How to Use System.gc() for Garbage Collection in Java
Sheeraz Gul
Feb 02, 2024
Java
Java Function
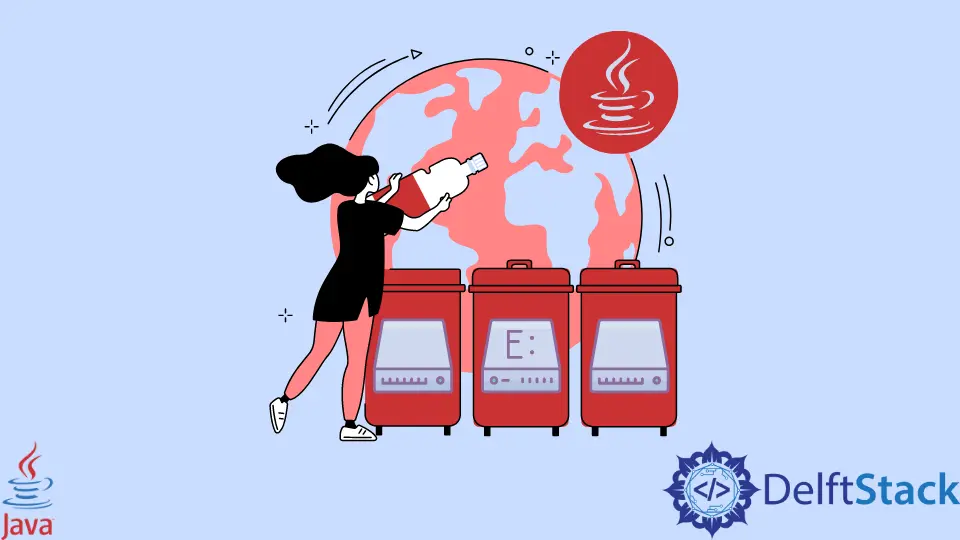
System.gc()
is an API provided in Java for garbage collection, which performs automatic memory management.
When we run a Java program, there might be some objects or data which is not needed anymore, System.gc()
collects that data and deletes them to free up the memory.
This tutorial will demonstrate how to use the System.gc()
in Java.
Demonstrate the Use of System.gc()
in Java
The System.gc()
can be invoked by the system, developer, or the external tools used for the applications.
Example:
package delftstack;
public class System_Gc {
public static void main(String... args) {
for (int x = 1; x < 15; x++) {
Demo_Class New_Demo_Class = new Demo_Class(x);
System.out.printf("Demo Class Generated, Demo= %s%n", New_Demo_Class.get_Demo());
System.gc();
}
}
public static class Demo_Class {
private final int Demo;
public Demo_Class(int Demo) {
this.Demo = Demo;
}
public int get_Demo() {
return Demo;
}
// the garbage collector will call this method each time before removing the object from memory.
@Override
protected void finalize() throws Throwable {
System.out.printf("-- %s is getting collected in the garbage --%n", Demo);
}
}
}