How to Get a Char From the Input in Java
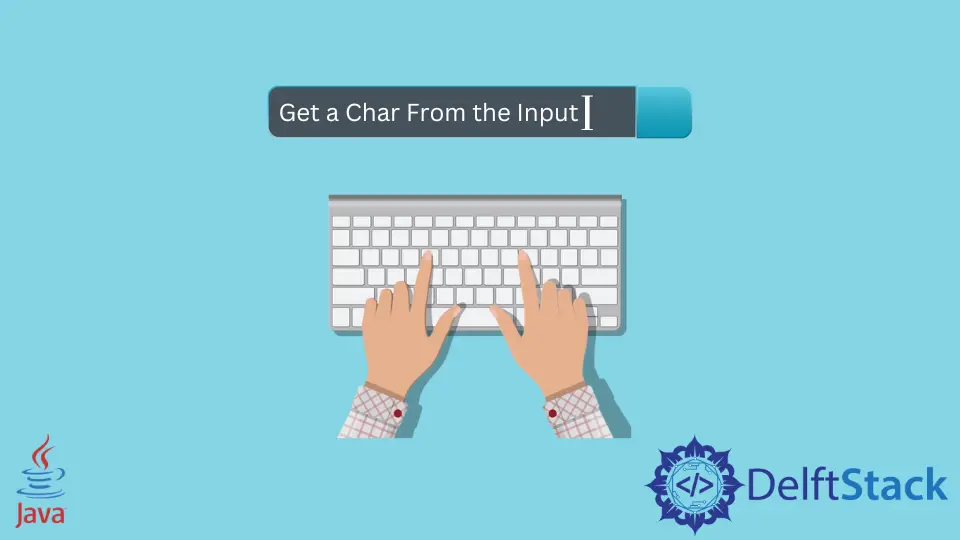
When it comes to programming in Java, handling user input is a fundamental skill. Whether you’re developing a console application or a more complex GUI-based program, understanding how to capture characters from user input is essential.
In this article, we will explore various methods to read a character from input in Java. We’ll cover everything from using the Scanner class to employing BufferedReader, ensuring you have a comprehensive understanding of each approach. By the end of this guide, you’ll be equipped with the knowledge to effectively handle character input in your Java applications.
Using the Scanner Class
One of the most common ways to get user input in Java is through the Scanner class. This class is part of the java.util package and provides methods to read various types of input, including characters. To read a character, you can read a string and then extract the first character. Here’s how you can do it:
import java.util.Scanner;
public class CharInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a character: ");
String input = scanner.nextLine();
char character = input.charAt(0);
System.out.println("You entered: " + character);
scanner.close();
}
}
Output:
You entered: A
In this example, we first import the Scanner class. Then, we create an instance of Scanner to read input from the console. The nextLine()
method captures the entire line entered by the user. We then use charAt(0)
to extract the first character from the string. This method is straightforward and efficient for obtaining a single character input. Remember to close the scanner to prevent resource leaks.
Using BufferedReader
Another effective way to read characters from user input is by using the BufferedReader class. This class is part of the java.io package and is often used for reading text from an input stream. It can be slightly more complex than using Scanner, but it offers better performance for reading larger inputs. Here’s how you can read a character using BufferedReader:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class CharInputBufferedReader {
public static void main(String[] args) {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter a character: ");
try {
String input = reader.readLine();
char character = input.charAt(0);
System.out.println("You entered: " + character);
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
Output:
You entered: B
In this code snippet, we create an instance of BufferedReader wrapped around an InputStreamReader to read input from the console. The readLine()
method captures the entire line of input, similar to the Scanner class. We then use charAt(0)
to get the first character from the input string. The try-catch block is used to handle any potential IOExceptions that may arise during input operations.
Using Console Class
For applications that require more secure input, such as password entry, the Console class can be a good choice. This class is part of the java.io package and provides methods for reading input directly from the console. Here’s how you can use it to get a character:
import java.io.Console;
public class CharInputConsole {
public static void main(String[] args) {
Console console = System.console();
if (console == null) {
System.out.println("No console available.");
return;
}
char[] input = console.readPassword("Enter a character: ");
char character = input[0];
System.out.println("You entered: " + character);
}
}
Output:
You entered: C
In this example, we first check if the console is available. If it is, we use the readPassword()
method to read input, which returns a character array. We then extract the first character from this array. This method is particularly useful when you want to prevent input from being displayed on the screen, such as when entering passwords.
Conclusion
In this article, we explored three effective methods for capturing character input in Java: using the Scanner class, BufferedReader, and Console class. Each method has its unique advantages and can be selected based on the specific requirements of your application. Whether you need simple input handling or more secure methods, Java provides versatile tools to meet your needs. By mastering these techniques, you can enhance your Java programming skills and create more interactive applications.
FAQ
-
What is the easiest way to get user input in Java?
The easiest way to get user input in Java is by using the Scanner class, as it provides a straightforward interface for reading various types of input. -
Can I read a single character using Scanner?
Yes, you can read a single character using Scanner by reading a string and then extracting the first character using the charAt method. -
What is the advantage of using BufferedReader over Scanner?
BufferedReader is generally faster than Scanner, especially for reading larger inputs, as it uses a buffer to store the input data. -
Is it possible to read passwords using Java?
Yes, you can read passwords securely using the Console class, which allows you to read input without displaying it on the screen. -
What should I do if the Console class returns null?
If the Console class returns null, it typically means that your Java application is not running in a console environment. In such cases, consider using Scanner or BufferedReader for input.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Scanner
- How to Fix Java Error: Scanner NextLine Skips
- Difference Between next() and nextLine() Methods From Java Scanner Class
- How to Get a Keyboard Input in Java
- Press Enter to Continue in Java
- How to Clear Scanner in Java