修复 Java Scanner NextLine Skips 错误
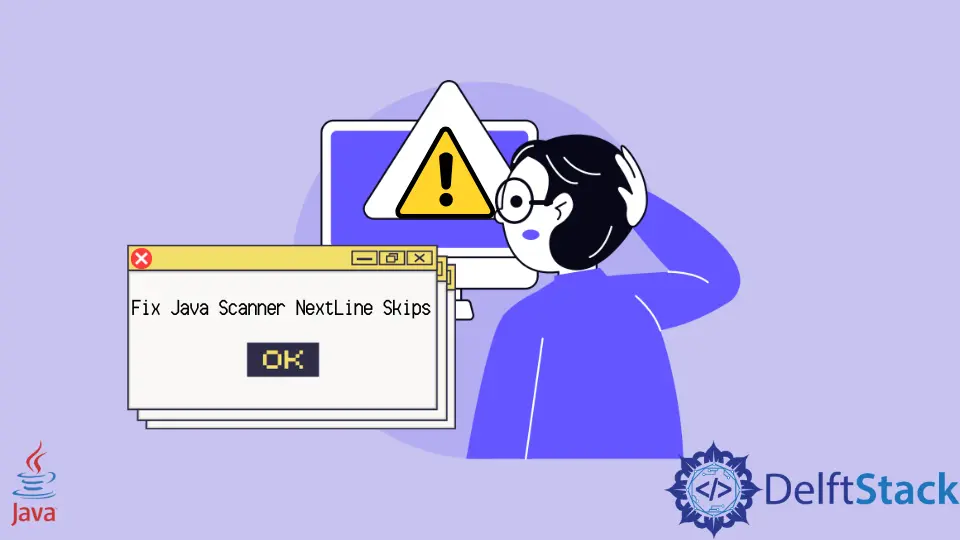
在 Java 中的 next()
或 nextInt()
方法之后使用 nextLine()
方法时,有时 nextLine()
方法会跳过要读取的当前输入并转到下一个输入。本文将讨论为什么会发生这种情况以及如何解决此问题。
在我们研究 nextLine()
方法的问题之前,让我们首先了解 nextLine()
方法的工作原理。此方法属于 java.util.Scanner
类。
此函数打印整行,除了末尾的行分隔符。它搜索输入以寻找行分隔符。如果没有找到行分隔符,它可能会在搜索要跳过的行的同时搜索所有输入。
此 Scanner 方法越过当前行。如果有任何输入被跳过,它会返回它。按照此链接参考该方法的文档。
语法:
public String nextLine()
请注意,此函数不接受任何参数并返回跳过的行。看看下面的例子,看看它是如何工作的。
// To demonstrate the working of nextLine() method
import java.util.*;
public class Example {
public static void main(String[] args) {
String a = "Java \n is \n intriguing";
// create a new scanner
Scanner sc = new Scanner(a);
// print the next line
System.out.println(sc.nextLine());
// print the next line again
System.out.println(sc.nextLine());
// print the next line again
System.out.println(sc.nextLine());
}
}
输出:
Java
is
intriguing
Java 中 nextLine()
方法的主要用途是读取带有空格的字符串输入。
请注意,我们也可以使用 next()
方法输入一个字符串,但它只读取它遇到的第一个空格。此外,next()
方法在输入后将光标放在同一行。
然而,nextLine()
方法在读取输入后将光标置于下一行。看下面的例子。
import java.util.*;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// Try reading a string with space using next()
System.out.println("Enter the name of a movie");
String name = sc.next();
// Done to bring the cursor to next line
sc.nextLine();
// Re-reading the string using nextLine()
System.out.println("Re-enter this name");
String rename = sc.nextLine();
System.out.println();
System.out.println("Here is the output:");
// printing the output
System.out.println(name);
System.out.println(rename);
}
}
输出:
Enter the name of a movie
Harry Potter
Re-enter this name
Harry Potter
Here is the output:
Harry
Harry Potter
请注意,next()
方法仅读取空格前的名字并忽略其余部分。然而,nextLine()
方法读取带有空格的整个字符串。
nextLine()
方法的问题
要了解此问题,请查看以下示例。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
int number = sc.nextInt();
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
输出:
Input the Roll Number:
1
Input your full name:
Input your branch:
Computer Science
Roll No: 1
Name:
Branch: Computer Science
但所需的输出是这样的:
Input the Roll Number : 1 Input your full name : Harry Potter Input your branch
: Computer Science Roll No : 1 Name : Harry Potter Branch : Computer Science
请注意,nextLine()
方法跳过 Name
的输入,而是转到分支的下一个输入。
为什么会出现这个问题
这是因为当 Scanner 类的 nextInt()
方法读取卷号时,它返回值 1
。该值存储在变量编号中。
但是读取卷号后光标不会转到下一行。它在 1
之后仍然存在。像这样:
1_ // the cursor remains here only
因此,当我们使用 nextLine()
方法读取名称时,它会从光标的当前位置开始读取输入。所以在 1
之后,下一行就是新行本身。 \n
字符表示这个新行。因此,名称只是\n
。
如何解决 Java Scanner NextLine 跳过的问题
有两种方法可以解决此问题。
使用 Java Integer.parseInt()
方法
parseInt()
方法属于 java.lang
包的 Integer 类,返回某个字符串的原始数据类型。这些方法主要有两种:
Integer.parseInt(String s)
Integer.parseInt(String s, int radix)
要使用 nextLine()
跳过,我们将使用第一种方法 - Integer.parseInt(String s)
。这会将整数的完整行读取为字符串。稍后,它将其转换为整数。
语法:
int value = Integer.parseInt(sc.nextLine());
让我们使用 Java Integer.parseInt()
方法实现我们上面使用的相同代码并纠正问题。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
// Using the parseInt() method this time
int number = Integer.parseInt(sc.nextLine());
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
输出:
Input the Roll Number:
1
Input your full name:
Harry Potter
Input your branch:
Computer Science
Roll No: 1
Name: Harry Potter
Branch: Computer Science
这次代码运行良好。但是我们不能将它用于 Byte character(Byte.parseByte(sc.nextLine()))
之后的输入字符串。
在这种情况下,我们可以使用第二种方法。这是文档的链接以获取更多信息。
使用额外的 nextLine()
语句
这会消耗剩余的新行。看例子。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
int number = sc.nextInt();
// An extra nextLine() to consume the leftover line
sc.nextLine();
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
输出:
Input the Roll Number:
1
Input your full name:
Harry Potter
Input your branch:
Computer Science
Roll No: 1
Name: Harry Potter
Branch: Computer Science
相关文章 - Java Error
- Java 中的 reached end of the file while parsing 错误
- 防止 Java 中的 Orphaned Case 错误
- 修复 Java 中的 NoSuchElementException 错误
- 修复 Java 中缺少返回语句的错误类型
- Java 中的 Class is not abstract and does not override abstract method 错误