Java 中的 reached end of the file while parsing 错误
-
reached end of the file while parsing
-Java 中缺少类大括号 -
reached end of the file while parsing
-Java 中缺少 if 大括号 -
reached end of the file while parsing
-Java 中缺少循环大括号 -
reached end of the file while parsing
-Java 中缺少方法大括号 -
在 Java 中避免出现
reached end of the file while parsing
的错误
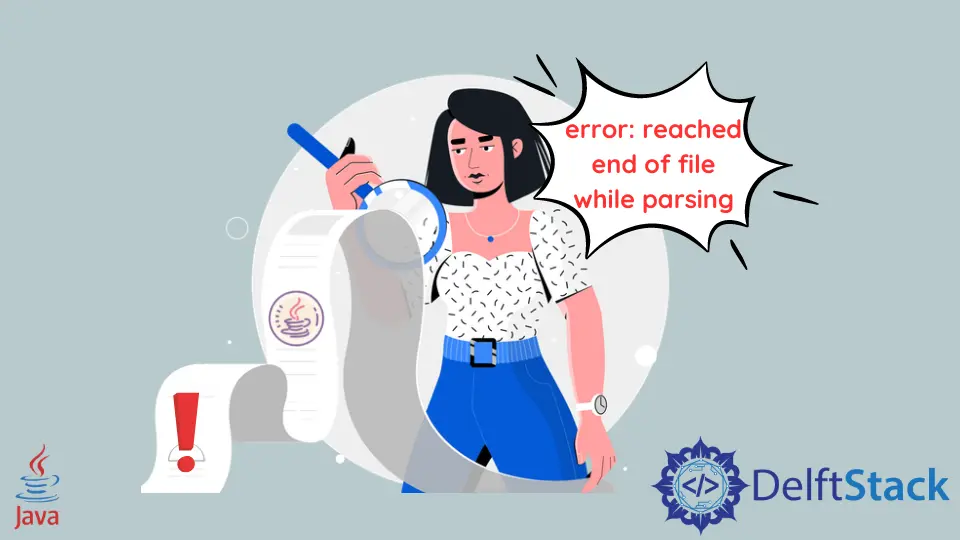
本教程介绍了在 Java 的代码编译过程中出现的 reached end of the file while parsing
错误。
reached end of the file while parsing
错误是编译时错误。当代码块缺少大括号或代码中有额外的大括号时。
本教程将介绍此错误如何发生以及如何解决的不同示例。reached end of file while parsing
错误是编译器告诉它已到达文件末尾但未找到其末尾的方式。
在 Java 中,每个左大括号 ({
) 都需要一个右大括号 (}
)。如果我们没有在需要的地方放置大括号,我们的代码将无法正常工作,并且会出现错误。
reached end of the file while parsing
-Java 中缺少类大括号
在下面的示例中,我们错过了为类添加右大括号。
当我们编译这段代码时,它会向控制台返回一个错误。如果大括号的数量少于所需的数量,则会发生 reached end of the file while parsing
错误。
看下面的代码:
public class MyClass {
public static void main(String args[]) {
print_something();
}
输出:
MyClass.java:6: error: reached end of file while parsing
}
^
1 error
上面的代码中缺少 MyClass
的右大括号。我们可以通过在代码末尾再添加一个大括号来解决这个问题。
看下面修改后的代码:
public class MyClass {
static void print_something() {
System.out.println("hello world");
}
public static void main(String args[]) {
print_something();
}
}
输出:
hello world
让我们看一下可能发生此错误的示例。
reached end of the file while parsing
-Java 中缺少 if 大括号
if
块在下面的代码中缺少右大括号。这会导致在 Java 代码编译期间出现 reached end of the file while parsing
错误。
public class MyClass {
public static void main(String args[]) {
int x = 38;
if (x > 90) {
// do something
System.out.println("Greater than 90");
}
}
输出:
MyClass.java:8: error: reached end of file while parsing
}
^
1 error
我们可以通过在适当的位置(在 if
块的末尾)添加大括号来解决此错误。看下面的代码:
public class MyClass {
public static void main(String args[]) {
int x = 38;
if (x > 90) {
// do something
System.out.println("Greater than 90");
} // this brace was missing
}
}
上面的代码编译没有给出任何错误。
输出:
Greater than 90
reached end of the file while parsing
-Java 中缺少循环大括号
缺少的大括号可能来自 while
或 for
循环。在下面的代码中,while
循环块缺少所需的右大括号,导致编译失败。
请参见下面的示例。
public class MyClass {
public static void main(String args[]) {
int x = 38;
while (x > 90) {
// do something
System.out.println("Greater than 90");
x--;
}
}
输出:
MyClass.java:10: error: reached end of file while parsing
}
^
1 error
我们可以通过将大括号放在所需位置(在 while
循环的末尾)来解决此错误。看下面修改后的代码:
public class MyClass {
public static void main(String args[]) {
int x = 38;
while (x > 90) {
// do something
System.out.println("Greater than 90");
x--;
} // This brace was missing
}
}
上面的代码编译没有给出任何错误。
输出:
Greater than 90
reached end of the file while parsing
-Java 中缺少方法大括号
在这种情况下,我们定义了一个缺少右大括号的方法,如果我们编译这段代码,我们会得到一个编译器错误。看看下面的代码。
public class MyClass {
public static void main(String args[]) {
customFunction();
}
static void customFunction() {
System.out.println("Inside the function");
}
输出:
MyClass.java:9: error: reached end of file while parsing
}
^
1 error
我们可以通过将大括号放在所需的位置(在函数体的末尾)来解决这个错误。看下面修改后的代码:
public class MyClass {
public static void main(String args[]) {
customFunction();
}
static void customFunction() {
System.out.println("Inside the function");
}
}
输出:
Inside the function
在 Java 中避免出现 reached end of the file while parsing
的错误
这个错误很常见,也很容易避免。
为了避免这个错误,我们应该正确缩进我们的代码。这将使我们能够轻松地找到丢失的右大括号。
我们还可以使用代码编辑器自动格式化我们的代码并将每个左大括号与其右大括号匹配。这将帮助我们找到右大括号缺失的地方。