How to Clear Scanner in Java
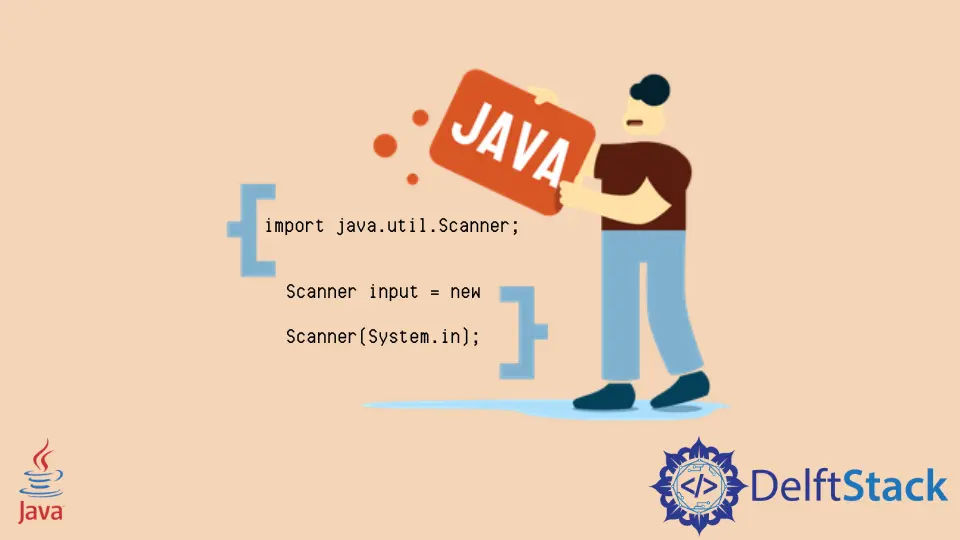
The Scanner
class in Java is often used to take input or output. We create an object of the Scanner
class to use its functions.
We cannot use the close()
method of Scanner
because once a Scanner
is closed by this method, we cannot take input as the input stream has been closed.
There are other ways to clear the Scanner
in Java, and below are the examples that explain these methods.
Java Clear Scanner Using nextLine()
To clear the Scanner
and to use it again without destroying it, we can use the nextLine()
method of the Scanner
class, which scans the current line and then sets the Scanner
to the next line to perform any other operations on the new line.
In the example below, inside the while
loop, we ask the user for an input and check if it is a valid binary using hasNextInt(radix)
. If it is a valid binary, then it will be stored in the binary
variable, and if it is not a binary value, then a message will be printed to ask the user to input a valid binary value.
In this situation, if we don’t clear the Scanner
, the loop will never end. If the value is not binary, then the Scanner
should go to a new line to take a new input. This is is why nextLine()
is used to skip the current line and go to a new line.
import java.util.Scanner;
public class ClearScanner {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int binary = 0;
int radix = 2;
while (binary == 0) {
System.out.print("Please input a valid binary: ");
if (input.hasNextInt(radix)) {
binary = input.nextInt(radix);
} else {
System.out.println("Not a Valid Binary");
}
input.nextLine();
}
System.out.print("Converted Binary: " + binary);
}
}
Output:
Create a New Scanner
Object to Clear Scanner
in Java
Another method to clear the Scanner
is to create a new Scanner
object when the user types a value other than a binary value. This method works because when a new object is created, the existing Scanner
object is cleared, and a new input stream is started.
import java.util.Scanner;
public class ClearScanner {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int binary = 0;
int radix = 2;
while (binary == 0) {
System.out.print("Please input a valid binary: ");
if (input.hasNextInt(radix)) {
binary = input.nextInt(radix);
} else {
System.out.println("Not a Valid Binary");
}
input = new Scanner(System.in);
}
System.out.print("Converted Binary: " + binary);
}
}
Output:
Please input a valid binary: 23
Not a Valid Binary
Please input a valid binary: 11101
Converted Binary: 29
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn