在 Java 中建立回撥函式
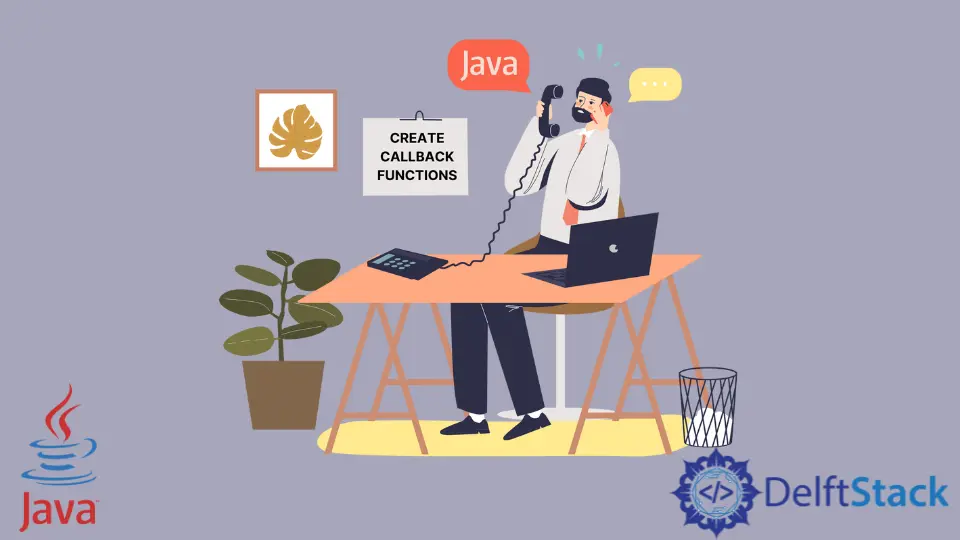
回撥功能用於事件驅動程式設計。當相應事件發生時,引用將傳遞給呼叫的函式。
我們使用介面來實現 Java 中的回撥,因為它不支援函式指標。
本教程演示如何在 Java 中建立和使用回撥函式。
在 Java 中使用介面建立回撥函式
Java 中的介面是指定類行為的抽象型別。它是一個類的藍圖類。
我們可以建立一個介面和多個類來演示 Java 中的回撥。
下面的程式碼實現了一個介面和四個類來計算員工的工資。要呼叫函式,我們傳遞介面的引用;這是回撥。
該程式碼通過從總工資中減去 10% 來計算淨工資。檢視每個類的輸出;我們執行程式碼四次。
import java.util.Scanner;
// Create interface
interface Salary {
double Person_Salary();
}
// Class for Jack's Salary
class Jack implements Salary {
public double Person_Salary() {
return 5000.0;
}
}
// Class for Michelle's Salary
class Michelle implements Salary {
public double Person_Salary() {
return 4000.0;
}
}
// Class for Jhonny's Salary
class Jhonny implements Salary {
public double Person_Salary() {
return 3000.0;
}
}
// Class for Mike's Salary
class Mike implements Salary {
public double Person_Salary() {
return 3500.0;
}
}
class Employee_Salary {
public static void main(String[] args)
throws ClassNotFoundException, IllegalAccessException, InstantiationException {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the Employee Name: ");
// name of the employee
String Employee = sc.next();
// Store the employee name in an object temp
Class temp = Class.forName(Employee);
// Create the new object of the class whose name is in temp
// Interface Salary's reference is now referencing the new object created above
Salary reference = (Salary) temp.newInstance();
// Call the method to calculate net salary and pass interface reference - this is a callback.
// Here reference refers to the function Person_Salary() of both Jack and Michelle's classes
Calculate_Salary(reference);
}
static void Calculate_Salary(Salary E_Salary) {
// calculate Salary Deduction Which is 10 percent
double salary_deduction = E_Salary.Person_Salary() * 0.1;
// The gross salary
double gross_salary = E_Salary.Person_Salary();
// Calculate the net salary
double net_salary = gross_salary - salary_deduction;
// Show the net salary
System.out.println("The Net Salary of Employee is =" + net_salary);
}
}
輸出:
Enter the Employee Name:
Jack
The Net Salary of Employee is =4500.0
Enter the Employee Name:
Michelle
The Net Salary of Employee is =3600.0
Enter the Employee Name:
Jhonny
The Net Salary of Employee is =2700.0
Enter the Employee Name:
Mike
The Net Salary of Employee is =3150.0
在 Java 中建立同步和非同步回撥函式
同步回撥
程式碼執行將等待或阻止同步回撥的事件,直到它返回響應。回撥將在返回撥用語句之前執行其所有工作。
同步有時似乎滯後。下面的程式碼為回撥函式實現了一個簡單的同步任務。
// Create Interface
interface Delftstack {
void delftstack_event();
}
class Delftstack_Two {
// Delftstack Field
private Delftstack Delft;
// setting the register_delftstack function
public void register_delftstack(Delftstack Delft) {
this.Delft = Delft;
}
// This is our synchronous task
public void Hello_Delftstack() {
// perform any operation
System.out.println("Hello! This is delftstack callback from before the synchronous task.");
// check if listener is registered.
if (this.Delft != null) {
// invoke the callback method of class A
Delft.delftstack_event();
}
}
// Main
public static void main(String[] args) {
Delftstack_Two Demo_Object = new Delftstack_Two();
Delftstack Delft = new Delftstack_One();
Demo_Object.register_delftstack(Delft);
Demo_Object.Hello_Delftstack();
}
}
class Delftstack_One implements Delftstack {
@Override
public void delftstack_event() {
System.out.println("Hello! This is delftstack callback from after the synchronous task.");
}
}
輸出:
Hello! This is delftstack callback from before the synchronous task.
Hello! This is delftstack callback from after the synchronous task.
非同步回撥
另一方面,非同步回撥不會阻止程式碼執行。
在 Java 中,我們需要建立一個執行緒來開發一個非同步任務,並在裡面實現回撥。當呼叫從事件返回時,它返回到非同步的回撥函式。
下面的程式碼示例為回撥函式實現了一個簡單的非同步任務。
// Create Interface
interface Delftstack {
void delftstack_event();
}
class Delftstack_Two {
// Delftstack Field
private Delftstack Delft;
// setting the register_delftstack function
public void register_delftstack(Delftstack Delft) {
this.Delft = Delft;
}
// The Asynchronous
public void Hello_Delftstack() {
// An Asynchronous must be executed in a new thread
new Thread(new Runnable() {
public void run() {
System.out.println("Hello! This is delftstack operation inside the asynchronous task.");
// check if Delft is registered.
if (Delft != null) {
// invoke the callback method of class A
Delft.delftstack_event();
}
}
}).start();
}
// Main
public static void main(String[] args) {
Delftstack_Two Demo_Object = new Delftstack_Two();
Delftstack Delft = new Delftstack_One();
Demo_Object.register_delftstack(Delft);
Demo_Object.Hello_Delftstack();
}
}
class Delftstack_One implements Delftstack {
@Override
public void delftstack_event() {
System.out.println("Hello! This is delftstack callback from after the asynchronous task.");
}
}
輸出:
Hello! This is delftstack operation inside the asynchronous task.
Hello! This is delftstack callback from after the asynchronous task.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook