Press Enter to Continue in Java
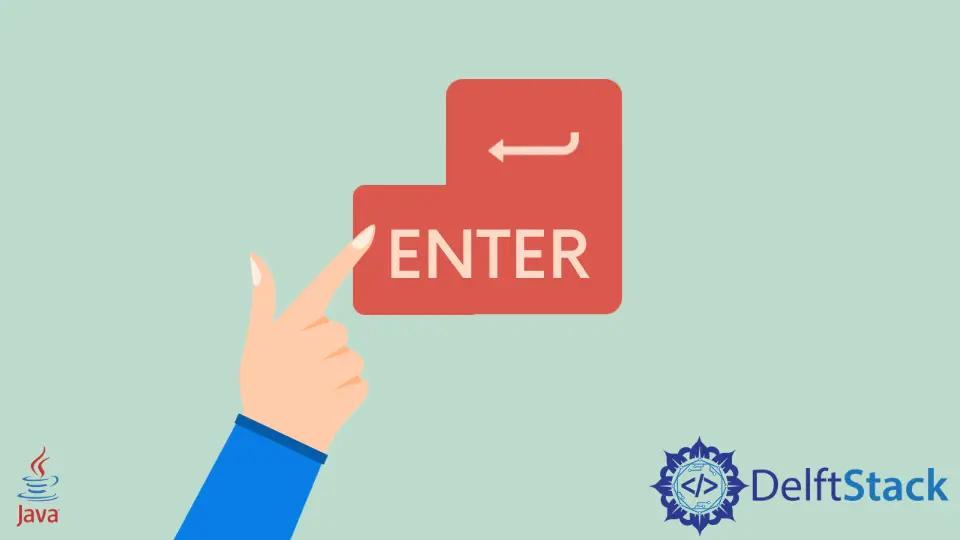
We have introduced how to get input from console in Java in another article. This article will show you how we put the console on hold until the user presses Enter, then the next message will be displayed. In the following example, we will use the Scanner
class to take three inputs and then show them on the console.
Using an Extra nextLine()
to Wait for Enter Key in Java
In the example, we create an object of the Scanner
class, sc
. Scanner
scans the text and parse primitive types like int
and String
. This class comes with a lot of methods that are used in input operations. The most commonly used methods are the nextInt()
, nextLine
, nextDouble
, and etc.
In the below example, we will see where the issue lies, and we’ll use a technique to solve it on the next one.
Here, we take three inputs: the int
type, and the next two are of the String
types. We use the Scanner
class to first call the nextInt()
method to get the input for age into an int
type variable; then, to take input for the first name and last name, we use two sc.nextLine()
methods and two variables to store them. At last, we show all the values of variables in the output.
The issue lies when the user inputs the value for age
and presses Enter. The console is supposed to get the input for firstName
, but it skips it and directly goes for the lastName
. The output below shows the issue clearly.
import java.util.Scanner;
public class EnterContinue {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter your age: ");
int age = sc.nextInt();
System.out.print("Enter your First Name: ");
String firstName = sc.nextLine();
System.out.print("Enter your last Name: ");
String lastName = sc.nextLine();
System.out.println("Your Info:\nAge: " + age + "\nFull Name: " + firstName + " " + lastName);
}
}
Output:
Enter your age: 23
Enter your First Name: Enter your last Name: John
Your Info:
Age: 23
Full Name: John
Process finished with exit code 0
Now that we know the issue, we can discuss the solution and why the problem occurs in the first place. When we take an int
value as an input, the method nextInt()
reads the int
value and cannot read the newline. This is why when we press Enter after reading int
, the console sees it as a newline and skips it to the following statement.
We have to use an extra nextLine()
method after the nextInt()
function that will skip the newline and shows the next line to tackle this issue. In the output, we can see that now we will enter the first name, which wasn’t possible in the previous example.
import java.util.Scanner;
public class EnterContinue {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter your age: ");
int age = sc.nextInt();
System.out.print("Enter your First Name: ");
String firstName = sc.nextLine();
sc.nextLine();
System.out.print("Enter your last Name: ");
String lastName = sc.nextLine();
System.out.println("Your Info:\nAge: " + age + "\nFull Name: " + firstName + " " + lastName);
}
}
Output:
Enter your age: 5
Enter your First Name: gfd
Enter your last Name: gfgf
Your Info:
Age: 5
Full Name: gfgf
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn