How to Fix Java Error: Scanner NextLine Skips
-
Understanding
nextLine()
Method -
Why Do Java
Scanner NextLine Skips
Occur? -
How to Fix This Problem of Java
Scanner NextLine Skips
- Conclusion
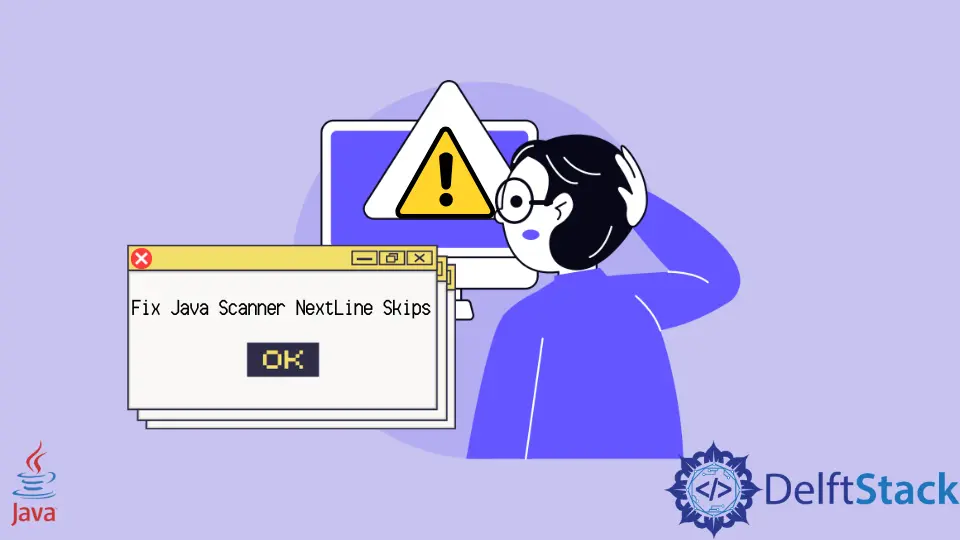
In this article, we explore the common occurrence of Java Scanner nextLine
skips and present straightforward methods to prevent these issues, ensuring a smoother and more reliable user input experience.
Understanding nextLine()
Method
Before we look into the issue with the nextLine()
method, let us first understand the working of the nextLine()
method. This method belongs to the java.util.Scanner
class.
Syntax:
public String nextLine()
This function prints the entire line except for the line separator at the end.
It searches for an input, looking for a line separator. If no line separator is found, it may search all the input while searching for the line to skip.
This scanner method goes past the current line. If there is any input that was skipped, it returns it. Follow this link to refer to the method’s documentation.
Why Do Java Scanner NextLine Skips
Occur?
The Scanner.nextLine()
method in Java reads the current line of input but stops reading at the newline character ('\n'
). If you have used other Scanner
methods (e.g., nextInt()
, nextDouble()
) before calling nextLine()
, there might be a leftover newline character in the input buffer.
When nextLine()
is invoked, it consumes this newline character and returns an empty string, leading to the perception that it skips
the input.
Let’s start with a simple Java program that showcases the Scanner nextLine
skips:
import java.util.Scanner;
public class ScannerExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter an integer: ");
int intValue = scanner.nextInt();
System.out.print("Enter a string: ");
String stringValue = scanner.nextLine();
System.out.println("Integer: " + intValue);
System.out.println("String: " + stringValue);
}
}
In the presented code snippet, a Scanner
object is initialized to capture input from the standard input stream (System.in
).
The user is prompted to input an integer and, subsequently, a string. The process unfolds as follows:
We create a Scanner
object named scanner
to handle input reading. Following this, the program prompts the user for an integer input, employing the nextInt()
method.
While the integer is successfully captured, it’s important to note that this method does not consume the newline character (Enter key) in the input buffer. Consequently, after entering the integer, an unconsumed newline character ('\n'
) remains in the input buffer.
The crux of the matter lies in the fact that when attempting to read the string using nextLine()
, it consumes the leftover newline character from the preceding input.
This behavior leads to an unexpected outcome, giving the impression that the program skips the user’s input. This intricacy underscores the importance of considering newline characters in the input buffer when using different Scanner
methods for input reading in Java.
If you were to run this code and enter an integer followed by a string, the output might look like this:
Notice that the string value is an empty string (""
). This unexpected behavior occurs due to the newline character being consumed by nextLine()
.
The provided code example sheds light on this common pitfall, empowering developers to write cleaner and more resilient code when handling user input in Java.
How to Fix This Problem of Java Scanner NextLine Skips
Using the Java Integer.parseInt()
Method
The parseInt()
method belongs to the Integer
class of the java.lang
package and returns the primitive data type of a certain string. These methods are of two types mainly:
Integer.parseInt(String s)
Integer.parseInt(String s, int radix)
To work with the nextLine()
skips, we will use the first method - Integer.parseInt(String s)
. This reads the complete line for the Integer as a String. Later, it converts it to an integer.
By incorporating the Integer.parseInt()
method and a custom input handling mechanism, we can successfully mitigate the challenges posed by nextLine
skips in Java Scanner
. This approach not only ensures a smoother user experience but also provides a robust solution for handling different types of input in Java programs.
Syntax:
int value = Integer.parseInt(sc.nextLine());
The parseInt()
method not only allows for streamlined integer input handling but also helps prevent unwanted nextLine
skips, providing a more consistent and reliable user experience.
