How to Convert Object to String in Java
-
Convert Object to String Using the
valueOf()
Method in Java -
Convert Object to String Using the
+
Operator in Java -
Convert Object to String Using the
toString()
Method in Java -
Convert Object to String Using the
toString()
Method in Java -
Convert Object to String Using
toString()
Method in Java -
Convert Object to String Using the
join()
Method in Java
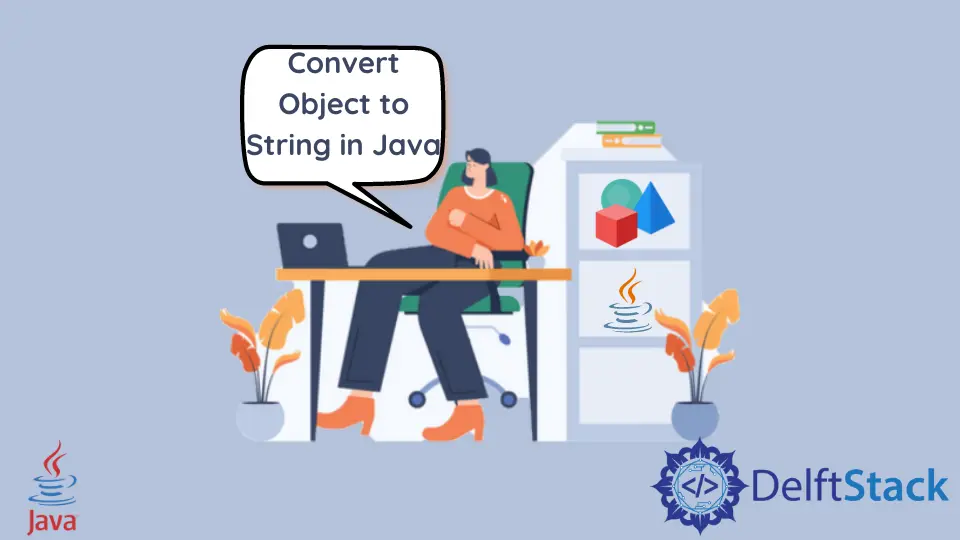
This tutorial introduces how to convert an object to a string in Java.
Convert Object to String Using the valueOf()
Method in Java
The valueOf()
method of the String
class can convert an object to a string. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
Object obj = "DelftStack Portal";
System.out.println("Object value: " + obj);
String str = String.valueOf(obj);
System.out.println("String value: " + str);
}
}
Output:
Object value: DelftStack Portal
String value: DelftStack Portal
Convert Object to String Using the +
Operator in Java
In Java, the plus operator +
concatenates any type value with the string and returns a resultant string. We can use it to convert an object to a string too. See the below example.
public class SimpleTesting {
public static void main(String[] args) {
Object obj = "DelftStack Portal";
System.out.println("Object value: " + obj);
String str = "" + obj;
System.out.println("String value: " + str);
}
}
Output:
Object value: DelftStack Portal
String value: DelftStack Portal
Convert Object to String Using the toString()
Method in Java
The toString()
method of the Object
class converts any object to the string. See the below example.
public class SimpleTesting {
public static void main(String[] args) {
Object obj = "DelftStack Portal";
System.out.println("Object value: " + obj);
String str = obj.toString();
System.out.println("String value: " + str);
}
}
Output:
Object value: DelftStack Portal
String value: DelftStack Portal
Convert Object to String Using the toString()
Method in Java
An object can be of any type. For example, if we have an integer object and want to get its string object, use the toString()
method. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
Integer iVal = 123;
System.out.println("Integer Object value: " + iVal);
String str = iVal.toString();
System.out.println("String value: " + str);
}
}
Output:
Hello
This
is
DelfStack
Convert Object to String Using toString()
Method in Java
This example explains how to convert a user-defined object to a string using the toString()
method. See the example below.
class Employee {
String fName;
String lName;
public Employee(String fName, String lName) {
this.fName = fName;
this.lName = lName;
}
public String getfName() {
return fName;
}
public void setfName(String fName) {
this.fName = fName;
}
public String getlName() {
return lName;
}
public void setlName(String lName) {
this.lName = lName;
}
@Override
public String toString() {
return "Employee [fName=" + fName + ", lName=" + lName + "]";
}
public String getString() {
return toString();
}
}
public class SimpleTesting {
public static void main(String[] args) {
Employee employee = new Employee("Rohan", "Mosac");
System.out.println(employee.getString());
}
}
Output:
Employee [fName=Rohan, lName=Mosac]
Convert Object to String Using the join()
Method in Java
Here, we convert an ArrayList
object to a string by using the join()
method. The join()
method of the String
class returns a string after joining them into a single String
object. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("Sun");
list.add("Moon");
list.add("Earth");
System.out.println("List object: " + list);
// list object to string
String str = String.join(",", list);
System.out.println("String: " + str);
}
}
Output:
List object: [Sun, Moon, Earth]
String: Sun,Moon,Earth
Related Article - Java Object
- How to Parse XML to Java Object
- How to Serialize Object to JSON in Java
- How to Serialize Object to String in Java
- How to Implement Data Access Object in Java
- How to Sort an Array of Objects in Java
- How to Print Objects in Java