解決済み:Java Scanner NextLine Skips
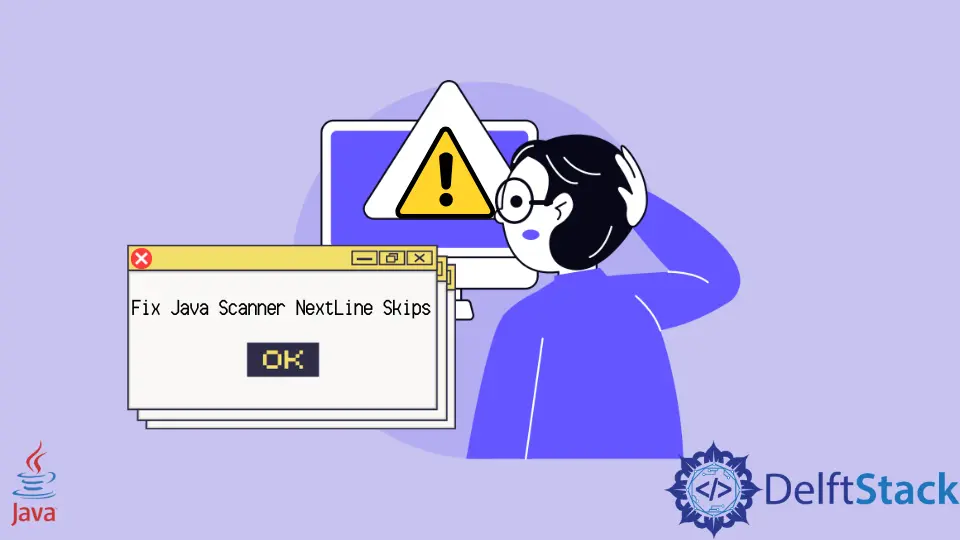
Java で next()
または nextInt()
メソッドの後に nextLine()
メソッドを使用しているときに、nextLine()
メソッドが現在の入力をスキップして読み取られ、次の入力に進むことがあります。この記事では、これが発生する理由とこの問題を修正する方法について説明します。
nextLine()
メソッドの問題を調べる前に、まず nextLine()
メソッドの動作を理解しましょう。このメソッドは、java.util.Scanner
クラスに属しています。
この関数は、最後の行区切り文字を除く行全体を出力します。行区切り文字を探して入力を検索します。行区切り文字が見つからない場合は、スキップする行を検索しているときにすべての入力を検索する場合があります。
このスキャナー方式は、現在の行を超えます。スキップされた入力がある場合は、それを返します。このリンクに従って、メソッドのドキュメントを参照してください。
構文:
public String nextLine()
この関数はパラメーターを受け取らず、スキップされた行を返すことに注意してください。以下の例を見て、どのように機能するかを確認してください。
// To demonstrate the working of nextLine() method
import java.util.*;
public class Example {
public static void main(String[] args) {
String a = "Java \n is \n intriguing";
// create a new scanner
Scanner sc = new Scanner(a);
// print the next line
System.out.println(sc.nextLine());
// print the next line again
System.out.println(sc.nextLine());
// print the next line again
System.out.println(sc.nextLine());
}
}
出力:
Java
is
intriguing
Java での nextLine()
メソッドの主な用途は、スペースを含む文字列入力を読み取ることです。
next()
メソッドを使用して文字列を入力することもできますが、最初に検出されたスペースまでしか読み取れないことに注意してください。また、next()
メソッドは、入力を受け取った後、カーソルを同じ行に置きます。
ただし、nextLine()
メソッドは、入力を読み取った後、カーソルを次の行に置きます。以下の例を見てください。
import java.util.*;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// Try reading a string with space using next()
System.out.println("Enter the name of a movie");
String name = sc.next();
// Done to bring the cursor to next line
sc.nextLine();
// Re-reading the string using nextLine()
System.out.println("Re-enter this name");
String rename = sc.nextLine();
System.out.println();
System.out.println("Here is the output:");
// printing the output
System.out.println(name);
System.out.println(rename);
}
}
出力:
Enter the name of a movie
Harry Potter
Re-enter this name
Harry Potter
Here is the output:
Harry
Harry Potter
next()
メソッドはスペースの前の名のみを読み取り、残りは無視することに注意してください。ただし、nextLine()
メソッドは、スペースを含む文字列全体を読み取ります。
nextLine()
メソッドの問題
この問題を理解するには、以下の例を見てください。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
int number = sc.nextInt();
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
出力:
Input the Roll Number:
1
Input your full name:
Input your branch:
Computer Science
Roll No: 1
Name:
Branch: Computer Science
ただし、必要な出力は次のとおりです。
Input the Roll Number : 1 Input your full name : Harry Potter Input your branch
: Computer Science Roll No : 1 Name : Harry Potter Branch : Computer Science
nextLine()
メソッドは Name
の入力をスキップし、代わりにブランチの次の入力に進むことに注意してください。
この問題が発生する理由
これは、Scanner クラスの nextInt()
メソッドがロール番号を読み取るときに、値 1
を返すために発生します。この値は変数番号に格納されます。
ただし、ロール番号を読み取った後、カーソルは次の行に移動しません。1
の直後に残ります。このような:
1_ // the cursor remains here only
したがって、nextLine()
メソッドを使用して名前を読み取ると、カーソルの現在の位置から入力の読み取りが開始されます。したがって、1
の後、次の行は新しい行自体に他なりません。\n
文字はこの新しい行を表します。したがって、名前は単に\n
です。
Java スキャナーNextLine スキップのこの問題を修正する方法
この問題を修正する方法は 2つあります。
Java Integer.parseInt()
メソッドの使用
parseInt()
メソッドは、java.lang
パッケージの Integer クラスに属し、特定の文字列のプリミティブデータ型を返します。これらのメソッドには、主に次の 2つのタイプがあります。
Integer.parseInt(String s)
Integer.parseInt(String s, int radix)
nextLine()
スキップを操作するには、最初のメソッドである Integer.parseInt(String s)
を使用します。これにより、整数の完全な行が文字列として読み取られます。後で、それを整数に変換します。
構文:
int value = Integer.parseInt(sc.nextLine());
上記で使用したのと同じコードを Java Integer.parseInt()
メソッドで実装し、問題を修正しましょう。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
// Using the parseInt() method this time
int number = Integer.parseInt(sc.nextLine());
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
出力:
Input the Roll Number:
1
Input your full name:
Harry Potter
Input your branch:
Computer Science
Roll No: 1
Name: Harry Potter
Branch: Computer Science
今回はコードは正常に実行されます。ただし、Byte character(Byte.parseByte(sc.nextLine()))
の後の入力文字列にはこれを使用できません。
その場合、2 番目の方法を使用できます。詳細については、ドキュメントへのリンクをご覧ください。
追加の nextLine()
ステートメントの使用
これにより、残りの新しい行が消費されます。例を見てください。
import java.util.Scanner;
public class Demo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// This part takes the input
System.out.println("Input the Roll Number:");
int number = sc.nextInt();
// An extra nextLine() to consume the leftover line
sc.nextLine();
System.out.println("Input your full name:");
String name = sc.nextLine();
System.out.println("Input your branch:");
String branch = sc.nextLine();
// This part prints the values
System.out.println("Roll No: " + number);
System.out.println("Name: " + name);
System.out.println("Branch: " + branch);
}
}
出力:
Input the Roll Number:
1
Input your full name:
Harry Potter
Input your branch:
Computer Science
Roll No: 1
Name: Harry Potter
Branch: Computer Science
関連記事 - Java Error
- $' ': Java の Command Not Found エラーを修正
- Class X Is Public を X.java という名前のファイルで宣言する必要がある問題を修正
- Eclipse エラー: 選択を起動できません
- Eclipse で Java ランタイム環境を続行するにはメモリが不足しています
- Eclipse の`Java 仮想マシンが見つかりませんでした`というエラーを修正する
- IntelliJ IDEA のスレッド メイン Java.Lang.ClassNotFoundException での例外