How to Return Nothing From a Function in Java
- Return Nothing in an Integer Function in Java
- Return Nothing in a String Function in Java
- Return Nothing in Any Object Function in Java
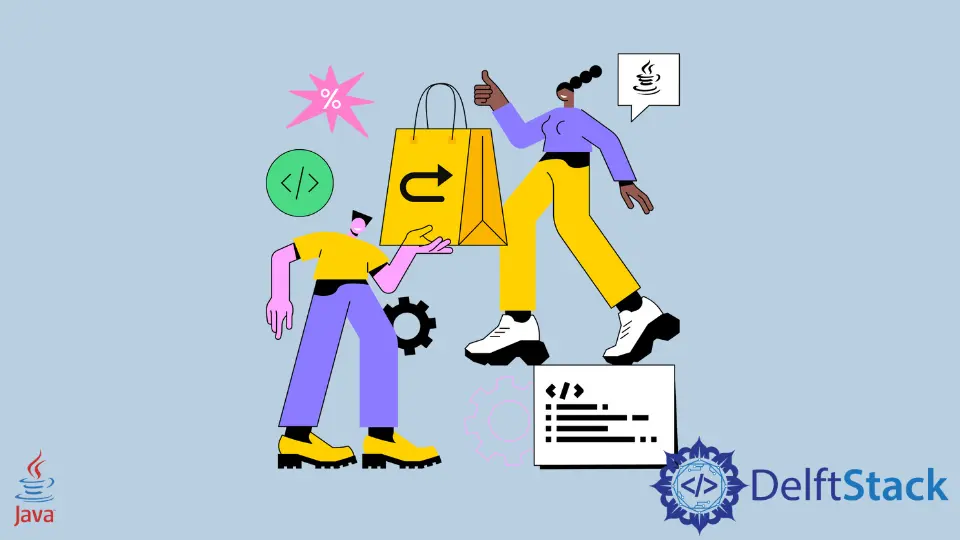
This tutorial introduces how to return nothing in function in Java.
Every function that has a return type in its signature must return a value of the same type, else the compile will generate an error, and the code will not execute. It means a return value is a value that is returned to the caller function, and if we wish to return nothing, then we will have to return some default value.
For example, we can return null
for String type and 0
for the integer value to handle such a situation. Let’s understand with some examples.
Return Nothing in an Integer Function in Java
In this example, we have a static method that returns an integer, but we want to return nothing in a special case, then we return 0
only. Although there is nothing in integer to return, we can do this to achieve our objective.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int result = getMax(12, 12);
System.out.println(result);
}
public static int getMax(int a, int b) {
if (a > b) {
return a;
} else if (b > a) {
return b;
}
return 0; // similar to nothing in int
}
}
Output:
0
Return Nothing in a String Function in Java
In this example, we have a static method that returns a String, but we want to return nothing in a special case, then we return null
only. Although there is nothing like nothing in String to return, we can achieve our objective.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String result = getMax("rohan", "sohan");
System.out.println("result " + result);
result = getMax("sohan", "sohan");
System.out.println("result " + result);
}
public static String getMax(String a, String b) {
if (a.compareTo(b) > 0) {
return a;
} else if (a.compareTo(b) < 0) {
return b;
}
return null; // similar to nothing in String
}
}
Output:
result sohan
result null
Return Nothing in Any Object Function in Java
In this example, we have a static method that returns an integer object, but we want to return nothing in a special case, then we return null
only as null
is a default value for objects in Java. Although there is nothing like nothing in objects to return, we can achieve our objective.
We can use this for any other object as well. See the example below.
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
list.add(23);
list.add(32);
list.add(33);
System.out.println(list);
Integer result = getMax(list);
System.out.println("result " + result);
}
public static Integer getMax(List<Integer> list) {
if (Collections.max(list) > 100) {
return 100;
} else if (Collections.max(list) < 50) {
return Collections.max(list);
}
return null; // similar to nothing in Integer object
}
}
Output:
[23, 32, 33]
result 33