Let’s delve into a practical example demonstrating how to fix Java Scanner nextLine skips
using the parseInt()
method:
import java.util.Scanner;
public class ScannerFixExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter an integer: ");
int intValue = readInt(scanner);
System.out.print("Enter a string: ");
String stringValue = scanner.nextLine();
System.out.println("Integer: " + intValue);
System.out.println("String: " + stringValue);
}
private static int readInt(Scanner scanner) {
while (true) {
try {
System.out.print("Enter an integer: ");
return Integer.parseInt(scanner.nextLine());
} catch (NumberFormatException e) {
System.out.println("Invalid input. Please enter a valid integer.");
}
}
}
}
In this code snippet, we focus on addressing the issue of nextLine
skips in Java Scanner
by incorporating the parseInt()
method.
We initialize a Scanner
object named scanner
to facilitate input reading from the standard input stream (System.in
).
For handling integer input, we introduce the readInt
method. Inside this method, we use a try-catch
block to handle potential NumberFormatExceptions
, which may occur if the input is not a valid integer.
Within the try
block, we prompt the user to enter an integer using scanner.nextLine()
and then convert the input to an integer using Integer.parseInt()
.
In the case of a NumberFormatException
, the catch
block is executed, informing the user of the invalid input and prompting them to enter a valid integer.
The while (true)
loop ensures that the user is continuously prompted until a valid integer is provided.
After successfully obtaining the integer input, we proceed to prompt the user for a string input using scanner.nextLine()
.
Finally, the program displays the results, showcasing the effectiveness of using parseInt()
to prevent nextLine
skips.
Upon running this program and entering an integer followed by a string, the output will demonstrate the effectiveness of using parseInt()
to fix nextLine
skips:
The program now gracefully handles integer input using Integer.parseInt()
, effectively resolving the nextLine
skips issue.
Using an Extra nextLine()
Statement
By strategically incorporating an extra nextLine()
statement, we can mitigate the challenges associated with nextLine
skips in Java Scanner. This simple yet powerful method ensures that newline characters are consumed appropriately, providing a reliable solution for handling user input in a way that is both effective and efficient.
Let’s delve into a comprehensive example that demonstrates how to fix Java Scanner nextLine skips
using an extra nextLine()
statement:
import java.util.Scanner;
public class ScannerFixExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter an integer: ");
int intValue = scanner.nextInt();
// Extra nextLine() statement to consume the newline character
scanner.nextLine();
System.out.print("Enter a string: ");
String stringValue = scanner.nextLine();
System.out.println("Integer: " + intValue);
System.out.println("String: " + stringValue);
}
}
In this code snippet, we employ a strategic use of an extra nextLine()
statement to absorb the newline character left unconsumed in the input buffer.
We initiate a Scanner
object named scanner
to facilitate input reading from the standard input stream (System.in
).
Prompt the user to enter an integer using nextInt()
, which captures the integer value but leaves a newline character in the input buffer.
Now, strategically insert an extra nextLine()
statement. This extra statement is essential as it consumes the leftover newline character from the previous input, effectively preventing any subsequent nextLine
skips.
Proceed to prompt the user for a string input using nextLine()
.
If you run this program and enter an integer followed by a string, the output will demonstrate the effectiveness of the extra nextLine()
statement:
This strategic use of the extra nextLine()
statement proves effective in mitigating the challenges associated with nextLine
skips, ensuring a smooth and reliable user input experience in Java programs.
Using trim()
Method
By incorporating the trim()
method, we can successfully address the issue of nextLine
skips in Java Scanner
. This elegant solution ensures that leading or trailing whitespaces are removed from the user’s input, providing a clean and consistent experience when handling both integer and string inputs in Java programs.
Let’s delve into a practical example demonstrating how to fix Java Scanner nextLine skips
using the trim()
method:
import java.util.Scanner;
public class ScannerFixExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter an integer: ");
int intValue = scanner.nextInt();
// Consume the newline character
scanner.nextLine();
System.out.print("Enter a string: ");
String stringValue = scanner.nextLine().trim();
System.out.println("Integer: " + intValue);
System.out.println("String: " + stringValue);
}
}
In this code snippet, we tackle the issue of nextLine
skips by utilizing the trim()
method to remove leading or trailing whitespaces.
We initialize a Scanner
object named scanner
to facilitate input reading from the standard input stream (System.in
).
Prompt the user to enter an integer using nextInt()
. However, keep in mind that this method does not consume the newline character, which is left in the input buffer.
To address this, we consume the newline character using scanner.nextLine()
.
Now, prompt the user for a string input using nextLine()
. This time, we immediately apply the trim()
method to the obtained string. The trim()
method removes any leading or trailing whitespaces, ensuring that the input is clean and free from any unwanted characters.
Upon running this program and entering an integer followed by a string, the output will demonstrate the effectiveness of using the trim()
method to fix nextLine
skips:
The program displays the results, showcasing the effectiveness of the trim()
method in preventing nextLine
skips and ensuring a seamless user input experience in Java programs.
Conclusion
Java Scanner nextLine skips
often occur due to newline characters left unconsumed in the input buffer, creating unexpected behavior during input reading. This article explored practical solutions to address this issue.
We discussed methods such as using an extra nextLine()
statement, employing Integer.parseInt()
for integer input, and leveraging the trim()
method to handle leading or trailing whitespaces. By understanding and implementing these techniques, developers can ensure a more robust and predictable user input experience, ultimately preventing nextLine
skips and enhancing the reliability of Java Scanner
in handling various input scenarios.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack