Resuelto: Java Scanner NextLine Skips
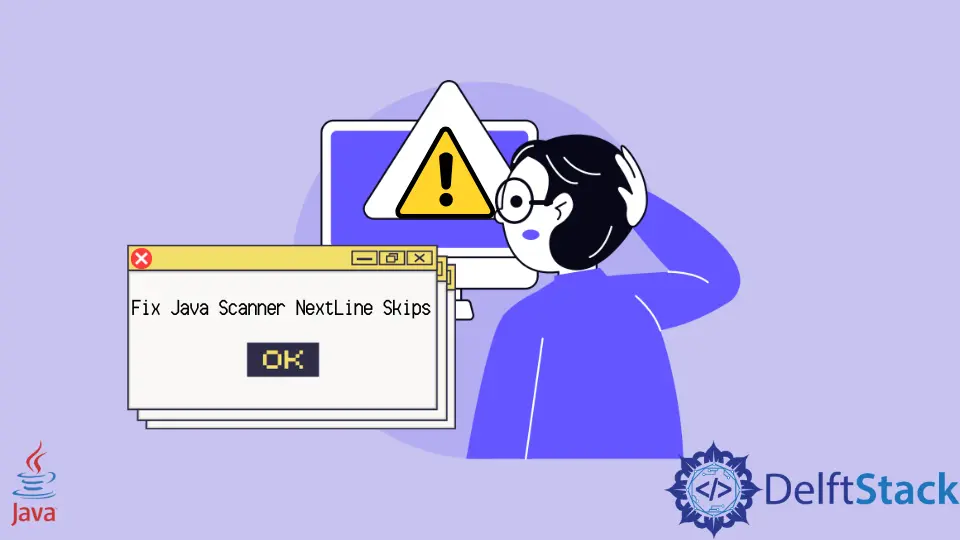
Al usar el método nextLine()
después del método next()
o nextInt()
en Java, a veces el método nextLine()
salta la entrada actual para leer y pasa a la siguiente entrada. Este artículo discutirá por qué sucede esto y cómo solucionar este problema.
Antes de analizar el problema con el método nextLine()
, primero comprendamos el funcionamiento del método nextLine()
. Este método pertenece a la clase java.util.Scanner
.
Esta función imprime la línea completa excepto el separador de línea al final. Busca una entrada buscando un separador de línea. Si no se encuentra un separador de línea, puede buscar toda la entrada mientras busca la línea para omitir.
Este método de escáner va más allá de la línea actual. Si hay alguna entrada que se omitió, la devuelve. Siga este enlace para consultar la documentación del método.
Sintaxis:
public String nextLine()
Tenga en cuenta que esta función no toma ningún parámetro y devuelve la línea omitida. Mira el siguiente ejemplo para ver cómo funciona.
// To demonstrate the working of nextLine() method
import java.util.*;
public class Example {
public static void main(String[] args) {
String a = "Java \n is \n intriguing";
// create a new scanner
Scanner sc = new Scanner(a);
// print the next line
System.out.println(sc.nextLine());
// print the next line again
System.out.println(sc.nextLine());
// print the next line again
System.out.println(sc.nextLine());
}
}
Producción :
Java
is
intriguing
El uso principal del método nextLine()
en Java es leer una entrada de cadena con espacio.
Tenga en cuenta que también podemos usar el método next()
para ingresar una cadena, pero solo lee hasta el primer espacio que encuentra. Además, el método next()
coloca el cursor en la misma línea después de tomar la entrada.
Sin embargo, el método nextLine()
coloca el cursor en la siguiente línea después de leer la entrada. Mira el siguiente ejemplo.
import java.util.*;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// Try reading a string with space using next()
System.out.println("Enter the name of a movie");
String name = sc.next();
// Done to bring the cursor to next line
sc.nextLine();
// Re-reading the string using nextLine()
System.out.println("Re-enter this name");
String rename = sc.nextLine();
System.out.println();
System.out.println("Here is the output:");
// printing the output
System.out.println(name);
System.out.println(rename);
}
}
Producción :
Enter the name of a movie
Harry Potter
Re-enter this name
Harry Potter
Here is the output:
Harry
Harry Potter
Tenga en cuenta que el método next()
solo lee el primer nombre antes del espacio e ignora el resto. Sin embargo, el método nextLine()
lee la cadena completa con espacio.
El problema con el método nextLine()
Para comprender este problema, observe el siguiente ejemplo.
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
int number = sc.nextInt();
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
Producción :
Input the Roll Number:
1
Input your full name:
Input your branch:
Computer Science
Roll No: 1
Name:
Branch: Computer Science
Pero la salida requerida es esta:
Input the Roll Number : 1 Input your full name : Harry Potter Input your branch
: Computer Science Roll No : 1 Name : Harry Potter Branch : Computer Science
Observe que el método nextLine()
omite la entrada de Name
y en su lugar va a la siguiente entrada de la rama.
¿Por qué ocurre este problema?
Esto sucede porque cuando el método nextInt()
de la clase Scanner lee el número de rollo, devuelve el valor 1
. Este valor se almacena en el número de variable.
Pero el cursor no pasa a la siguiente línea después de leer el número de rollo. Queda justo después del 1
. Como esto:
1_ // the cursor remains here only
Por lo tanto, cuando usamos el método nextLine()
para leer el nombre, comienza a leer la entrada desde la posición actual del cursor. Entonces, después de 1
, la siguiente línea no es más que la nueva línea en sí. El carácter \n
representa esta nueva línea. Por lo tanto, el nombre es simplemente \n
.
Cómo solucionar este problema de los saltos NextLine de Java Scanner
Hay dos formas en las que podemos solucionar este problema.
Usando el método Java Integer.parseInt()
El método parseInt()
pertenece a la clase Integer del paquete java.lang
y devuelve el tipo de dato primitivo de una determinada cadena. Estos métodos son de dos tipos principalmente:
Integer.parseInt(String s)
Integer.parseInt(String s, int radix)
Para trabajar con los saltos nextLine()
, utilizaremos el primer método: Integer.parseInt(String s)
. Esto lee la línea completa del Integer como String. Más tarde, lo convierte en un número entero.
Sintaxis:
int value = Integer.parseInt(sc.nextLine());
Implementemos el mismo código que usamos anteriormente con el método Java Integer.parseInt()
y rectifiquemos el problema.
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
// Using the parseInt() method this time
int number = Integer.parseInt(sc.nextLine());
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
Producción :
Input the Roll Number:
1
Input your full name:
Harry Potter
Input your branch:
Computer Science
Roll No: 1
Name: Harry Potter
Branch: Computer Science
Esta vez el código funciona bien. Pero no podemos usar esto para la cadena de entrada después de Byte character(Byte.parseByte(sc.nextLine()))
.
Podemos usar el segundo método en ese caso. Aquí está el enlace a documentación para obtener más información.
Uso de una instrucción extra nextLine()
Esto consume la nueva línea sobrante. Mira el ejemplo.
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
int number = sc.nextInt();
// An extra nextLine() to consume the leftover line
sc.nextLine();
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
Producción :
Input the Roll Number:
1
Input your full name:
Harry Potter
Input your branch:
Computer Science
Roll No: 1
Name: Harry Potter
Branch: Computer Science
Artículo relacionado - Java Error
- Arreglar Java fue iniciado por el código de salida devuelto = 1
- Arreglar JAVA_HOME no se puede determinar a partir del error de registro en R
- Arreglar java.io.IOException: No queda espacio en el dispositivo en Java
- Arreglar Java.IO.NotSerializableException en Java
- Arreglar Java.Lang.IllegalStateException de Android: no se pudo ejecutar el método de la actividad
- Arreglar Java.Lang.NoClassDefFoundError: No se pudo inicializar el error de clase