Java 中用於垃圾回收的 System.gc()
Sheeraz Gul
2023年10月12日
Java
Java Function
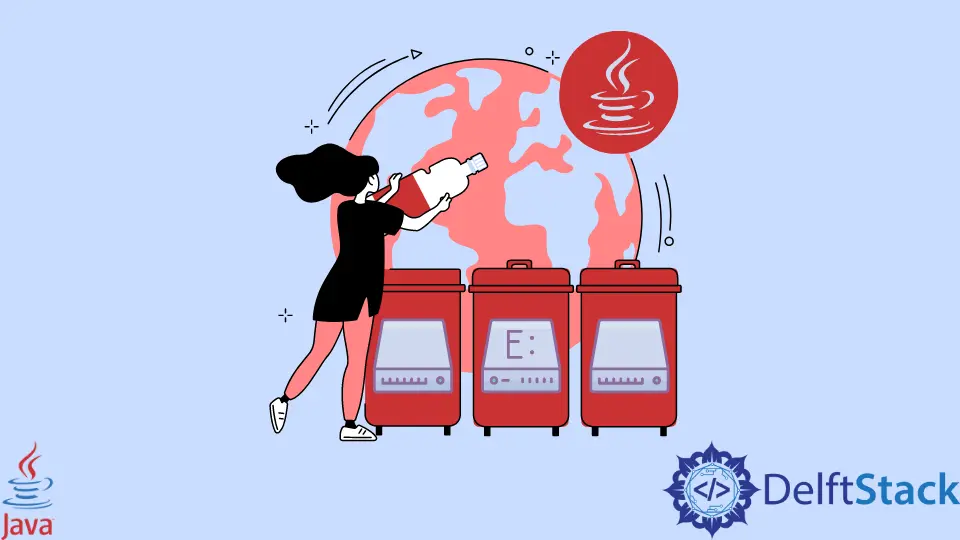
System.gc()
是 Java 中提供的用於垃圾收集的 API,它執行自動記憶體管理。
當我們執行 Java 程式時,可能會有一些不再需要的物件或資料,System.gc()
收集這些資料並刪除它們以釋放記憶體。
本教程將演示如何在 Java 中使用 System.gc()
。
在 Java 中演示使用 System.gc()
System.gc()
可以由系統、開發人員或用於應用程式的外部工具呼叫。
例子:
package delftstack;
public class System_Gc {
public static void main(String... args) {
for (int x = 1; x < 15; x++) {
Demo_Class New_Demo_Class = new Demo_Class(x);
System.out.printf("Demo Class Generated, Demo= %s%n", New_Demo_Class.get_Demo());
System.gc();
}
}
public static class Demo_Class {
private final int Demo;
public Demo_Class(int Demo) {
this.Demo = Demo;
}
public int get_Demo() {
return Demo;
}
// the garbage collector will call this method each time before removing the object from memory.
@Override
protected void finalize() throws Throwable {
System.out.printf("-- %s is getting collected in the garbage --%n", Demo);
}
}
}
上面的程式碼給出了 System.gc()
的示例用法,其中一個方法被呼叫了 14 次,系統收集了 13 次垃圾。
輸出:
Demo Class Generated, Demo= 1
Demo Class Generated, Demo= 2
Demo Class Generated, Demo= 3
-- 1 is getting collected in the garbage --
Demo Class Generated, Demo= 4
-- 2 is getting collected in the garbage --
Demo Class Generated, Demo= 5
-- 3 is getting collected in the garbage --
Demo Class Generated, Demo= 6
-- 4 is getting collected in the garbage --
Demo Class Generated, Demo= 7
-- 5 is getting collected in the garbage --
Demo Class Generated, Demo= 8
-- 6 is getting collected in the garbage --
Demo Class Generated, Demo= 9
-- 7 is getting collected in the garbage --
Demo Class Generated, Demo= 10
-- 8 is getting collected in the garbage --
-- 9 is getting collected in the garbage --
Demo Class Generated, Demo= 11
Demo Class Generated, Demo= 12
-- 10 is getting collected in the garbage --
-- 11 is getting collected in the garbage --
Demo Class Generated, Demo= 13
-- 12 is getting collected in the garbage --
Demo Class Generated, Demo= 14
-- 13 is getting collected in the garbage --
System.gc()
不是主要由開發人員使用,因為他們認為它沒有用,但它是處理快取的好工具。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook