修復 Java Scanner NextLine Skips 錯誤
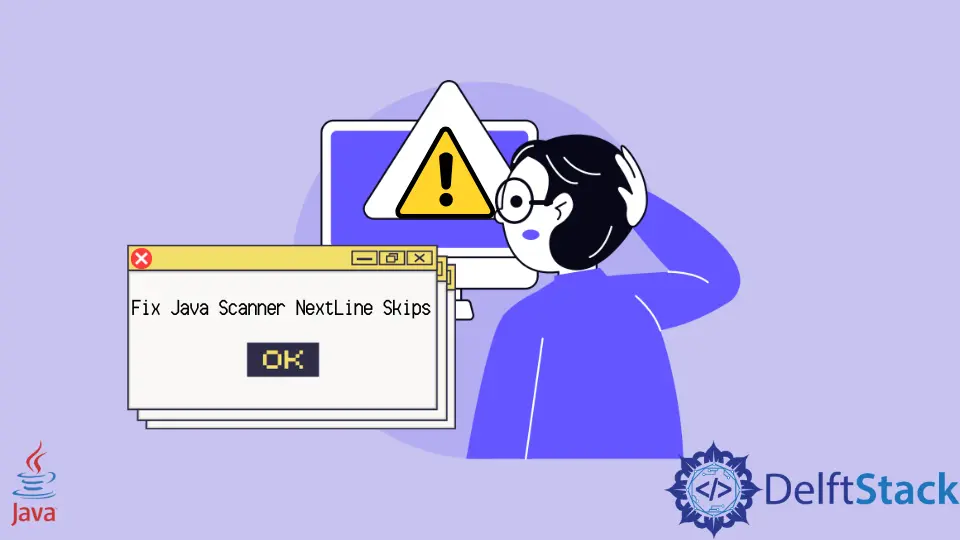
在 Java 中的 next()
或 nextInt()
方法之後使用 nextLine()
方法時,有時 nextLine()
方法會跳過要讀取的當前輸入並轉到下一個輸入。本文將討論為什麼會發生這種情況以及如何解決此問題。
在我們研究 nextLine()
方法的問題之前,讓我們首先了解 nextLine()
方法的工作原理。此方法屬於 java.util.Scanner
類。
此函式列印整行,除了末尾的行分隔符。它搜尋輸入以尋找行分隔符。如果沒有找到行分隔符,它可能會在搜尋要跳過的行的同時搜尋所有輸入。
此 Scanner 方法越過當前行。如果有任何輸入被跳過,它會返回它。按照此連結參考該方法的文件。
語法:
public String nextLine()
請注意,此函式不接受任何引數並返回跳過的行。看看下面的例子,看看它是如何工作的。
// To demonstrate the working of nextLine() method
import java.util.*;
public class Example {
public static void main(String[] args) {
String a = "Java \n is \n intriguing";
// create a new scanner
Scanner sc = new Scanner(a);
// print the next line
System.out.println(sc.nextLine());
// print the next line again
System.out.println(sc.nextLine());
// print the next line again
System.out.println(sc.nextLine());
}
}
輸出:
Java
is
intriguing
Java 中 nextLine()
方法的主要用途是讀取帶有空格的字串輸入。
請注意,我們也可以使用 next()
方法輸入一個字串,但它只讀取它遇到的第一個空格。此外,next()
方法在輸入後將游標放在同一行。
然而,nextLine()
方法在讀取輸入後將游標置於下一行。看下面的例子。
import java.util.*;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// Try reading a string with space using next()
System.out.println("Enter the name of a movie");
String name = sc.next();
// Done to bring the cursor to next line
sc.nextLine();
// Re-reading the string using nextLine()
System.out.println("Re-enter this name");
String rename = sc.nextLine();
System.out.println();
System.out.println("Here is the output:");
// printing the output
System.out.println(name);
System.out.println(rename);
}
}
輸出:
Enter the name of a movie
Harry Potter
Re-enter this name
Harry Potter
Here is the output:
Harry
Harry Potter
請注意,next()
方法僅讀取空格前的名字並忽略其餘部分。然而,nextLine()
方法讀取帶有空格的整個字串。
nextLine()
方法的問題
要了解此問題,請檢視以下示例。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
int number = sc.nextInt();
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
輸出:
Input the Roll Number:
1
Input your full name:
Input your branch:
Computer Science
Roll No: 1
Name:
Branch: Computer Science
但所需的輸出是這樣的:
Input the Roll Number : 1 Input your full name : Harry Potter Input your branch
: Computer Science Roll No : 1 Name : Harry Potter Branch : Computer Science
請注意,nextLine()
方法跳過 Name
的輸入,而是轉到分支的下一個輸入。
為什麼會出現這個問題
這是因為當 Scanner 類的 nextInt()
方法讀取卷號時,它返回值 1
。該值儲存在變數編號中。
但是讀取卷號後游標不會轉到下一行。它在 1
之後仍然存在。像這樣:
1_ // the cursor remains here only
因此,當我們使用 nextLine()
方法讀取名稱時,它會從游標的當前位置開始讀取輸入。所以在 1
之後,下一行就是新行本身。 \n
字元表示這個新行。因此,名稱只是\n
。
如何解決 Java Scanner NextLine 跳過的問題
有兩種方法可以解決此問題。
使用 Java Integer.parseInt()
方法
parseInt()
方法屬於 java.lang
包的 Integer 類,返回某個字串的原始資料型別。這些方法主要有兩種:
Integer.parseInt(String s)
Integer.parseInt(String s, int radix)
要使用 nextLine()
跳過,我們將使用第一種方法 - Integer.parseInt(String s)
。這會將整數的完整行讀取為字串。稍後,它將其轉換為整數。
語法:
int value = Integer.parseInt(sc.nextLine());
讓我們使用 Java Integer.parseInt()
方法實現我們上面使用的相同程式碼並糾正問題。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
// Using the parseInt() method this time
int number = Integer.parseInt(sc.nextLine());
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
輸出:
Input the Roll Number:
1
Input your full name:
Harry Potter
Input your branch:
Computer Science
Roll No: 1
Name: Harry Potter
Branch: Computer Science
這次程式碼執行良好。但是我們不能將它用於 Byte character(Byte.parseByte(sc.nextLine()))
之後的輸入字串。
在這種情況下,我們可以使用第二種方法。這是文件的連結以獲取更多資訊。
使用額外的 nextLine()
語句
這會消耗剩餘的新行。看例子。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
int number = sc.nextInt();
// An extra nextLine() to consume the leftover line
sc.nextLine();
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
輸出:
Input the Roll Number:
1
Input your full name:
Harry Potter
Input your branch:
Computer Science
Roll No: 1
Name: Harry Potter
Branch: Computer Science
相關文章 - Java Error
- Java 中的 reached end of the file while parsing 錯誤
- 防止 Java 中的 Orphaned Case 錯誤
- 修復 Java 中的 NoSuchElementException 錯誤
- 修復 Java 中缺少返回語句的錯誤型別
- Java 中的 Class is not abstract and does not override abstract method 錯誤