How to Write an Anonymous Function in Java
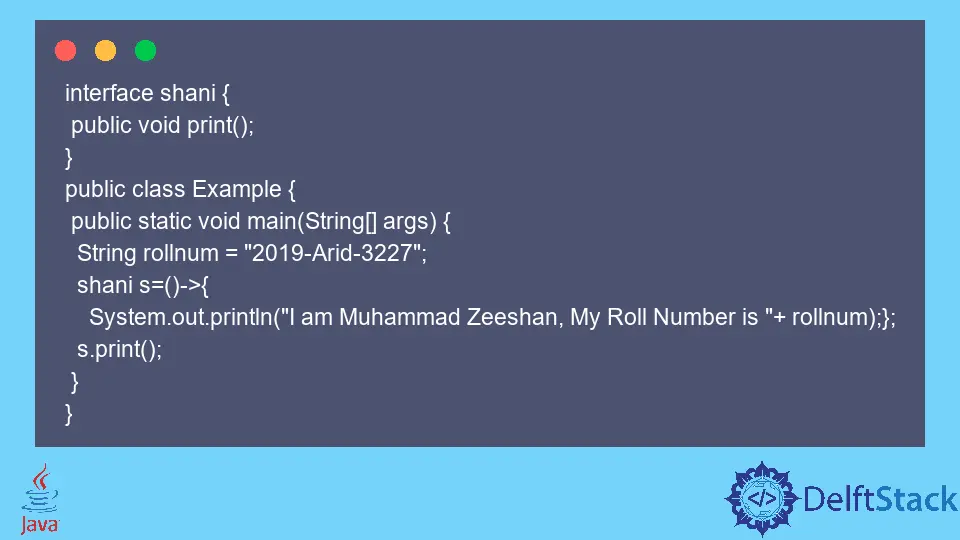
This quick tutorial will look at creating an anonymous function in Java. Anonymous methods are now supported in Java 8 due to the lambda expression feature included in this version.
The following is an explanation of how the lambda expression works in Java.
Lambda Expression
The lambda expression is often employed to offer an implementation of an interface with a functional interface. It clears out a lot of unnecessary code.
If we use a lambda expression, we do not need to create the method once again to provide the implementation.
Simply putting the implementation code in place is all left to do here. It offers a simple notation for expressing the interface of a single method.
Syntax:
(Argument) -> {body of lambda}
The lambda operator is flexible and can operate with various argument numbers, as seen below.
-
With no argument:
() -> { }
-
With one argument:
(num1) -> { }
-
With two arguments:
(num2) -> { }
Use Lambda Expression to Write an Anonymous Function in Java
Let’s have a Java example to understand lambda expressions with no parameters. The lambda expression works as an implementation of the Functional Interface in the following example.
The following program will print the roll number.
-
First, we created an interface and a
print()
function.interface shani { public void print(); }
-
Initialize a
string
variable and populate it with data.String rollnum = "2019-Arid-3227";
-
Lastly, we’ll utilize the lambda expression to display the roll number.
shani s = () -> { System.out.println("I am Muhammad Zeeshan, My Roll Number is " + rollnum); }; s.print();
Complete source code:
interface shani {
public void print();
}
public class Example {
public static void main(String[] args) {
String rollnum = "2019-Arid-3227";
shani s = () -> {
System.out.println("I am Muhammad Zeeshan, My Roll Number is " + rollnum);
};
s.print();
}
}
Output:
I am Muhammad Zeeshan, My Roll Number is 2019-Arid-3227
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn