Fix the Reach End of File While Parsing Error in Java
-
The
Reached End of File While Parsing
Error -
The
Reached End of File While Parsing
Error: Causes and Solutions - Conclusion
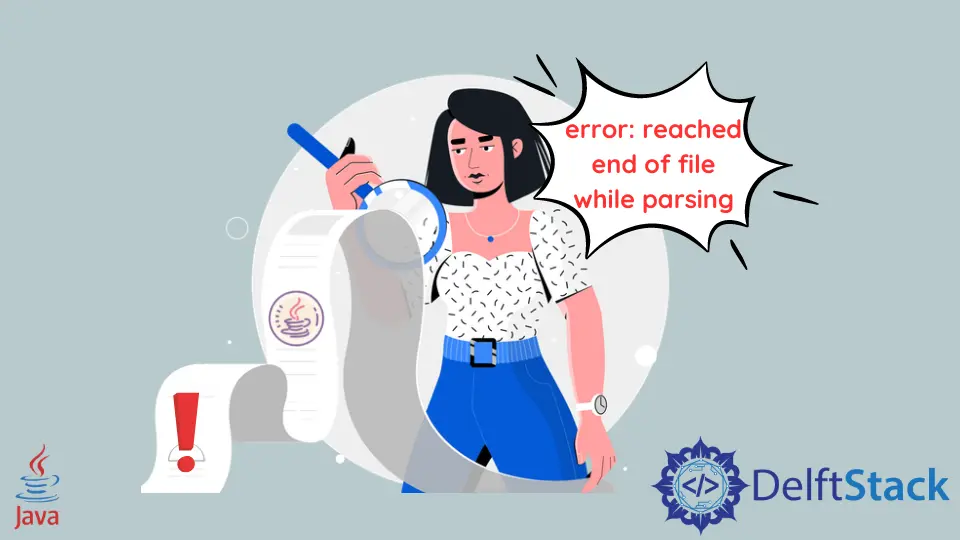
The article explores the causes and solutions of the Reached End of File While Parsing
error in Java, providing insights into resolving this common issue during code compilation.
The Reached End of File While Parsing
Error
The Reached End of File While Parsing
error in Java occurs when the compiler is parsing (analyzing and interpreting) your code and unexpectedly encounters the end of the file (EOF). This usually happens because the code is incomplete or has syntax errors, preventing the compiler from successfully understanding and processing the entire program.
In simple terms, it’s like the compiler saying, “I expected more code, but the file ended abruptly”.
To resolve this error, you need to check for missing or incorrect syntax in your code.
The Reached End of File While Parsing
Error: Causes and Solutions
The reached end of file while parsing
error in Java typically occurs when the compiler encounters an unexpected end-of-file (EOF) while parsing the source code. This error can have various causes, and here are some common ones, along with possible solutions:
Issue | Cause | Solution |
---|---|---|
Missing Parentheses, Braces, or Brackets | An opening parenthesis, brace, or bracket might not have a corresponding closing one. | Check your code for missing or mismatched parentheses, braces, or brackets. Ensure that each opening symbol has a corresponding closing symbol. |
Incomplete Statements | A statement might be incomplete, missing a semicolon, or lacking some required elements. | Double-check your statements for completeness. Ensure that each statement is properly terminated with a semicolon, and make sure all required elements are present. |
Incomplete Class or Method | A class or method declaration might be incomplete, missing curly braces or other necessary components. | Verify that all class and method declarations are complete with the correct syntax. Ensure that each opening curly brace has a corresponding closing one. |
Incorrect Nesting of Braces | Incorrect nesting of braces can lead to the parser reaching the end of the file unexpectedly. | Review your code for proper nesting of braces. Ensure that opening and closing braces are properly matched and nested. |
Missing main Method |
In a Java application, the main method is the entry point. If it is missing or incorrectly defined, the parser may encounter EOF prematurely. |
Make sure your Java program has a properly defined main method with the correct signature: public static void main(String[] args) . |
Missing import Statements |
If your code relies on external classes and the necessary import statements are missing, it can lead to parsing errors. |
Add import statements for the classes you are using in your code. |
Incorrectly Placed or Misused Comments | Comments that are not properly closed or are placed inappropriately can cause parsing issues. | Review your comments and ensure that they are correctly placed and closed. |
Incorrect Package Declarations | If the package declaration is incorrect or missing, it can lead to parsing errors. | Verify that your package declaration is correct and matches the directory structure of your Java source files. |
Encoding Issues | If there are encoding issues in your source code file, it might lead to parsing errors. | Ensure that your source code file is saved with the correct encoding (e.g., UTF-8). |
File Corruption | The source code file may be corrupted. | Try reopening the file or recovering it from a backup. |
Remember to carefully review your code, paying attention to syntax, proper nesting, and correct structure to identify and resolve parsing errors. Additionally, using an Integrated Development Environment (IDE) can help catch these errors early and provide helpful suggestions for fixing them.
We will explore common examples of errors and provide solutions to resolve them effectively in Java.
Missing Parentheses, Braces, or Brackets
In Java programming, encountering the Reached End of File While Parsing
error can be frustrating, especially when caused by missing parentheses, braces, or brackets. These symbols are essential for defining the structure of code blocks, and their absence can lead to parsing errors.
Code Example:
public class ParsingErrorExample {
public static void main(String[] args) {
// Missing closing parenthesis
// int result = addNumbers(5, 10; // Error: missing closing parenthesis
// Corrected method call
int correctedResult = addNumbers(5, 10);
System.out.println("Corrected Result: " + correctedResult);
}
// Corrected method with complete parentheses
private static int addNumbers(int a, int b) {
return a + b;
}
}
In the provided code example, we have a simple Java program containing a method to add two numbers. However, a parsing error is introduced deliberately by omitting the closing parenthesis in the method call within the main
method.
The main
method attempts to call the addNumbers
method without the closing parenthesis, resulting in a parsing error. This error occurs because the compiler expects to find a closing parenthesis to complete the method call, but it reaches the end of the file instead.
To solve this parsing error, we need to ensure that all opening parentheses in the code have corresponding closing parentheses. In this case, we simply need to add the missing closing parenthesis to the method call within the main
method.
After correcting the error by adding the missing closing parenthesis, the code will compile successfully and produce the following output:
The corrected result is printed to the console, demonstrating the effectiveness of resolving the parsing error caused by missing parentheses. By ensuring proper syntax and structure, we can avoid parsing errors and maintain clean and error-free Java code.
Incomplete Statements
Incomplete statements occur when a line of code is missing essential elements, such as a semicolon or required arguments. These errors can disrupt the flow of code execution and hinder program compilation.
Code Example:
public class IncompleteStatementsExample {
public static void main(String[] args) {
// Incomplete statement
// int result = addNumbers(5, 10) // Error: missing semicolon
// Corrected statement
int correctedResult = addNumbers(5, 10);
System.out.println("Corrected Result: " + correctedResult);
}
// Corrected method with complete statement
private static int addNumbers(int a, int b) {
return a + b;
}
}
In the provided code example, we have a simple Java program containing a method to add two numbers. However, a parsing error is introduced deliberately by omitting the semicolon at the end of the statement within the main
method.
The main
method attempts to assign the result of the addNumbers
method call to a variable, but the statement is incomplete due to the missing semicolon. As a result, the compiler encounters the end of the file while parsing and reports an error.
To solve this parsing error, we need to ensure that all statements in the code are complete, ending with a semicolon. In this case, we simply need to add the missing semicolon to the statement within the main
method.
After correcting the error by adding the missing semicolon, the code will compile successfully and produce the following output:
The corrected result is printed to the console, demonstrating the effectiveness of resolving the parsing error caused by incomplete statements. By ensuring that each statement in the code is complete, we can avoid parsing errors and maintain clean and error-free Java code.
Incomplete Class or Method Declaration
Incomplete class or method declarations occur when essential components, such as curly braces or method bodies, are missing. These errors can disrupt the compilation process and hinder the execution of Java programs.
Code Example:
public class IncompleteDeclarationExample {
public static void main(String[] args) {
// Incomplete method declaration
// int result = addNumbers(5, 10); // Error: missing method body
// Corrected method call
int correctedResult = addNumbers(5, 10);
System.out.println("Corrected Result: " + correctedResult);
}
// Incomplete method declaration
private static int addNumbers(int a, int b); // Error: missing method body
// Corrected method with complete declaration and body
private static int addNumbers(int a, int b) {
return a + b;
}
}
In the provided code example, we have a simple Java program containing a method to add two numbers. However, a parsing error is introduced deliberately by omitting the method body within the addNumbers
method declaration.
The main
method attempts to call the addNumbers
method, but the declaration is incomplete because it lacks a method body. As a result, the compiler encounters the end of the file while parsing and reports an error.
To solve this parsing error, we need to ensure that all class and method declarations in the code are complete, including the required components such as curly braces and method bodies. In this case, we need to provide the missing method body for the addNumbers
method.
After correcting the error by providing the missing method body, the code will compile successfully and produce the following output:
The corrected result is printed to the console, demonstrating the effectiveness of resolving the parsing error caused by incomplete class or method declarations. By ensuring that all class and method declarations are complete, we can avoid parsing errors and maintain clean and error-free Java code.
Missing main
Method
In Java, the main
method serves as the entry point for execution, and its absence can lead to parsing errors during compilation. Understanding how to resolve this error is crucial for ensuring the successful execution of Java programs.
Code Example:
public class MissingMainMethodExample {
// Missing main method
// public static void (String[] args) {
// Correct code
public static void main(String[] args) {
System.out.println("Hello, world!");
}
}
In the provided code example, we have a simple Java class named MissingMainMethodExample
. However, a parsing error is introduced deliberately by omitting the main
method, which serves as the entry point for program execution in Java.
Without the main
method, the compiler encounters the end of the file while parsing and reports an error. This error occurs because the Java Virtual Machine (JVM) requires the main
method to identify where to start executing the program.
To solve this parsing error, we need to ensure that the class contains a main
method with the correct signature. By adding the main
method to the class, we provide the JVM with the necessary entry point for executing the program.
After adding the main
method to the class, the code will compile successfully and produce the following output:
The output demonstrates the successful execution of the program, indicating that the parsing error caused by the missing main
method has been resolved. By ensuring the presence of the main
method, we enable the JVM to execute the Java program without encountering parsing errors.
Missing import
Statements
import
statements are essential for including external classes and packages in Java programs. Failure to include necessary import statements can result in parsing errors during compilation.
Understanding how to resolve this error is vital for ensuring the smooth execution of Java programs.
Code Example:
// missing import will cause parsing error
import java.util.ArrayList;
public class MissingImportExample {
public static void main(String[] args) {
// Attempting to use a class
ArrayList<String> list = new ArrayList<>();
list.add("Hello");
// Displaying the content of the list
System.out.println("List: " + list);
}
}
In the provided code example, we have a simple Java class named MissingImportExample
. However, a parsing error is introduced deliberately by attempting to use the ArrayList
class without importing it.
Without the necessary import
statement (import java.util.ArrayList;
), the compiler is unable to recognize the ArrayList
class, resulting in a parsing error. This error occurs because the compiler expects to find the import
statement to provide access to the ArrayList
class.
By adding the import
statement, we inform the compiler that the ArrayList
class is located in the java.util
package and should be accessible for use in the program.
After adding the import
statement, the code will compile successfully and produce the following output:
The output demonstrates the successful execution of the program, indicating that the parsing error caused by the missing import
statement has been resolved. By ensuring the inclusion of necessary import
statements, we enable the compiler to recognize and utilize external classes and packages effectively in Java programs.
Correct Package Declaration
In Java programming, correct package declarations play a crucial role in organizing and structuring code. A package declaration specifies the package to which a Java file belongs, and errors in this declaration can disrupt the compilation process.
Understanding how to resolve this error is essential for ensuring the successful compilation and execution of Java programs.
Code Example:
// Incorrect package declaration
// package example;
package com.example.error;
public class IncorrectPackageExample {
public static void main(String[] args) {
System.out.println("Hello, world!");
}
}
In the provided code example, we have a simple Java class named IncorrectPackageExample
. However, a parsing error is introduced deliberately by including an incorrect package declaration (package example;
).
The package declaration specifies that the Java file belongs to the example
package. However, if the Java file is not located in the appropriate directory structure corresponding to the package name, it will result in a parsing error.
The incorrect package declaration leads to a parsing error because the file is not in the expected directory structure.
To solve this parsing error, we need to ensure that the package declaration matches the directory structure of the Java file. In this example, we can either remove the package declaration or place the Java file in a directory named example
to match the package declaration.
After resolving the parsing error by correcting the package declaration or adjusting the directory structure, the code will compile successfully and produce the following output:
The output demonstrates the successful execution of the program, indicating that the parsing error caused by the incorrect package declaration has been resolved. By ensuring consistency between package declarations and directory structures, we enable the compiler to correctly identify and parse Java files, resulting in error-free compilation and execution of Java programs.
Conclusion
Understanding and addressing the Reached End of File While Parsing
error in Java is crucial for maintaining clean and error-free code. By adopting best practices, such as correct syntax, brace usage, and proper code organization, developers can ensure a smoother development experience.
This comprehensive approach helps prevent unexpected end-of-file situations during parsing, promoting reliable and efficient Java programming.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack