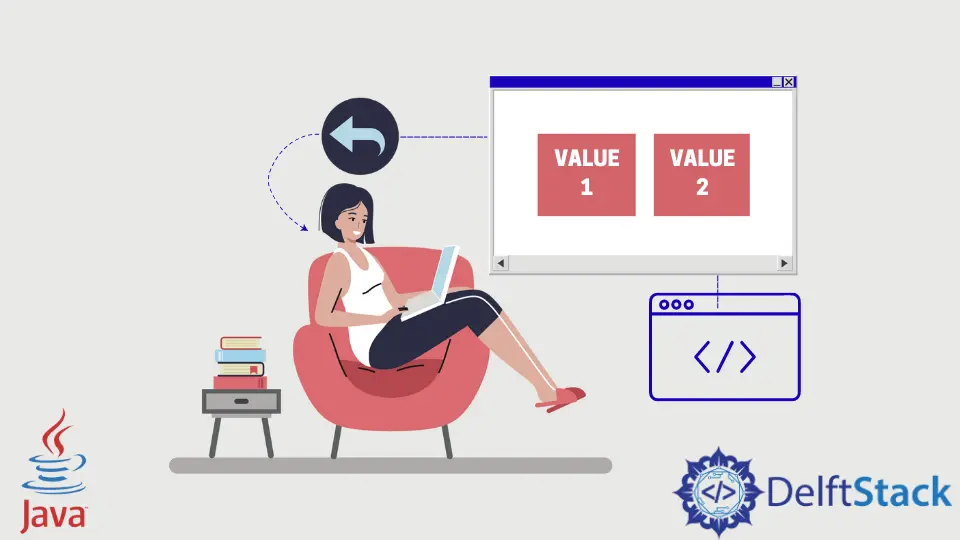
In Java, there is no privilege to return two values to a function. The resolution to the problem that comes with multi-value returns can be solved by either creating a user-defined class to store the output or using built-in datatypes as Array
, Pair
(if only two values are there to return), HashMap
and String Array
.
Return Multiple Values Using the ArrayList
Class in Java
Below is the small code block to demonstrate the Java functions that return more than two values.
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
ArrayList<String> list = callFunction();
list.forEach(System.out::println);
}
private static ArrayList<String> callFunction() {
return new ArrayList<>(Arrays.asList("Hi", "Hello"));
}
}
In the code above, a method is defined to understand the return functionality in Java. The callFunction
method is called from the main
function or driver class; it is private to the JavaReturnTwoValues
class.
In the function definition, an ArrayList
object gets instantiated initially with two values. The values are allowed in the public class constructor. Using the Arrays.asList()
function allows inline instantiation, hence, defining the values at declaration time.
The static asList
method returns a defined list specified as parameters. The return
keyword always accepts a single parameter after it. If more than one parameter is specified, it throws compile-time error
issues.
The values from the function are preserved in the list
local variable. Over the local variable, the forEach()
method is called. This specified method takes a Consumer
instance, a functional interface with a single accept
process. It means that it only consumes values or classes and does not return anything.
So, the statement emits the elements sequentially from the list instance, and the forEach
method consumes the elements to print the values on the console. The ::
is a method reference operator and is similar to a lambda expression, a-> System.out.println(a)
.
The output of the code block above is shown below.
Hi Hello World
Return Two Values Using the Pair
Class in Java
Below is the code block that uses the user-defined Pair
class to understand its functionality.
public class Main {
public static void main(String[] args) {
Pair<String, String> pair = callPairFunction();
System.out.println(pair.getKey() + " " + pair.getValue());
}
private static Pair<String, String> callPairFunction() {
return new Pair<String, String>("Key", "I am value");
}
}
class Pair<T1, T2> {
private final T1 key;
private final T2 value;
public Pair(T1 first, T2 second) {
this.key = first;
this.value = second;
}
public T1 getKey() {
return key;
}
public T2 getValue() {
return value;
}
}
In the program above, there are two classes defined: one is the public class that holds the main
or driver function, and the other is the user-defined Pair
class to illustrate the functionality of the pair internally.
The driver class has code for calling the function and value manipulation process. The callPairFunction
is a private method that returns a Pair
user-defined datatype. The class gets declared to store a pair of values that is the key and its value.
Below the main class is a user-defined class, Pair<T1, T2>
. It is generically defined as and works on the concept of Generics
in Java. The parameters T1
and T2
are generic data types, meaning they can take the form of the datatype that gets passed. In our case, the string is passed, so T1
and T2
become of the type String
.
The class has a public constructor that instantiates the local variables with the values passed. Using the public getKey
and getValue
methods, the key and value are returned after getting instantiated in the constructor.
In the callPairFunction
method, an instance of the Pair
class is returned using a new keyword. The statement invokes the public constructor of the class and sets the key and value variables. The instance returned is used to retrieve the values on the left and right sides of the Pair class.
Below is the output defined in the code above.
Key I am value
Notably, the org.apache.commons.lang3.tuple
package also provides a similar built-in class for usage. Pair
is a class present in the package that holds existing functions and operations to work on the values.
One can often use a pre-defined class to avoid writing the cumbersome code and be overhead of creating a new Pair
class. The dependency to inject in the project is shown here.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-configuration2</artifactId>
<version>2.7</version>
</dependency>
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedInRelated Article - Java Function
- How to Return a Boolean Method in Java
- Covariant Return Type in Java
- How to Write an Anonymous Function in Java
- How to Use System.gc() for Garbage Collection in Java
- How to Create Callback Functions in Java
- How to Return Nothing From a Function in Java